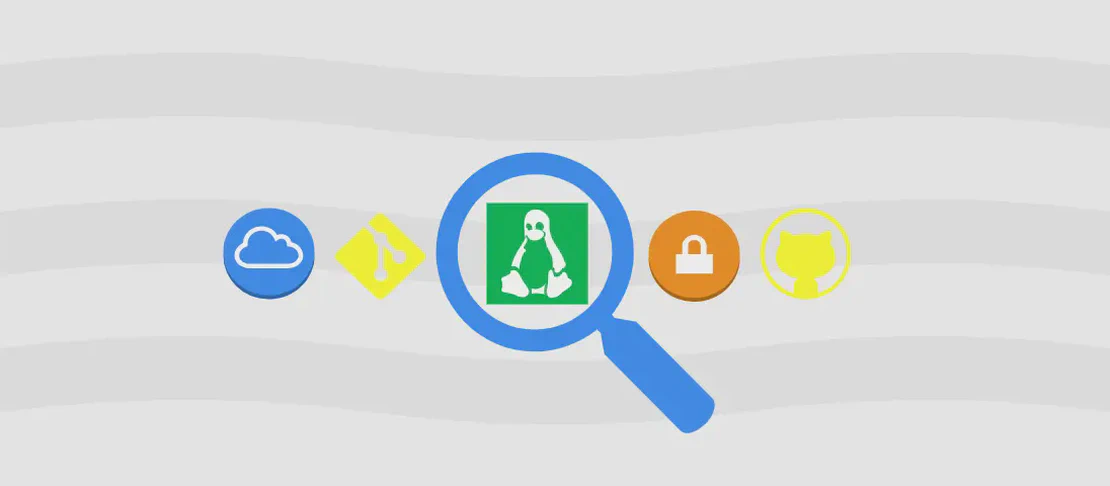
Install Python Packages (with examples)
1: Install a package
Code:
pip install package
Motivation:
This use case is used when you want to install a specific Python package on your system.
Explanation:
The command pip install package
is used to install a specific Python package. The word “package” should be replaced with the name of the package you want to install. This command will download and install the latest version of the package from the Python Package Index (PyPI).
Example Output:
Collecting package
Downloading package-1.0.0.tar.gz (10 kB)
Building wheels for collected packages: package
Building wheel for package (setup.py): started
Building wheel for package (setup.py): finished with status 'done'
Created wheel for package: filename-1.0.0-py3-none-any.whl (12 kB)
Stored in directory: /Users/username/Library/Caches/pip/wheels/3d/61/2d/4b26a2341b3ae2aeb79e663398084d5a9e27a89f920b291bcf
Successfully built package
Installing collected packages: package
Successfully installed package-1.0.0
2: Install a specific version of a package
Code:
pip install package==version
Motivation:
Sometimes, you may need to install a specific version of a package instead of the latest version. This could be due to compatibility issues or specific feature requirements.
Explanation:
The command pip install package==version
is used to install a specific version of a package. Replace “package” with the name of the package you want to install and “version” with the desired version number. This command will download and install the specified version of the package from PyPI.
Example Output:
Collecting package==1.0.0
Downloading package-1.0.0.tar.gz (10 kB)
Building wheels for collected packages: package
Building wheel for package (setup.py): started
Building wheel for package (setup.py): finished with status 'done'
Created wheel for package: filename-1.0.0-py3-none-any.whl (12 kB)
Stored in directory: /Users/username/Library/Caches/pip/wheels/3d/61/2d/4b26a2341b3ae2aeb79e663398084d5a9e27a89f920b291bcf
Successfully built package
Installing collected packages: package
Successfully installed package-1.0.0
3: Install packages listed in a file
Code:
pip install --requirement path/to/requirements.txt
Motivation:
When working on a project, it is common to have a list of required packages stored in a text file. This use case allows you to easily install all the required packages at once.
Explanation:
The command pip install --requirement path/to/requirements.txt
is used to install packages listed in a requirements.txt file. Replace “path/to/requirements.txt” with the actual path to your requirements.txt file. This command will read the file and install all the packages specified in the file.
Example Output:
Collecting package1==1.0.0
Downloading package1-1.0.0.tar.gz (10 kB)
Building wheels for collected packages: package1
Building wheel for package1 (setup.py): started
Building wheel for package1 (setup.py): finished with status 'done'
Created wheel for package1: filename-1.0.0-py3-none-any.whl (12 kB)
Stored in directory: /Users/username/Library/Caches/pip/wheels/3d/61/2d/4b26a2341b3ae2aeb79e663398084d5a9e27a89f920b291bcf
Successfully built package1
Installing collected packages: package1
Successfully installed package1-1.0.0
Collecting package2==2.0.0
Downloading package2-2.0.0.tar.gz (10 kB)
Building wheels for collected packages: package2
Building wheel for package2 (setup.py): started
Building wheel for package2 (setup.py): finished with status 'done'
Created wheel for package2: filename-2.0.0-py3-none-any.whl (12 kB)
Stored in directory: /Users/username/Library/Caches/pip/wheels/3d/61/2d/4b26a2341b3ae2aeb79e663398084d5a9e27a89f920b291bcf
Successfully built package2
Installing collected packages: package2
Successfully installed package2-2.0.0
4: Install packages from an URL or local file archive (.tar.gz | .whl)
Code:
pip install --find-links url|path/to/file
Motivation:
There may be cases where you want to install packages from a specific URL or a local file archive (.tar.gz or .whl) instead of directly from PyPI. This could be useful when working with custom or unlisted packages.
Explanation:
The command pip install --find-links url|path/to/file
is used to install packages from an URL or local file archive. Replace “url|path/to/file” with the URL from where you want to install the package or the path to the local file archive. This command will look for the package in the specified location and install it.
Example Output:
Looking in links: <URL>
Processing <file>
Collecting package3==3.0.0
Downloading package3-3.0.0.tar.gz (10 kB)
Building wheels for collected packages: package3
Building wheel for package3 (setup.py): started
Building wheel for package3 (setup.py): finished with status 'done'
Created wheel for package3: filename-3.0.0-py3-none-any.whl (12 kB)
Stored in directory: /Users/username/Library/Caches/pip/wheels/3d/61/2d/4b26a2341b3ae2aeb79e663398084d5a9e27a89f920b291bcf
Successfully built package3
Installing collected packages: package3
Successfully installed package3-3.0.0
5: Install the local package in the current directory in develop (editable) mode
Code:
pip install --editable .
Motivation:
When developing a package, it is beneficial to install the package in “editable” mode. This means that changes made to the code will be immediately reflected without needing to reinstall the package.
Explanation:
The command pip install --editable .
is used to install the local package in the current directory in develop (editable) mode. This command should be executed from the root directory of the package. It will create a symlink to the package’s code, allowing changes to be immediately visible when importing the package.
Example Output:
Processing <current_directory>
Installing collected packages: package4
Running setup.py develop for package4
Successfully installed package4
By following these examples, you can effectively use the pip install
command to install Python packages, whether you need to install a specific package, a specific version, packages listed in a file, packages from an URL or local file archive, or install a local package in develop (editable) mode.