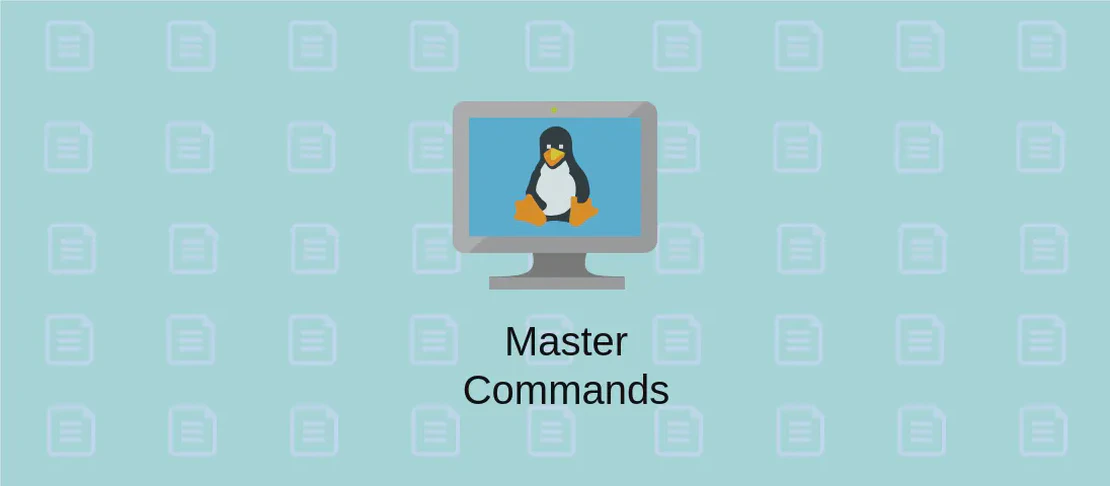
How to use the 'pnpm' Package Manager (with examples)
pnpm is a fast, disk space-efficient package manager for Node.js. It serves as an alternative to other Node.js package managers like npm and Yarn and is particularly appreciated for its ability to save disk space by linking identical packages instead of duplicating them. pnpm ensures projects are fast and reduce redundancies, boasting significant improvements in disk space usage and installation times.
Create a package.json
file
Code:
pnpm init
Motivation:
Creating a package.json
file is the foundational step to managing a Node.js project. This file contains information about your project, scripts, and lists of dependencies, and is crucial for the seamless operation of Node.js applications. Utilizing pnpm init
simplifies the creation of this file by guiding you through a series of prompts about your project’s details.
Explanation:
init
: This flag initializes a new pnpm-based project, creating apackage.json
file that includes the basic structure for your project metadata and dependencies.
Example Output:
This utility will walk you through creating a package.json file.
Press ^C at any time to quit.
package name: (your-app)
version: (1.0.0)
description: Your app description
entry point: (index.js)
test command:
git repository:
keywords:
author:
license: (ISC)
About to write to /path/to/your/project/package.json:
{
"name": "your-app",
"version": "1.0.0",
"description": "Your app description",
"main": "index.js",
...
}
Download all the packages listed as dependencies in package.json
Code:
pnpm install
Motivation:
When you start working on an existing Node.js project, installing all the required dependencies is necessary to ensure the project functions correctly. pnpm install
reads through the package.json
file and downloads the specified packages, enabling developers to quickly set up their development environments.
Explanation:
install
: This command reads thepackage.json
and downloads all the packages mentioned under dependencies and devDependencies, making them available for use in your application.
Example Output:
Packages: +100
...
Resolving: total 100, reused 100, downloaded 0, progress: resolved 100, reused 95, downloaded 5, done
dependencies have been linked in node_modules
Download a specific version of a package and add it to the list of dependencies in package.json
Code:
pnpm add module_name@version
Motivation:
Sometimes, specific versions of a package are required to maintain version compatibility or to leverage certain features that newer or older versions might not have. The pnpm add
command allows developers to specify and lock a particular version of a package, adding it directly to the dependencies list.
Explanation:
add
: This command is used to fetch and install new packages.module_name@version
: This is the specific notation used to specify the module’s name and its desired version.
Example Output:
Packages: +1
Resolving: total 1, reused 0, downloaded 1, progress: resolved 1, reused 0, downloaded 1, done
Download a package and add it to the list of [D]ev dependencies in package.json
Code:
pnpm add -D module_name
Motivation:
Development dependencies are packages only required during the development phase and not in the production environment. By using pnpm add -D
, developers can categorize these packages correctly, keeping the production build lightweight and performance-optimized.
Explanation:
add
: Initiates the installation of a new package.-D
: This option specifies that the package is being added as a devDependency, meaning it’s only utilized during development.
Example Output:
Packages: +1
Resolving: total 1, reused 0, downloaded 1, progress: resolved 1, reused 0, downloaded 1, done
Download a package and install it [g]lobally
Code:
pnpm add -g module_name
Motivation:
There are situations where a Node.js package is needed across various projects or for command-line utilities. pnpm add -g
installs the module globally, making it accessible from anywhere on the system without needing to reinstall it for each individual project.
Explanation:
add
: This command handles the installation of the specified package.-g
: This flag sets the installation scope to global, allowing the package to be used across the entire system.
Example Output:
Packages: +1
...
Resolving: total 1, reused 0, downloaded 1, progress: resolved 1, reused 0, downloaded 1, done
/usr/local/bin/module_name
Uninstall a package and remove it from the list of dependencies in package.json
Code:
pnpm remove module_name
Motivation:
Over time, certain packages may become obsolete, or you might want to replace them with alternatives. pnpm remove
facilitates the removal of packages and ensures the package.json
file reflects this change by deleting the corresponding entry from the dependencies list.
Explanation:
remove
: This command is used to uninstall a package. It deletes the package’s files and references frompackage.json
.
Example Output:
Packages: -1
dependencies have been de-linked in node_modules
Print a tree of locally installed modules
Code:
pnpm list
Motivation:
Understanding the hierarchical structure of installed packages in a project can assist developers in troubleshooting or optimizing their dependencies. By providing a detailed view of the package tree, pnpm list
allows developers to verify dependency installations and their versions.
Explanation:
list
: Displays a hierarchical structure of the project’s dependencies, showing how each package is related.
Example Output:
my-app@1.0.0 /path/to/my/project
├── package-a@1.0.0
└── package-b@2.0.0
└── package-c@1.0.0
List top-level [g]lobally installed modules
Code:
pnpm list -g --depth=0
Motivation:
Globally installed packages might sometimes need auditing or updates. Using pnpm list -g --depth=0
, developers can easily view a summary of all globally available top-level packages, which is helpful for maintenance and ensuring the availability of necessary command-line tools.
Explanation:
list
: Lists the installed packages.-g
: Specifies that the listing should focus on globally installed packages.--depth=0
: Limits the output to top-level packages only, excluding nested dependencies for clarity.
Example Output:
Pilots-Env@ /Users/yourname/.pnpm-global/2.22.1
├── module_1@3.0.0
├── module_2@4.0.0
└── module_3@5.0.0
Conclusion:
pnpm offers a variety of commands that simplify the management of Node.js project dependencies. By providing functionality ranging from project initialization to installing and uninstalling packages, pnpm ensures that developers have efficient and space-saving methods of managing their project’s dependencies. This fast package manager not only economizes disk space but also optimizes workflows, making it a valuable tool for modern Node.js developers.