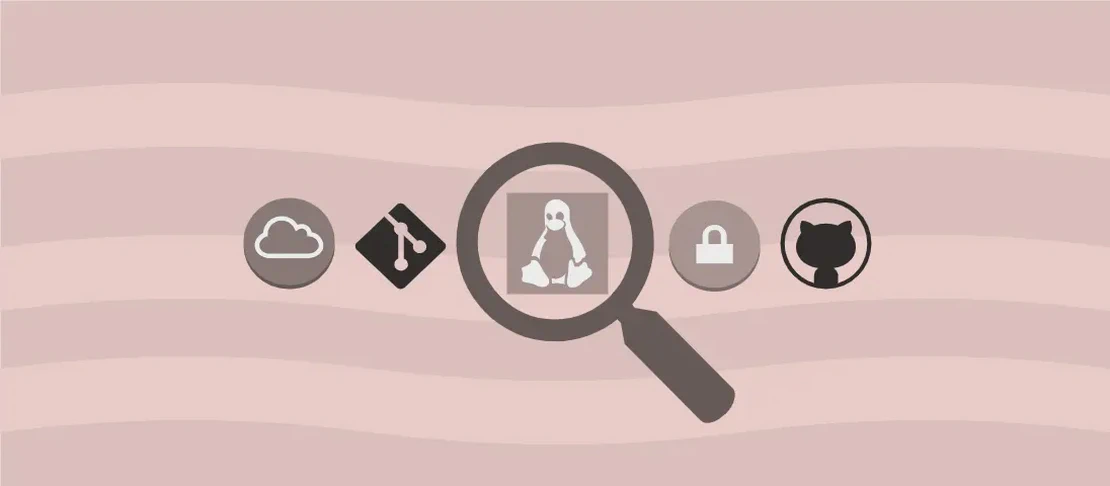
How to Use the Python Package Manager 'pip' (with examples)
The Python Package Manager, commonly referred to as pip
, is an essential tool for Python developers. It allows users to install and manage third-party Python packages with ease. This command-line utility streamlines the process of incorporating libraries into projects, ensuring efficient dependency handling and easy integration. Whether you’re looking to install new packages, upgrade current ones, or manage your project’s requirements, pip
provides the necessary functionality with just a few simple commands. Below, we illustrate the versatility of pip
through several practical use cases.
Install a Package
Code:
pip install package
Motivation:
Installing a package using pip
is a fundamental operation for any Python developer. By leveraging the extensive repository of Python packages available in the Python Package Index (PyPI), developers can easily enhance their applications with additional functionality without reinventing the wheel.
Explanation:
pip install
: Theinstall
subcommand tellspip
that you want to add a new package to your environment.package
: This placeholder represents the name of the package you wish to install. Replace it with the actual package name.
Example Output:
Collecting package
Downloading package-x.x.x-py2.py3-none-any.whl (size)
Installing collected packages: package
Successfully installed package-x.x.x
Install a Package to the User’s Directory
Code:
pip install --user package
Motivation:
There are scenarios where you may not have administrative privileges to install packages system-wide, such as on a shared server. Installing to the user’s directory circumvents this issue, allowing you to add packages to your local environment without affecting others or needing special permissions.
Explanation:
--user
: This option directspip
to install the package to a directory specific to the user rather than system-wide.package
: The package name you intend to install.
Example Output:
Collecting package
Downloading package-x.x.x-py2.py3-none-any.whl (size)
Installing collected packages: package
WARNING: The script package is installed in '/home/user/.local/bin' which is not on PATH.
Successfully installed package-x.x.x
Upgrade a Package
Code:
pip install --upgrade package
Motivation:
Keeping dependencies up to date is crucial for security and performance. Upgrading packages ensures that your project benefits from the latest bug fixes, features, and security patches, reducing vulnerabilities and improving stability.
Explanation:
--upgrade
: This flag signals pip to upgrade the specified package to the latest available version.package
: The package name you wish to upgrade.
Example Output:
Collecting package
Downloading package-x.x.x-py2.py3-none-any.whl (size)
Installing collected packages: package
Attempting uninstall: package
Found existing installation: package x.x.x
Uninstalling package-x.x.x:
Successfully uninstalled package-x.x.x
Successfully installed package-x.x.x
Uninstall a Package
Code:
pip uninstall package
Motivation:
Streamlining your development environment by removing unnecessary or obsolete packages can avoid conflicts and reduce storage usage. Uninstalling a package that is no longer needed keeps your environment lean and manageable.
Explanation:
uninstall
: This command informspip
that you want to remove the specified package.package
: The package name you want to uninstall.
Example Output:
Found existing installation: package x.x.x
Uninstalling package-x.x.x:
Would remove:
/path/to/package
Proceed (Y/n)? y
Successfully uninstalled package-x.x.x
Save Installed Packages to File
Code:
pip freeze > requirements.txt
Motivation:
Capturing the current state of your project’s dependencies makes sharing and replication practical and efficient. This command is invaluable when setting up a new development environment or deploying an application, ensuring consistency across different setups.
Explanation:
freeze
: This command outputs the installed packages within your current environment.> requirements.txt
: This shell redirection symbol writes the output into a file namedrequirements.txt
.
Example Output:
pippackage-x.x.x
anotherpackage-y.y.y
yetanotherpackage-z.z.z
Show Installed Package Info
Code:
pip show package
Motivation:
Having detailed information about installed packages aids in dependency management. Knowing the version, author, home page, and other metadata helps manage upgrades, resolve conflicts, or contact maintainers for support.
Explanation:
show
: This command displays detailed information about a specified package.package
: The name of the package you wish to know more about.
Example Output:
Name: package
Version: x.x.x
Summary: A short description of the package
Home-page: https://package-homepage.com
Author: Package Author
Author-email: author@example.com
License: License Info
Location: /path/to/package
Requires: dependencies
Install Packages from a File
Code:
pip install --requirement requirements.txt
Motivation:
When starting a project or setting up a new environment, installing dependencies via a requirements.txt
file guarantees all necessary dependencies are installed at once, replicating a known environment state efficiently.
Explanation:
--requirement
: This option specifies that the list of packages to be installed is located in a file.requirements.txt
: The file that defines the packages and their versions required for your project.
Example Output:
Collecting package-x
Downloading package-x.x.x-py2.py3-none-any.whl (size)
Collecting package-y
Downloading package-y.y.y-py2.py3-none-any.whl (size)
Installing collected packages: package-x, package-y
Successfully installed package-x.x.x package-y.y.y
Conclusion
The pip
command is an indispensable tool in the Python ecosystem, providing a straightforward interface for managing packages. By understanding and utilizing its various functionalities, developers can effortlessly maintain their Python environments and focus on building robust applications. Whether you’re installing, upgrading, or documenting dependencies, pip
offers efficient and powerful solutions to meet your development needs.