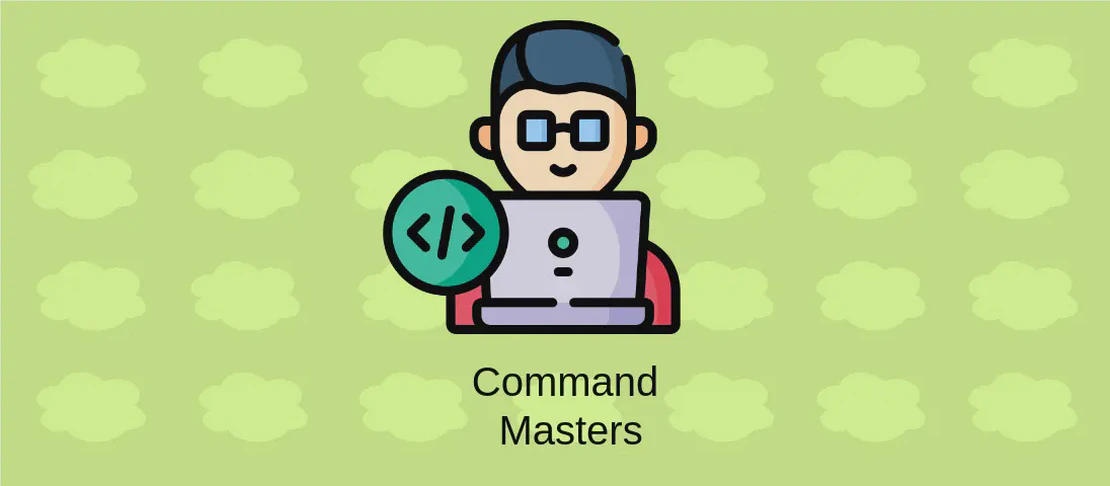
Mastering PM2 for Node.js Process Management (with examples)
PM2 is a production-ready process manager for Node.js applications. It is designed to facilitate seamless application deployment and management, thereby ensuring smooth performance, robust monitoring, and efficient logging. Its primary function is to manage application processes, allowing developers to keep their Node.js applications alive forever, reload them without downtime, and facilitate exceptional application monitoring. Let’s delve into several use cases where PM2 proves invaluable, demonstrating how to start, monitor, and maintain your Node.js applications efficiently.
Start a process with a name that can be used for later operations
Code:
pm2 start app.js --name application_name
Motivation: Naming a process is crucial for easily managing, stopping, restarting, or monitoring your application. When you assign a name to the process, you can perform operations on the process later without having to remember its ID, which can be cumbersome, especially when dealing with multiple processes.
Explanation:
pm2 start app.js
is a command to initiate a process in PM2.app.js
refers to your Node.js application that you wish to run.--name application_name
allows you to assign a human-readable name (application_name
) to your process. This alias makes it easier to perform future operations on this specific application without relying on potentially changing process IDs.
Example output:
[PM2] Starting app.js in fork_mode (1 instances)
[PM2] Done.
┌────────────┬────┬──────┬────────┬────────────┐
│ App name │ id │ mode │ status │ restart(s) │
├────────────┼────┼──────┼────────┼────────────┤
│ application_name │ 0 │ fork │ online │ 0 │
└────────────┴────┴──────┴────────┴────────────┘
List processes
Code:
pm2 list
Motivation: Listing processes allows you to view all currently managed applications by PM2. This is invaluable when you have multiple applications running, as it provides a quick overview of their current states, including metrics like status, CPU usage, memory consumption, and number of restarts.
Explanation:
pm2 list
is a simple command that outputs a snapshot of all processes currently managed by PM2. There are no additional arguments required; it gives information critical for regular monitoring and management.
Example output:
┌────────────┬────┬──────┬────────┬─────┬──────┬────────┬──────┬───────┐
│ App name │ id │ mode │ status │ cpu │ mem │ user │ watching │ restart │
├────────────┼────┼──────┼────────┼─────┼──────┼────────┼──────┼───────┤
│ app1 │ 0 │ fork │ online │ 0% │ 43MB │ user │ disabled │ 0 │
│ app2 │ 1 │ fork │ online │ 1% │ 65MB │ user │ disabled │ 1 │
└────────────┴────┴──────┴────────┴─────┴──────┴────────┴───────┴───────┘
Monitor all processes
Code:
pm2 monit
Motivation: Process monitoring is key to ensuring optimal application performance. The pm2 monit
command opens a dashboard-like interface directly in the terminal, providing real-time metrics of all PM2-managed applications. This interactive view is essential for in-depth monitoring and troubleshooting of issues as they occur.
Explanation:
pm2 monit
engages a real-time terminal-based dashboard displaying metrics like CPU and memory usage, events occurring in the application, and other performance indicators. This command does not require additional arguments.
Example output:
[PM2] Monit Dashboard
┌───────────────┬───────────────────────┬───────────────┐
│ App name │ CPU (%) │ Memory Usage (RSS) │ Status │
├───────────────┼───────────────┼───────────────┤
│ app1 │ 1% │ 52 MB │ online │
│ app2 │ 3% │ 75 MB │ online │
└───────────────┴───────────────┴───────────────┘
Stop a process
Code:
pm2 stop application_name
Motivation: Stopping a process is sometimes necessary for freeing up resources, performing maintenance, or updating part of the application without taking the whole server down. This command allows you to suspend specific applications without affecting other services managed by PM2.
Explanation:
pm2 stop
is used to halt a particular application. Theapplication_name
afterstop
indicates the target process by its assigned name, offering a straightforward approach to managing stopping procedures without identifying the process by an ID number.
Example output:
[PM2] Stopping application_name
[PM2] App [application_name] stopped
┌────────────┬────┬──────┬────────┬─────┬──────┬────────┬──────┬───────┐
│ App name │ id │ mode │ status │ cpu │ mem │ user │ watching │ restart │
├────────────┼────┼──────┼────────┼─────┼──────┼────────┼──────┼───────┤
│ application_name │ 0 │ fork │ stopped │ 0% │ 0MB │ user │ disabled │ 0 │
└────────────┴────┴──────┴────────┴─────┴──────┴────────┴───────┴───────┘
Restart a process
Code:
pm2 restart application_name
Motivation: When updates to your application code or its environment occur, a restart is often required. The pm2 restart
command makes it easy to reload an application with the new changes without downtime, ensuring deployment efficiency and continuity in service.
Explanation:
pm2 restart
specifically targets an application to restart. You specify theapplication_name
, which you’ve previously set, to identify which app needs reloading, thus ensuring that only the desired process restarts.
Example output:
[PM2] Restarting application_name
[PM2] Application restarted
┌────────────┬────┬──────┬────────┬─────┬──────┬────────┬──────┬───────┐
│ App name │ id │ mode │ status │ cpu │ mem │ user │ watching │ restart │
├────────────┼────┼──────┼────────┼─────┼──────┼────────┼──────┼───────┤
│ application_name │ 0 │ fork │ online │ 0% │ 48MB │ user │ disabled │ 1 │
└────────────┴────┴──────┴────────┴─────┴──────┴────────┴───────┴───────┘
Dump all processes for resurrecting them later
Code:
pm2 save
Motivation: Saving the current state of all managed processes is essential, especially when making changes that could impact your server, such as restarting the host machine. The pm2 save
command allows you to retain the configuration of your apps, ensuring they can easily be restored to their original state if needed.
Explanation:
pm2 save
does not require any additional arguments. This simple but powerful command saves the state of all running processes to a JSON file. When needed, this file can be used to recreate all previously running applications at their last known state.
Example output:
[PM2] Saving process list
[PM2] Process list saved successfully
Resurrect previously dumped processes
Code:
pm2 resurrect
Motivation: Resurrecting processes is the key to quickly recovering your previous application states after a machine reboot or server crash. With a single command, you can restore all processes to their state prior to saving, minimizing downtime and administrative overhead.
Explanation:
pm2 resurrect
uses the saved JSON file created bypm2 save
. This action reinitializes all processes according to their prior configuration, launching each application to continue from where they left off.
Example output:
[PM2] Resurrecting processes
[PM2] Process list restored
┌────────────┬────┬──────┬────────┬─────┬──────┬────────┬──────┬───────┐
│ App name │ id │ mode │ status │ cpu │ mem │ user │ watching │ restart │
├────────────┼────┼──────┼────────┼─────┼──────┼────────┼──────┼───────┤
│ application_name │ 0 │ fork │ online │ 0% │ 50MB │ user │ disabled │ 1 │
└────────────┴────┴──────┴────────┴─────┴──────┴────────┴───────┴───────┘
Conclusion:
PM2 stands out as a must-have tool for managing Node.js applications effectively. Through its command-line utility, it simplifies complex tasks such as starting, stopping, and monitoring processes while providing robust solutions for fault tolerance and process resurrection. The use cases showcased above highlight PM2’s versatility and importance in a production environment, ensuring that applications are always performing at their peak and offering a seamless path for recovery and configuration management.