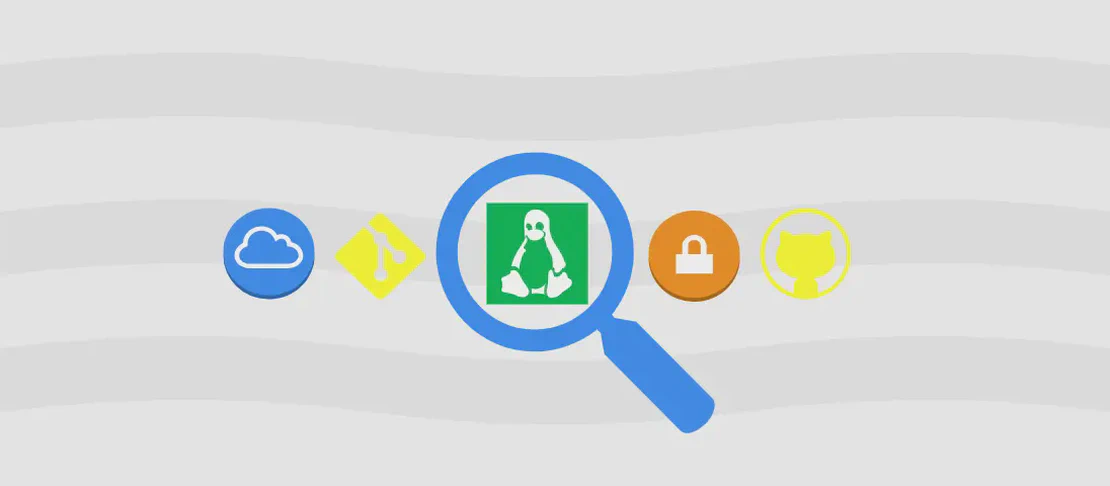
Mastering Python Package Management with pip3 (with examples)
Pip3 is a powerful and versatile command-line utility used for managing Python packages. It is the official package manager for Python, allowing developers to easily install, upgrade, and manage project dependencies in their environments. Pip3 ensures smooth collaboration, reproducibility, and consistency across different development setups by handling the installation and management of Python packages effortlessly.
Use case 1: Install a package
Code:
pip3 install package
Motivation: Installing packages is the foundational step in leveraging external libraries and tools in Python programming. This is essential for enriching your development environment with needed functionalities from third-party libraries, thus saving time and effort by reusing existing code.
Explanation:
pip3
: This signals the use of the Python 3 version of the pip command.install
: This argument directs pip3 to fetch and install the specified package.package
: Replace this placeholder with the actual name of the package you wish to install.
Example Output:
Collecting package-name
Downloading package-name-package-version.tar.gz (size KB)
Installing collected packages: package-name
Successfully installed package-name-package-version
Use case 2: Install a specific version of a package
Code:
pip3 install package==version
Motivation: It is sometimes crucial to install a specific version of a package, especially when newer versions introduce breaking changes, or when you want to ensure compatibility with other components in your project.
Explanation:
package==version
: Here,package
needs to be replaced with the package name andversion
should be the exact version number desired, enabling fine-grained control over package installs.
Example Output:
Collecting package-name==desired-version
Downloading package-name-package-desired-version.tar.gz (size KB)
Installing collected packages: package-name
Successfully installed package-name-desired-version
Use case 3: Upgrade a package
Code:
pip3 install --upgrade package
Motivation: Keeping packages up-to-date ensures that you benefit from the latest features, optimizations, and security patches. Upgrading packages is a best practice to maintain the health and efficiency of your software over time.
Explanation:
--upgrade
: This argument tells pip3 to update the package to the latest version.package
: The package name is provided here to specify which one needs to be upgraded.
Example Output:
Collecting package-name
Downloading package-name-package-new-version.tar.gz (size KB)
Installing collected packages: package-name
Found existing installation: package-name-old-version
Uninstalling package-name-old-version:
Successfully uninstalled package-name-old-version
Successfully installed package-name-new-version
Use case 4: Uninstall a package
Code:
pip3 uninstall package
Motivation: Over time, some packages might become redundant or unnecessary, particularly when the project’s requirements evolve. Uninstalling such packages helps in maintaining a clean environment, avoiding bloat and potential conflicts.
Explanation:
uninstall
: This argument indicates the intent to remove a package.package
: This replaces the placeholder to signify which package should be removed from the environment.
Example Output:
Found existing installation: package-name package-version
Uninstalling package-name-package-version:
Successfully uninstalled package-name-package-version
Use case 5: Save the list of installed packages to a file
Code:
pip3 freeze > requirements.txt
Motivation: Capturing the current state of installed packages is vital for project reproducibility. Saving this information in a file allows for easy sharing with collaborators or deploying the same setup in different environments, offering consistency across setups.
Explanation:
freeze
: Lists all installed packages and their versions, creating a snapshot of the environment.>
: A shell operator that directs the output (package list) to be saved into a specified file.requirements.txt
: A conventional filename that stores the list of packages, suitable for version control and sharing.
Example Output:
In the file requirements.txt:
package-name==package-version
another-package==another-version
Use case 6: Install packages from a file
Code:
pip3 install --requirement requirements.txt
Motivation: Installing packages from a requirements file lets you replicate environments and seamlessly set up instances with all necessary dependencies. This is particularly useful in deploying applications and onboarding new team members in projects.
Explanation:
--requirement
: Indicates the command should read from a file that lists all packages to be installed.requirements.txt
: The name of the file containing the package list with specified versions.
Example Output:
Collecting package-name
Downloading package-name-package-version.tar.gz (size KB)
Installing collected packages: package-name, another-package
Successfully installed package-name-package-version another-package-another-version
Use case 7: Show installed package info
Code:
pip3 show package
Motivation: Viewing detailed information about an installed package assists in understanding its metadata, dependencies, and installation location. Such insight can support debugging or decision-making when considering upgrades or replacements.
Explanation:
show
: Directs pip to display detailed information about a package.package
: Replace this with the package name for which you want detailed information.
Example Output:
Name: package-name
Version: package-version
Summary: A brief summary of the package
Home-page: URL
Author: Author Name
Author-email: author@example.com
License: License Type
Location: /path/to/package/location
Requires: dependency1, dependency2
Conclusion
Pip3 is a comprehensive tool for managing Python packages, offering various functionalities that cater to the full lifecycle of package management. From installation, upgrading, and uninstalling to replicating environments and inspecting package details, pip3 supports seamless, efficient management of dependencies, ensuring reliable and consistent Python development environments.