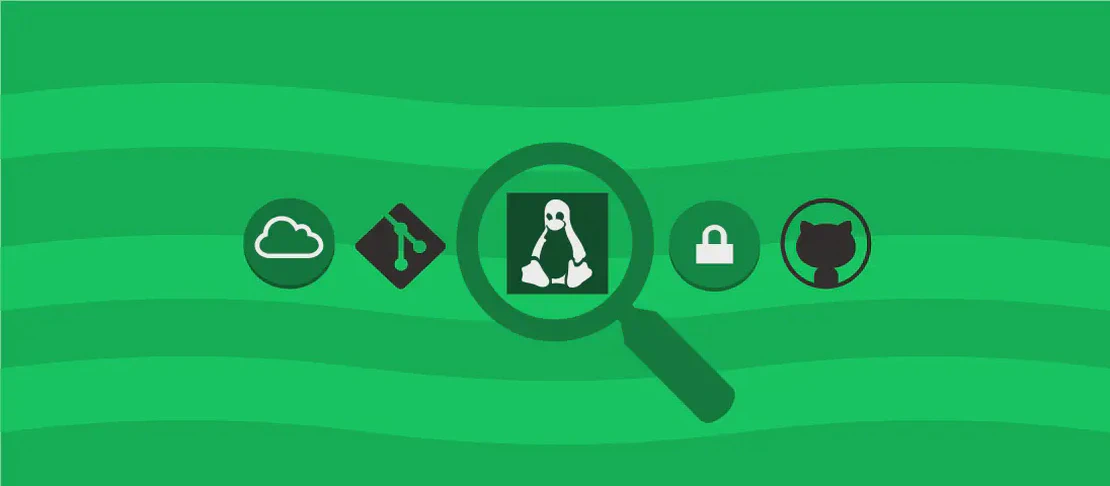
An Introduction to PHPStan (with examples)
PHPStan is a powerful static analysis tool designed for PHP developers. By examining your code without executing it, PHPStan identifies potential bugs and logical errors, ensuring higher quality code and reducing runtime issues. Unlike traditional debugging methods, PHPStan allows you to catch problems early in the development process, offering significantly improved code robustness. This article explores several use cases of PHPStan, illustrating how developers can leverage this tool for better PHP code analysis.
Analyze one or more directories
Code:
phpstan analyse path/to/directory1 path/to/directory2 ...
Motivation: You may be working on a large PHP project spread across multiple directories. Analyzing these directories simultaneously with PHPStan allows you to maintain consistency and ensure that potential errors are caught early across the entire codebase. This comprehensive scan is crucial for maintaining code quality in projects with multiple interdependent components.
Explanation:
analyse
: This command initiates the analysis process in PHPStan.path/to/directory1 path/to/directory2 ...
: Specifies the directories you want PHPStan to analyze. You can include as many as needed, separated by spaces, allowing you to target all relevant parts of your project at once.
Example Output:
123/456 [▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓ ] 27%
Detected issues in path/to/directory1/File1.php:
Return type of method someMethod() should be void, but returns string.
Detected issues in path/to/directory2/File2.php:
Expected type 'int', found 'string' instead.
Analyze a directory using a configuration file
Code:
phpstan analyse path/to/directory --configuration path/to/config
Motivation: Large projects often have specific analysis requirements or configurations unique to their architecture or coding standards. Utilizing a configuration file allows you to define these specifics, such as custom rule sets, exclusion patterns, and directory-specific settings, ensuring PHPStan tailors its analysis according to your project’s unique needs.
Explanation:
analyse
: Initiates the analysis process.path/to/directory
: Indicates which directory PHPStan should analyze.--configuration path/to/config
: Points PHPStan to a configuration file where you can specify custom rules, directories to ignore, and other project-specific settings.
Example Output:
114/200 [▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓ ] 57%
Detected configuration errors:
Line 34: The type 'array<string>' does not match project standards.
Analyze using a specific rule level (0-7, higher is stricter)
Code:
phpstan analyse path/to/directory --level level
Motivation: Different projects require varying levels of strictness in code analysis, depending on their stage of development or coding guidelines. PHPStan’s level system (ranging from 0 to 7) provides the flexibility to adjust the strictness of the analysis. By choosing a higher level, developers can enforce more stringent code checks, fostering better code quality and discipline.
Explanation:
analyse
: Starts the analysis.path/to/directory
: Directory to be analyzed.--level level
: Number between 0 and 7 defining the strictness of analysis, where higher numbers enforce stricter checks.
Example Output:
Analyzing with strictness level 5...
40/40 [▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓ ] 100%
No issues found at level 5.
Specify an autoload file to load before analyzing
Code:
phpstan analyse path/to/directory --autoload-file path/to/autoload_file
Motivation: PHP projects often use autoloaders to include classes and dependencies at runtime. For more accurate analysis, PHPStan should understand your project’s loading mechanisms. Specifying an autoload file ensures PHPStan can accurately interpret your code’s reference classes, interfaces, and traits as they would appear during runtime.
Explanation:
analyse
: Initiates PHPStan’s analysis.path/to/directory
: Directs which directory should be analyzed.--autoload-file path/to/autoload_file
: Instructs PHPStan to load a specified autoload file, allowing it to resolve class dependencies correctly.
Example Output:
77/77 [▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓▓ ] 100%
Warning: Class 'DependencyClass' not found, ensure the autoload file is complete.
Specify a memory limit during analysis
Code:
phpstan analyse path/to/directory --memory-limit memory_limit
Motivation: Large codebases or limited system resources may require controlling the memory allocated to PHPStan during analysis. Setting a memory limit can prevent your system from becoming overburdened, reducing the risk of slowdowns or crashes during the analysis process, especially for resource-intensive environments.
Explanation:
analyse
: Starts analyzing your PHP code.path/to/directory
: The target directory for analysis.--memory-limit memory_limit
: Specifies the maximum memory PHPStan can use. This can be set in shorthand (e.g., 512M for 512 megabytes or 1G for 1 gigabyte).
Example Output:
Starting analysis with memory limit of 512M...
Memory limit exceeded, consider increasing the limit for optimal performance.
Display available options for analysis
Code:
phpstan analyse --help
Motivation:
Understanding the full range of PHPStan’s capabilities is crucial for developers looking to leverage all its features. The --help
option provides a comprehensive list of commands and options, serving as a quick reference to learn about various functionalities or refresh existing knowledge.
Explanation:
analyse
: This basic command initializes the process but here is used for showing options.--help
: Displays comprehensive help information, including all available commands, options, and potential usages.
Example Output:
Usage:
command [options] [arguments]
Options:
--level Define analysis strictness level (0-7)
--configuration Specify a configuration file
--autoload-file Path to autoload file
--memory-limit Define maximum memory usage
...
Conclusion
PHPStan serves as an invaluable tool for PHP developers aiming to ensure code integrity and reduce the risk of runtime errors. By offering a variety of customizable options such as directory analysis, configuration settings, rule strictness, and memory management, PHPStan empowers developers to conform to best coding practices tailored to their project needs. Whether you are analyzing small projects or large multi-directory applications, PHPStan provides the flexibility and rigor needed for superior code quality assurance.