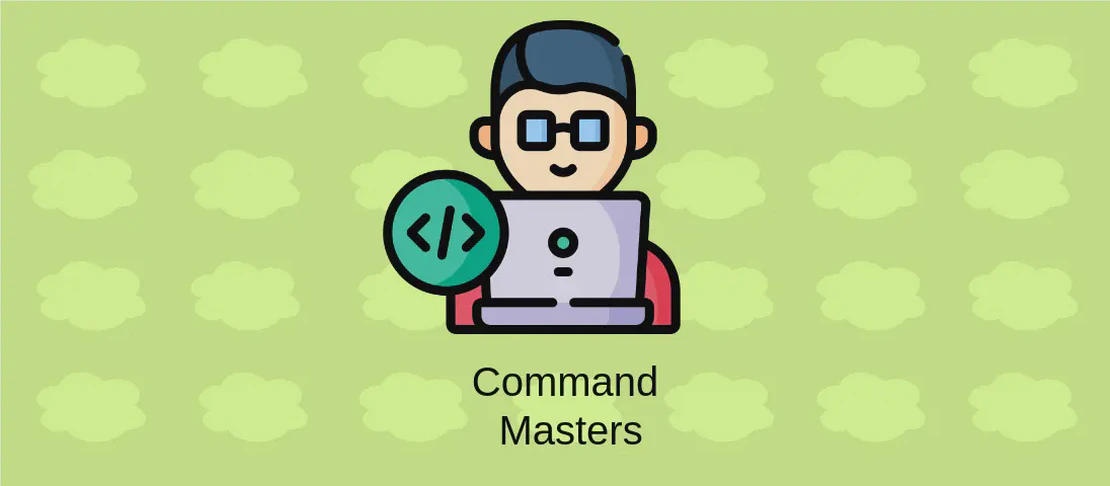
How to Use Pipenv (with Examples)
Pipenv is a tool that aims to bring the best of all packaging worlds to the Python world. It manages virtual environments and dependencies for Python projects in a simple and unified format. By combining the functionalities of pip
, Pipfile
, and virtual environments, Pipenv simplifies the development process by categorizing packages into development and production dependencies and streamlining the handling of these dependencies.
Create a New Project
Code:
pipenv
Motivation: Creating a new project is the first step in any development process. By initializing a new development environment, you set up an isolated workspace where all dependencies specific to the project can be managed separately from other projects and system packages. This helps avoid dependency conflicts and streamline the project setup process.
Explanation:
Running pipenv
in a directory initializes a new virtual environment and creates two essential files: Pipfile
and Pipfile.lock
. These files help track project dependencies and ensure reproducible installations on other systems.
Example Output:
Creating a Pipfile for this project…
Create a New Project Using Python 3
Code:
pipenv --three
Motivation: As Python 2 has reached the end of its life, it is crucial to explicitly specify Python 3 to ensure your projects are future-proof. Initiating a project with Python 3 ensures compatibility with the latest Python features and libraries.
Explanation:
The --three
flag is provided to explicitly instruct pipenv
to create a virtual environment using Python 3. This is highly beneficial when transitioning projects from Python 2 to Python 3 or when starting new projects to take advantage of Python 3’s capabilities.
Example Output:
Creating a virtualenv using python3 in /your/project/path…
Install a Package
Code:
pipenv install package
Motivation: Installing packages is a fundamental part of development as it allows you to leverage external libraries and tools to enhance your project’s functionality. Using pipenv to manage package installations helps maintain dependencies efficiently.
Explanation:
pipenv install package
installs the specified package into the project’s virtual environment and updates the Pipfile
to include this new dependency. It also updates the Pipfile.lock
to record the exact package version installed, ensuring consistent builds.
Example Output:
Installing package…
Adding package to Pipfile's [packages]…
Install All the Dependencies for a Project
Code:
pipenv install
Motivation:
When working on a collaborative project, ensuring everyone has the exact same setup is critical. Installing all dependencies listed in the Pipfile.lock
ensures your development environment matches other team members’, including the development and production servers.
Explanation:
Running pipenv install
without specifying a package checks the Pipfile
and installs all the listed packages, ensuring your environment is fully set up according to the project’s requirements.
Example Output:
Installing dependencies from Pipfile…
Locking dependencies…
Install All the Dependencies for a Project (Including Dev Packages)
Code:
pipenv install --dev
Motivation: Developing software often involves tools and libraries used exclusively during the development phase, such as linters, testing frameworks, or compilers. Differentiating these from production dependencies is crucial for optimized performance and security on deployment.
Explanation:
The --dev
flag adds development dependencies to the installation process. This ensures that any packages specified under the [dev-packages]
section in the Pipfile
are also installed.
Example Output:
Installing dependencies from Pipfile and dev-packages from Pipfile…
Locking dependencies…
Uninstall a Package
Code:
pipenv uninstall package
Motivation: Over time, some packages may become obsolete or unnecessary for a project. Efficiently removing them helps keep the environment clean and maintainable.
Explanation:
This command removes the specified package from the virtual environment and updates the Pipfile
and Pipfile.lock
to reflect this uninstallation. It ensures that the project remains lean and reduces the risk of unforeseen issues caused by outdated packages.
Example Output:
Uninstalling package…
Removing package from Pipfile…
Start a Shell Within the Created Virtual Environment
Code:
pipenv shell
Motivation: Running a shell within a virtual environment isolates your session from the global Python environment, ensuring that you are using the project’s dependencies. This is essential for testing and debugging the project in a controlled and consistent environment.
Explanation:
pipenv shell
activates the virtual environment and opens a new shell session. This allows you to run commands and scripts using the Python interpreter and libraries specific to the current project.
Example Output:
Launching subshell in virtual environment…
projectname @ /path/to/project $
Generate a requirements.txt
for a Project
Code:
pipenv lock --requirements
Motivation:
While Pipenv uses Pipfile
and Pipfile.lock
, many deployment systems still rely on requirements.txt
. Generating this file is crucial for maintaining compatibility with these systems, especially in continuous integration and deployment pipelines.
Explanation:
The command generates a requirements.txt
file from the Pipfile.lock
, listing all installed packages and their versions. This allows for easy integration with existing tools and workflows that depend on requirements.txt
.
Example Output:
Creating requirements.txt from Pipfile.lock...
Conclusion:
Pipenv provides a streamlined and efficient way to manage Python environments and dependencies, catering to various needs throughout the software development life cycle. From initializing projects with specific Python versions to handling development-specific dependencies and exporting compatibility files, Pipenv simplifies the process, reducing potential issues and enhancing collaboration among team members. By understanding and utilizing these commands effectively, developers can maintain cleaner environments and produce more robust software.