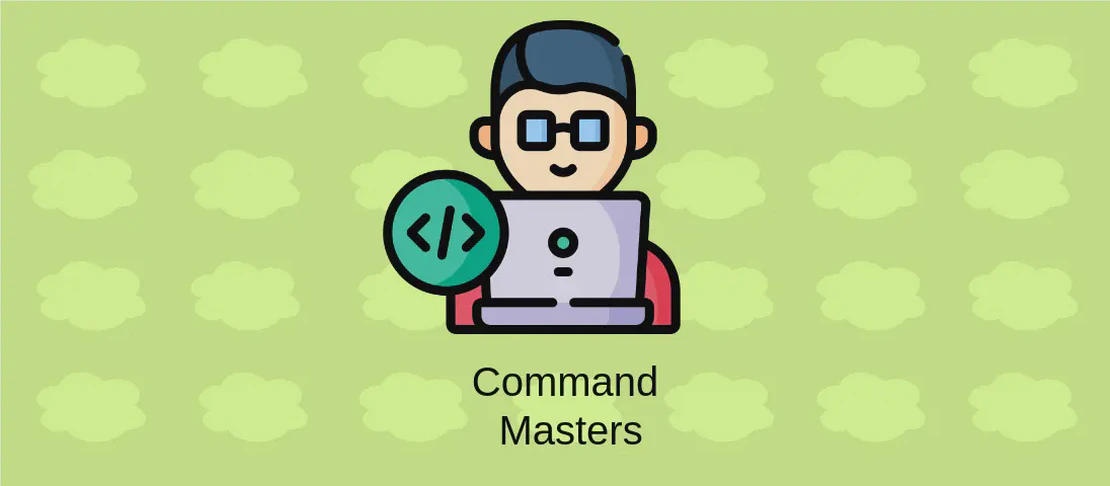
Harnessing the Power of PHPUnit: A Guide to Use Cases (with examples)
PHPUnit is a widely-used framework in the PHP development ecosystem designed for unit testing. It allows developers to write and run tests, ensuring that code behaves as expected. The framework’s command-line tool, phpunit
, provides a variety of functionalities that streamline the testing process. These features include executing all tests in a directory, targeting specific test files, running grouped tests, and generating detailed code coverage reports. This article delves into these use cases, highlighting the practical application of PHPUnit’s command-line functionalities.
Use case 1: Run tests in the current directory
Code:
phpunit
Motivation for using this example:
Running all tests within the current directory is one of the most straightforward and commonly used features of PHPUnit. It allows developers to quickly execute their entire test suite, ensuring that all parts of their application are functioning correctly before proceeding to deploy or further develop the software. This holistic approach to testing helps in identifying any unexpected breakdowns between different parts of the code.
Explanation:
This command does not require any additional arguments, assuming that the user has a phpunit.xml
configuration file in the current directory. The phpunit.xml
file dictates the test suite’s setup and provides PHPUnit with information about test file locations and any specific configurations needed for those tests.
Example output:
PHPUnit 9.0 by Sebastian Bergmann and contributors.
...................
Time: 1.21 seconds, Memory: 12.00 MB
OK (17 tests, 34 assertions)
Use case 2: Run tests in a specific file
Code:
phpunit path/to/TestFile.php
Motivation for using this example:
Sometimes, a developer may only need to verify the tests within a specific file, especially when focusing on a particular module or segment of their application. This targeted approach saves time by bypassing other tests, allowing for a focused check on recently modified or problematic code sections.
Explanation:
path/to/TestFile.php
specifies the path to the particular test file that needs to be executed. By providing this path, PHPUnit runs only the tests contained within that file, rather than the entire suite.
Example output:
PHPUnit 9.0 by Sebastian Bergmann and contributors.
...
Time: 0.13 seconds, Memory: 6.00 MB
OK (3 tests, 6 assertions)
Use case 3: Run tests annotated with the given group
Code:
phpunit --group name
Motivation for using this example:
In complex projects, tests can be organized into groups based on functionality or other categorizations. Running tests by group is beneficial when different teams are responsible for separate aspects of a project, or when a developer wants to focus on specific areas, such as performance or regression tests, without executing unrelated tests.
Explanation:
--group name
: This flag tells PHPUnit to only execute tests annotated with the specified group name. The group name is defined within the test files using@group name
annotations.
Example output:
PHPUnit 9.0 by Sebastian Bergmann and contributors.
.....
Time: 0.50 seconds, Memory: 8.00 MB
OK (5 tests, 10 assertions)
Use case 4: Run tests and generate a coverage report in HTML
Code:
phpunit --coverage-html path/to/directory
Motivation for using this example:
Generating a code coverage report is an essential step for ensuring all parts of the code are tested adequately. HTML reports make this information accessible and visually interpretable, providing insights into untested or under-tested parts of the codebase. This serves as a valuable tool for improving test quality and code reliability.
Explanation:
--coverage-html path/to/directory
: This flag generates an HTML coverage report. Thepath/to/directory
argument specifies where the report will be saved. This report visually displays which lines of code were executed during the tests and which were not, helping developers to identify untested code.
Example output:
PHPUnit 9.0 by Sebastian Bergmann and contributors.
Generating code coverage report in HTML format ... done
Time: 2.40 seconds, Memory: 18.00 MB
OK (20 tests, 40 assertions)
Conclusion:
PHPUnit’s versatility through its command-line capabilities facilitates effective test execution tailored to specific needs, be it running a complete suite, validating changes in a single file, focusing on grouped functionalities, or analyzing code coverage. By utilizing these options, developers enhance their ability to maintain high-quality, reliable software, ultimately leading to better application performance and user satisfaction.