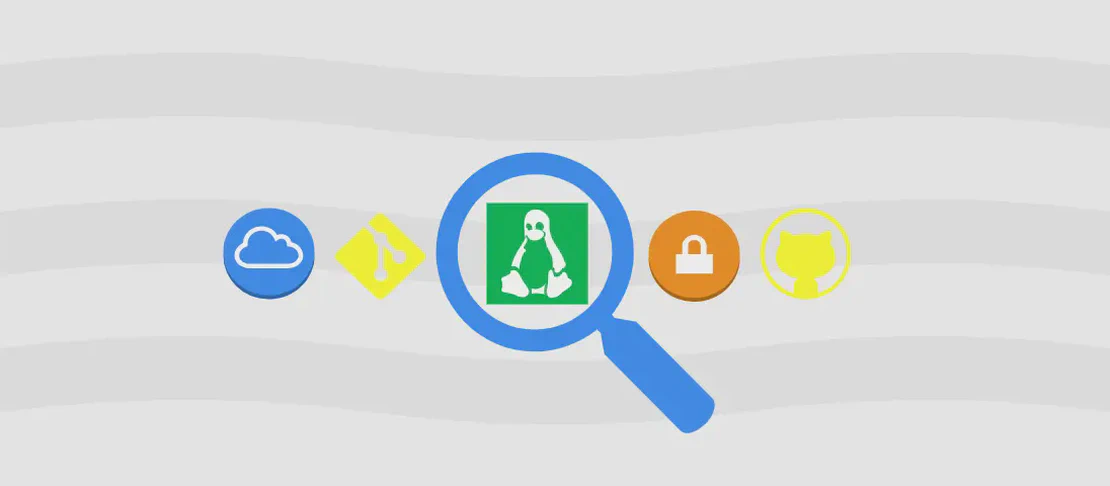
Understanding PHP Mess Detector (phpmd) with Examples
PHP Mess Detector (phpmd) is a powerful command-line tool designed for PHP developers. It helps in identifying potential problems and improving the quality of the codebase by scanning PHP files or directories. This tool makes it easier to enforce coding standards and practices, promoting maintainable and clean code. The tool works by applying a series of pre-defined or custom rulesets to the code it analyzes. In this article, we will explore various use cases of the phpmd command, providing motivation, detailed explanations, and sample outputs for each.
Display a List of Available Rulesets and Formats
Code:
phpmd
Motivation:
The ability to view a list of available rulesets and formats is crucial for users who are new to PHP Mess Detector or those who need a reference for possible options. It allows developers to understand which rulesets can be applied and in what formats results can be obtained, aiding in customizing the tool to fit specific project needs.
Explanation:
phpmd
: This is the base command for PHP Mess Detector. When run without additional arguments, it displays the available rulesets and output formats that can be used for analyzing code.
Example Output:
Available formats: xml, text, html
Available rulesets: cleancode, codesize, controversial, design, naming, unusedcode
Scan a File or Directory for Problems Using Comma-Separated Rulesets
Code:
phpmd path/to/file_or_directory xml|text|html ruleset1,ruleset2,...
Motivation:
Scanning a file or directory for potential code issues using specific rulesets is one of the primary functions of phpmd. This use case highlights the capability of the tool to apply multiple rulesets during analysis, thus ensuring comprehensive code checks according to different guidelines or project requirements.
Explanation:
path/to/file_or_directory
: Specifies the path to the file or directory to be analyzed. This path can be absolute or relative.xml|text|html
: Determines the format in which the report output should be generated. The choice depends on the user’s preference or project documentation requirements.ruleset1,ruleset2,...
: A comma-separated list of rulesets to be applied during the analysis. Rulesets determine the types of problems that phpmd checks for within the code.
Example Output:
/path/to/file.php
line 8 message Potential problem detected...
line 15 message Code size exceeds threshold...
Specify the Minimum Priority Threshold for Rules
Code:
phpmd path/to/file_or_directory xml|text|html ruleset1,ruleset2,... --minimumpriority priority
Motivation:
In some projects, it may be necessary to focus on higher-priority issues first. By specifying a minimum priority threshold, developers can filter out lower-priority issues and concentrate on resolving critical problems, optimizing resource allocation and time management.
Explanation:
--minimumpriority priority
: Sets the minimum priority level of rules to be reported. Priority levels range from 1 (most severe) to 5 (least severe). Only issues with a level equal or lower to the specified threshold will be included in the report.
Example Output:
/path/to/file.php
line 10 message Critical issue found...
Include Only the Specified Extensions in Analysis
Code:
phpmd path/to/file_or_directory xml|text|html ruleset1,ruleset2,... --suffixes extensions
Motivation:
Projects may contain files with various extensions, and not all may be relevant for PHP analysis. Limiting analysis to specific file extensions ensures that phpmd focuses only on relevant files, improving performance and generating more meaningful results.
Explanation:
--suffixes extensions
: Limits the analysis to files with the specified extensions. This flag accepts a comma-separated list of file suffixes (e.g.,php,inc
) and ignores files with other extensions.
Example Output:
Considering only files with extensions: php
Analyzing file.php...
Exclude the Specified Comma-Separated Directories
Code:
phpmd path/to/file_or_directory1,path/to/file_or_directory2,... xml|text|html ruleset1,ruleset2,... --exclude directory_patterns
Motivation:
In complex projects, certain directories might contain generated code or vendor libraries that should be excluded from analysis. This feature allows developers to focus phpmd on the core application code, avoiding unnecessary issues from these excluded paths.
Explanation:
--exclude directory_patterns
: Directories that match the provided patterns will be excluded from analysis. Multiple patterns can be specified, separated by commas, allowing for fine-grained control over which parts of a codebase should be analyzed.
Example Output:
Excluding directories: /vendor,/cache
Analyzing remaining codebase...
Output the Results to a File Instead of stdout
Code:
phpmd path/to/file_or_directory xml|text|html ruleset1,ruleset2,... --reportfile path/to/report_file
Motivation:
For continuous integration processes or documentation purposes, it is often necessary to store analysis results in a file. This functionality supports automated workflows by recording phpmd outputs in a specified location for further review or integration with other tools.
Explanation:
--reportfile path/to/report_file
: Directs phpmd to write its report to a specified file instead of displaying it on standard output. This can be useful for logging purposes or when integrating phpmd with CI/CD pipelines.
Example Output:
Results saved to: path/to/report_file
Ignore the Use of Warning-Suppressive PHPDoc Comments
Code:
phpmd path/to/file_or_directory xml|text|html ruleset1,ruleset2,... --strict
Motivation:
Certain projects may rely heavily on PHPDoc comments to suppress warnings. Using the strict option allows phpmd to ignore such suppressions, potentially revealing a more comprehensive set of issues and facilitating improvements in code quality where implicit assumptions were previously made.
Explanation:
--strict
: This option instructs phpmd to disregard any PHPDoc comments intended to suppress warnings, thus ensuring that all potential issues are reported regardless of these annotations.
Example Output:
Ignoring suppression comments...
/path/to/file.php
line 20 message Suppressed warning reported...
Conclusion:
PHP Mess Detector (phpmd) is a versatile tool that assists developers in maintaining high standards of code quality by identifying potential issues within PHP projects. Through the diverse use cases explored in this article, the flexible and customizable nature of phpmd is evident, meeting the needs of various stakeholders in a development cycle. Whether it’s focusing on specific files, integrating with automated processes, or excluding irrelevant directories, phpmd provides the functionality necessary for efficient and thorough code analysis.