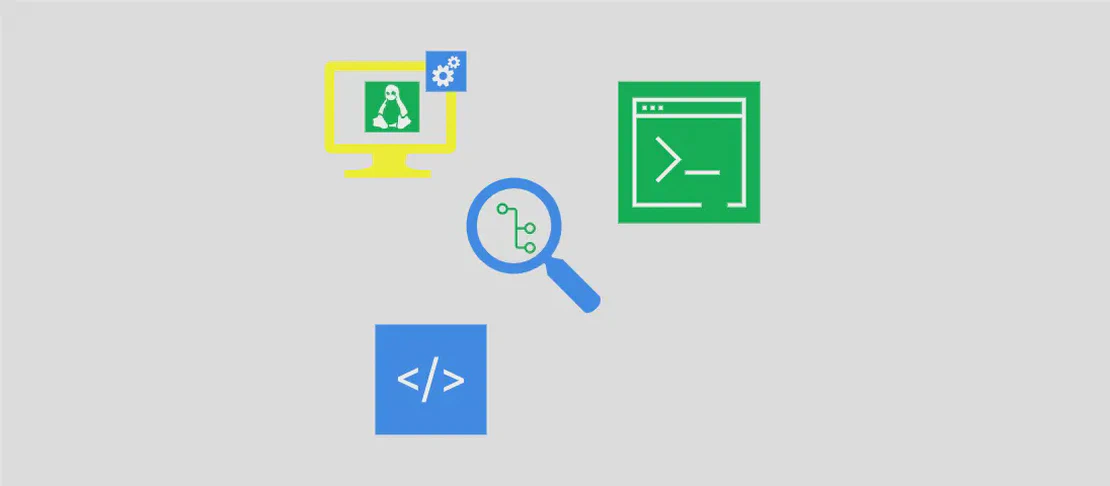
Mastering the 'phpspec' Command for PHP Development (with examples)
Phpspec is a powerful behavior-driven development (BDD) tool for PHP that facilitates writing clean and tested code by focusing on how the software should behave. By writing specifications or “specs” in PHP, phpspec encourages developers to describe the expected behavior of their classes and methods. It helps guide the design of clean, maintainable code by supporting test-driven development practices.
Use case 1: Create a Specification for a Class
Code:
phpspec describe class_name
Motivation:
When starting to build a new PHP component, it is crucial to have a clear specification of what the component is supposed to achieve. Using phpspec to create a specification for a class helps in defining this behavior upfront, leading to a clearer design. This approach allows a developer to explore the requirements and expected behaviors of a specific class before writing the actual implementation, adhering to the test-first philosophy of behavior-driven development.
Explanation:
describe
: This is a phpspec command that initiates the creation of a specification file for the indicated class.class_name
: This represents the name of the class for which you want to create a specification. Phpspec will use this name to generate a spec file in the appropriate directory.
Example Output:
Executing this command might result in the creation of a file such as spec/ClassNameSpec.php
, populated with a template specification that can be expanded with behavioral examples and expectations.
Use case 2: Run All Specifications in the “spec” Directory
Code:
phpspec run
Motivation:
Running all specifications is a standard action during the development process to ensure that the entire codebase adheres to its expected behavior. It provides a comprehensive verification, ensuring all components work as intended. This is particularly useful for regression testing after any modification, as it quickly highlights any unintended breakages in the existing behavior.
Explanation:
run
: This command tells phpspec to execute all specs located within the default specification directory, typicallyspec
. It compiles the results of these tests to confirm conformances or discrepancies with the described behavior.
Example Output:
After execution, phpspec will output a summary that indicates which specs passed or failed, including detailed information on any mismatches found between expected and actual behavior.
Use case 3: Run a Single Specification
Code:
phpspec run path/to/class_specification_file
Motivation:
Running a single specification file is beneficial during isolated development of a component or when troubleshooting a specific behavior without running the full suite of tests. This focused approach is more efficient, saving time and resources during development, especially when refining or debugging a specific part of the codebase.
Explanation:
run
: As previously described, this command runs the spec tests.path/to/class_specification_file
: The path points directly to the specific spec file you wish to execute. It allows you to narrow down the test execution to just this one file, providing targeted feedback.
Example Output:
The output would include a test report for just this one specification file, highlighting any deviations from the expected output or confirming success.
Use case 4: Run Specifications Using a Specific Configuration File
Code:
phpspec run -c path/to/configuration_file
Motivation:
Sometimes projects require different configurations for their tests, particularly in environments with multiple dependencies or particular settings needed for certain tests. By specifying a configuration file, developers can customize the testing environment, thus maintaining the flexibility of test execution across varying contexts such as local development, staging, or production environments.
Explanation:
run
: Initiates the execution of tests.-c
: This parameter specifies that a custom configuration file is to be used for this run.path/to/configuration_file
: This is the path to the configuration file that contains specific settings phpspec should use.
Example Output:
Using a custom configuration file may result in phpspec executing tests with different environment variables, settings, or extensions loaded, which could alter test results depending on configurations.
Use case 5: Run Specifications Using a Specific Bootstrap File
Code:
phpspec run -b path/to/bootstrap_file
Motivation:
Bootstrap files in PHP initialization scripts reduce manual configuration efforts across test and execution environments. Using a bootstrap file when running phpspec tests ensures that essential setups, such as loading necessary frameworks or setting global configurations, are consistently applied, thus creating a constant environment for running specifications.
Explanation:
run
: Commands execution of all specific specs.-b
: Denotes the use of a bootstrap file.path/to/bootstrap_file
: The file path specifies the script to be executed before running any specifications, initializing the environment.
Example Output:
Tests would be executed with environments possibly pre-seeded with data, initialized connections, or other pre-run configurations as specified in the bootstrap file.
Use case 6: Disable Code Generation Prompts
Code:
phpspec run --no-code-generation
Motivation:
While phpspec provides interactive prompts to assist with code generation based on failing tests, there are times when a developer might prefer to manually manage code updates. Disabling these prompts avoids interruptions during test runs, allowing for quicker iteration, particularly when automatic code changes might disrupt an intended manual refactor.
Explanation:
run
: Executes the given specs command.--no-code-generation
: This option turns off automatic prompts for creating or updating class methods according to specifications, giving full control to the developer.
Example Output:
Running this command results in a simpler output with straightforward pass/fail messages without any suggestions or prompts for automatic changes in code.
Use case 7: Enable Fake Return Values
Code:
phpspec run --fake
Motivation:
Enabling fake return values allows developers to concentrate on defining behaviors without needing all dependencies to be fully implemented. This is particularly useful during the early stages of development or when working with external dependencies that are yet to be integrated, as it speeds up the specification writing by avoiding real data dependencies.
Explanation:
run
: Initiates the test process.--fake
: Activates phpspec’s ability to return dummy data for method calls that are not yet implemented, aiding quick spec writing.
Example Output:
The command would execute with phpspec returning “fake” or default values, providing immediate feedback on specs that might otherwise fail due to missing implementations.
Conclusion:
The phpspec command and its various options empower PHP developers to employ BDD practices efficiently. With the ability to create specifications, execute them with different configurations, and manage testing environments, developers can craft well-defined and tested PHP codebases, enhancing both code quality and maintainability. By using phpspec’s capabilities to their fullest, teams can ensure their software behaves as intended right from the conceptualization and design stages.