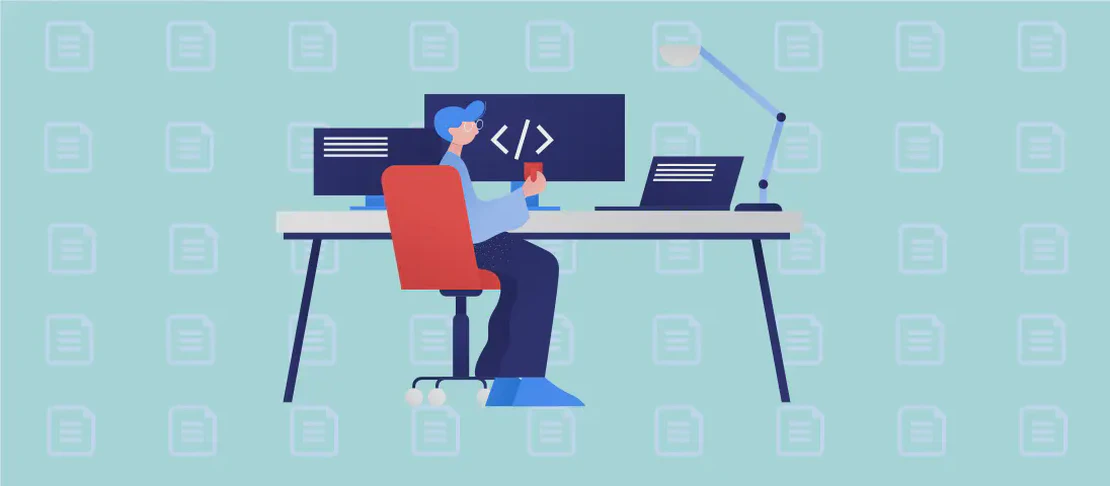
How to Use the Command 'pip freeze' (with examples)
The command pip freeze
is a valuable tool for developers working with Python. It lists all the installed packages in your Python environment, showing the exact versions currently installed. This is particularly useful when you want to share your development environment settings or ensure consistency across different setups. The output can be directed to a requirements.txt
file, which is commonly used to recreate the same environment elsewhere or to configure deployment systems.
Use case 1: List all installed packages
Code:
pip freeze
Motivation:
Listing all installed packages is essential for keeping track of dependencies in your project. It’s particularly helpful when debugging environment issues, upgrading packages, or sharing your current environment with collaborators. By generating a current list of installed packages, you can also identify unnecessary packages and clean up your environment.
Explanation:
The command pip freeze
without any arguments will enumerate all the packages installed in the current environment. Pip achieves this by traversing the site-packages directory and reporting the package names and their respective versions. This provides a comprehensive overview of all Python libraries currently in use.
Example output:
Django==3.2.8
numpy==1.21.2
requests==2.26.0
This output shows that Django version 3.2.8, NumPy version 1.21.2, and Requests version 2.26.0 are installed.
Use case 2: List installed packages and write it to the requirements.txt
file
Code:
pip freeze > requirements.txt
Motivation:
Creating a requirements.txt
file allows you to document the exact packages and their versions used in your project, promoting a reproducible environment. This is crucial for sharing your codebase with others or deploying your application, as it ensures that everyone uses the same set of dependencies, thus minimizing compatibility issues.
Explanation:
The redirection operator >
is used to pipe the output of pip freeze
into a file named requirements.txt
. This file acts as a snapshot of your current environment, capturing all installed package names and versions, which can later be used with pip install -r requirements.txt
to replicate the environment elsewhere.
Example output (contents of requirements.txt
):
Flask==2.0.1
pandas==1.3.3
SQLAlchemy==1.4.25
This file indicates that Flask version 2.0.1, Pandas version 1.3.3, and SQLAlchemy version 1.4.25 are required.
Use case 3: List installed packages in a virtual environment, excluding globally installed packages
Code:
pip freeze --local > requirements.txt
Motivation:
When working in a virtual environment, it’s vital to distinguish between packages installed locally within that environment and those available globally on the system. pip freeze --local
is used in virtual environments to ensure that the generated list only includes packages explicitly installed in that environment, thereby preventing possible conflicts with global packages.
Explanation:
The --local
flag restricts the listed packages to those installed directly in the virtual environment, excluding any global packages. This distinction ensures that your requirements.txt
file only reflects the isolated environment setup, preventing unintended dependencies from influencing other projects on your machine.
Example output (contents of requirements.txt
):
Jinja2==3.0.1
Werkzeug==2.0.1
Only packages specifically installed in the virtual environment are listed, such as Jinja2 version 3.0.1 and Werkzeug version 2.0.1.
Use case 4: List installed packages in the user-site
Code:
pip freeze --user > requirements.txt
Motivation:
Some installations are done specifically for a single user rather than system-wide. This is often the case where there are restrictions on modifying the system, or when you wish to avoid interference with system-managed software. Creating a user-scoped list of installed packages can be critical for diagnosing personal environment issues or transferring a personal setup across different machines or user accounts.
Explanation:
The --user
flag directs pip to report only the packages installed within the user’s scope. This list omits system-wide and virtual environment packages, thus providing a clear picture of modules personally added by the user.
Example output (contents of requirements.txt
):
matplotlib==3.4.3
pytz==2021.1
This file reveals packages installed by the user, such as Matplotlib version 3.4.3 and pytz version 2021.1.
Use case 5: List all packages, including pip
, distribute
, setuptools
, and wheel
Code:
pip freeze --all > requirements.txt
Motivation:
By default, pip freeze
excludes certain core packages like pip itself, distribute, setuptools, and wheel. However, in some scenarios, such as debugging or ensuring a pristine environment recreation, knowing all installed packages, including these core tools, can be essential.
Explanation:
Using the --all
flag alongside pip freeze
includes the otherwise excluded packages in the output. This ensures a thorough capture of your Python environment’s package landscape, capturing every installed component regardless of its nature.
Example output (contents of requirements.txt
):
pip==21.2.4
setuptools==57.5.0
wheel==0.36.2
virtualenv==20.7.2
This output records the current versions of pip, setuptools, wheel, and any other non-default installations such as virtualenv, revealing a comprehensive account of all packages, including base utilities.
Conclusion:
The pip freeze
command is a versatile tool, aiding developers in managing and documenting their Python project dependencies. From listing installed packages to creating requirements files tailored to virtual environments, users, or complete setups, pip freeze
streamlines the process of maintaining consistency and reliability across various development and production environments.