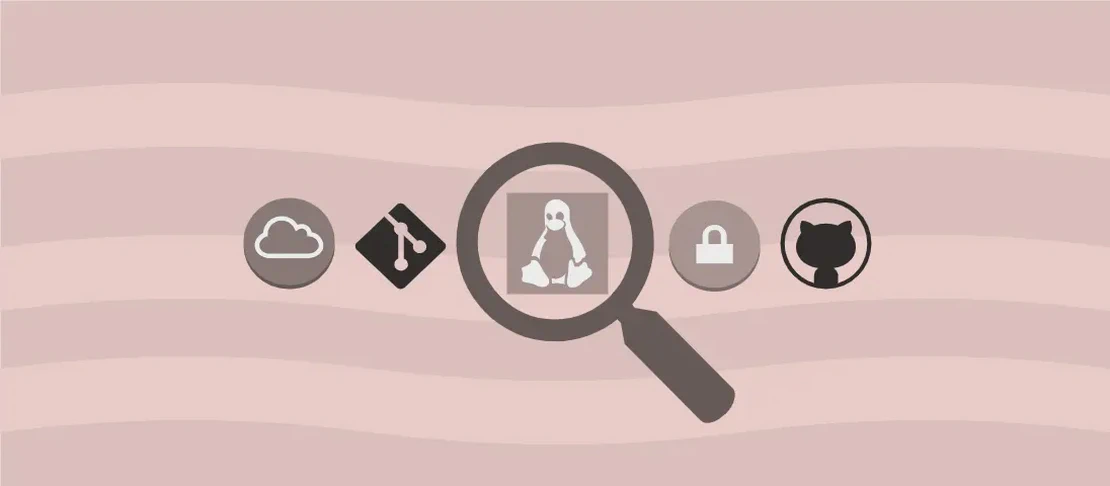
How to Use the Command 'pipx' (with examples)
pipx
is a powerful command-line tool that allows you to install and run Python applications in isolated environments with ease. This ensures that the Python packages you install do not interfere with each other or with your system’s Python packages. By using a dedicated virtual environment for each application, you can manage Python command-line tools more flexibly and keep them up-to-date without dealing with dependency issues.
Use case 1: Run an app in a temporary virtual environment
Code:
pipx run pycowsay moo
Motivation:
Sometimes, you may want to run a Python application once without permanently installing it on your system. pipx
helps achieve this by creating a temporary virtual environment that automatically cleans up after the app exits. This is especially useful for running lightweight scripts or utilities just to see their output or effectiveness without cluttering your setup.
Explanation:
pipx
: The command itself used to run or install Python applications.run
: A subcommand that temporarily installs and runs a package.pycowsay
: The package or application you want to execute.moo
: An argument passed directly to the specified application (here,pycowsay
).
Example Output:
--------
< moo >
--------
\
\
^__^
(oo)\_______
(__)\ )\/\
||----w |
|| ||
Use case 2: Install a package in a virtual environment and add entry points to path
Code:
pipx install package
Motivation:
When you frequently use a specific Python application, installing it through pipx
helps maintain an isolated environment, meaning dependencies wonβt cross with other packages or system libraries. Additionally, pipx
ensures the application’s entry points are added to your systemβs PATH, allowing you to use the app’s command-line interface without needing to navigate to its install directory.
Explanation:
pipx
: The tool to install the application.install
: Subcommand used to install a package into its own environment.package
: Placeholder for the actual package name you wish to install.
Example Output:
Installed package package v1.0.0, location /home/user/.local/pipx/venvs/package
These apps are now globally available
- package-cli
done! β¨ π β¨
Use case 3: List installed packages
Code:
pipx list
Motivation:
Managing various installed packages and being aware of what’s in your system can be difficult when dealing with numerous Python applications. pipx list
is beneficial for auditing these installations, showing you a clear overview of the apps managed by pipx, their versions, and environments.
Explanation:
pipx
: The command used for package management in isolated environments.list
: Subcommand that outputs a list of all packages currently installed and managed bypipx
.
Example Output:
venvs are in /home/user/.local/pipx/venvs
apps are exposed on your $PATH at /home/user/.local/bin
package v1.0.0, Python 3.8.5
other-package v2.3.4, Python 3.9.1
Use case 4: Run an app in a temporary virtual environment with a package name different from the executable
Code:
pipx run --spec httpx-cli httpx http://www.github.com
Motivation:
At times, the desired executable name may differ from the package name due to maintenance or app structuring reasons. This use case highlights how pipx
accommodates that difference, letting developers specify the correct executable alias while running applications so that they can link the correct libraries and execute their application accurately.
Explanation:
pipx
: Core tool used for managing Python apps.run
: Subcommand indicating a temporary run without permanent installation.--spec httpx-cli
: Specifies the name of the package, distinct from the executable name.httpx
: The executable that should be run from the specified package.http://www.github.com
: The argument passed to the executable, here URL input tohttpx
.
Example Output:
[2023-03-15 12:34:56,789] INFO: Sending GET request to http://www.github.com
[2023-03-15 12:34:56,789] INFO: Response status: 200 OK
Use case 5: Inject dependencies into an existing virtual environment
Code:
pipx inject package dependency1 dependency2 ...
Motivation:
When you need to add specific dependencies to an already existing and installed package, pipx inject
provides a straightforward method to manage these without needing to recreate or modify the environment manually. This is particularly helpful when updating certain functionalities of the app or when certain features need additional modules.
Explanation:
pipx
: The command facilitating package management in isolated environments.inject
: Subcommand used to add new dependencies to an installed package’s environment.package
: Name of the installed package environment targeted for injection.dependency1 dependency2 ...
: One or more dependencies you want added.
Example Output:
Inserting dependencies into package...
Dependency dependency1 installed.
Dependency dependency2 installed.
done! β¨ π β¨
Use case 6: Install a package in a virtual environment with pip arguments
Code:
pipx install --pip-args='pip-args' package
Motivation:
Sometimes you might need to pass additional arguments to pip when installing a package, such as specifying a source for the package or using a particular version. pipx
provides this flexibility, thus accommodating advanced installation scenarios which may be required in environments with specific constraints or policies.
Explanation:
pipx
: The command-line tool used to install the Python package.install
: Subcommand to set a package in an isolated environment.--pip-args='pip-args'
: Additional pip arguments encased in quotes, providing supplementary instructions or parameters for the installation.package
: Placeholder for the actual package you’re installing.
Example Output:
Running pip with arguments 'pip-args'
Installed package with pip arguments, location /home/user/.local/pipx/venvs/package
done! β¨ π β¨
Use case 7: Upgrade/reinstall/uninstall all installed packages
Code:
pipx upgrade-all|uninstall-all|reinstall-all
Motivation:
Managing multiple packages over time can lead to outdated, misconfigured or unnecessary installations. pipx
consolidates several administrative operations here, enabling users to update, remove, or reinstall their packages comprehensively and efficiently. This ensures all packages are current, useful, or removed in bulk without requiring individual attention.
Explanation:
pipx
: The framework for managing separate Python app installations.upgrade-all
: Subcommand to update all installed packages.uninstall-all
: Subcommand to remove all installed packages from the environment.reinstall-all
: Subcommand to refresh the installation of all packages, rectifying any issues from prior installations.
Example Output for upgrade-all
:
Upgrading installed packages:
- package1 upgraded from 1.0.0 to 1.1.0
- package2 is already up-to-date
done! β¨ π β¨
Example Output for uninstall-all
:
Uninstalling all packages:
- package1 removed
- package2 removed
done! β¨ π β¨
Example Output for reinstall-all
:
Reinstalling all packages:
- package1 reinstalled
- package2 reinstalled
done! β¨ π β¨
Conclusion:
pipx
offers Python users a robust toolset for managing command-line applications effectively and independently. These examples illustrate how it facilitates not only simplified installations but also provides flexible, maintainable, and overall robust management of Python environments directly from the command line.