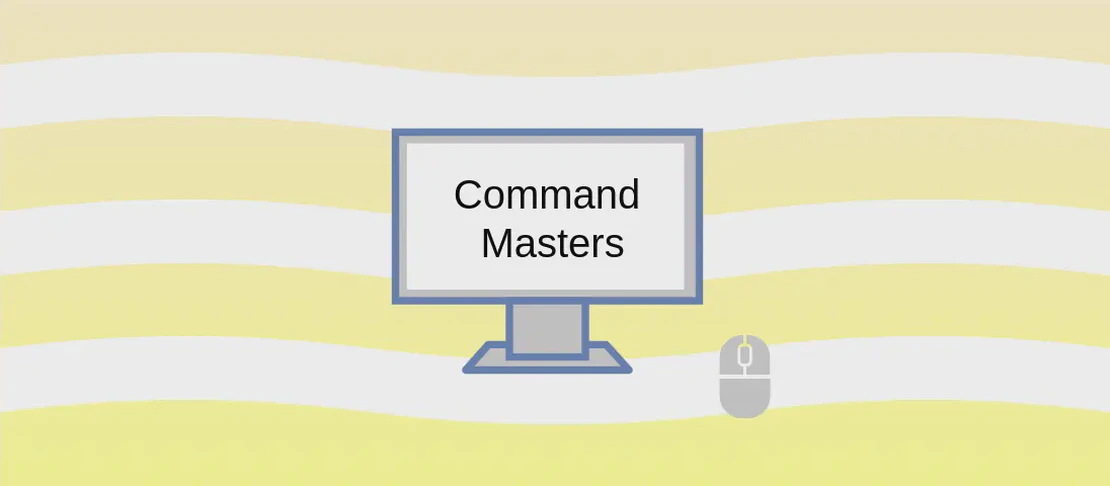
How to Use the Command 'podman-compose' (with Examples)
Podman-compose is a command-line tool used for managing containers as defined in a Compose Specification, allowing users to manage an application’s infrastructure as a group rather than individually. It’s a valuable tool for developers who prefer working with Podman instead of Docker for containerized environments. It provides an easy interface for handling multi-container Podman applications, much like Docker Compose does for Docker, facilitating various operations such as starting, stopping, and monitoring containers.
Use Case 1: Listing All Running Containers
Code:
podman-compose ps
Motivation: Sometimes, you need an overview of your application environment to determine which containers are currently running. This is particularly useful for debugging or ensuring that your full stack is up and operational.
Explanation: The command podman-compose ps
lists all of the containers that are currently running within the scope of the compose application. The ps
is short for “process status” and is a diagnostic tool providing information about active processes.
Example Output:
Name Command State Ports
--------------------------------------------------------------------
app_web_1 /start.sh Up 0.0.0.0:5000->80/tcp
app_db_1 postgres Up 0.0.0.0:5432->5432/tcp
Use Case 2: Creating and Starting All Containers in the Background
Code:
podman-compose up -d
Motivation: Often, developers want their application environment to launch quickly and run in the background without occupying the terminal. This allows them to continue working or manage other tasks while their environment remains active.
Explanation: The up
command starts the services defined in the docker-compose.yml
file. The -d
flag, short for “detached mode,” runs all containers in the background without showing their logs, similar to running a process like a daemon.
Example Output:
Creating network app_default
Creating volume app_data
Creating app_db_1
Creating app_web_1
Use Case 3: Building and Starting All Containers
Code:
podman-compose up --build
Motivation: When updating applications, containers may require rebuilding to incorporate new changes. This ensures that any alterations to images or dependencies are acknowledged before starting the containers.
Explanation: The --build
option rebuilds the images before starting the containers. This is particularly useful when there have been changes in the codebase or in the Dockerfile, reflecting these updates in the new container image.
Example Output:
Building web
Step 1/4 : FROM python:3.6
...
Successfully built abc123
Successfully tagged app_web:latest
Creating app_db_1
Creating app_web_1
Use Case 4: Starting All Containers Using an Alternate Compose File
Code:
podman-compose -f path/to/file.yaml up
Motivation: Multiple environments like development, testing, and production might require different configurations. This command is essential when you want to use specific Compose files tailored for each environment.
Explanation: The -f
or --file
flag specifies a different YAML file for podman-compose to read instead of the default docker-compose.yml
. This adaptability allows you to switch between configurations seamlessly.
Example Output:
Creating network app_dev
Creating app_db_1
Creating app_web_1
Use Case 5: Stopping All Running Containers
Code:
podman-compose stop
Motivation: When conserving resources or preparing for maintenance or modifications, stopping all containers becomes crucial. This allows the developer to pause the application’s execution without halting the entire environment.
Explanation: The command stop
halts the running containers associated with the current compose file, without removing them, allowing for quick reactivation.
Example Output:
Stopping app_web_1 ... done
Stopping app_db_1 ... done
Use Case 6: Removing All Containers, Networks, and Volumes
Code:
podman-compose down --volumes
Motivation: To clear all residual data, networks, and containers, especially before a fresh deployment or when a full clean-up is required. This command ensures no leftovers affect new environments.
Explanation: down
stops and removes containers, networks, and optionally, volumes. The flag --volumes
ensures that any volumes created by the compose file are deleted, clearing all persisted data.
Example Output:
Removing network app_default
Removing volume app_data
Removing app_web_1
Removing app_db_1
Use Case 7: Following Logs for a Container
Code:
podman-compose logs --follow container_name
Motivation: Monitoring application logs in real-time is essential for debugging and understanding runtime behavior, detecting errors, and confirming successful execution of tasks.
Explanation: The logs
command retrieves logs for one or more containers, and the --follow
flag allows you to stream new log output in real time as it appears, providing continuous feedback on container activity.
Example Output:
Attaching to app_web_1
web_1 | Starting up server on port 3000
web_1 | Request received: /index.html
Use Case 8: Running a One-Time Command in a Service
Code:
podman-compose run service_name command
Motivation: Developers frequently need to perform operations on containers, such as running database migrations or administrative commands without exposing services to the network.
Explanation: The run
command executes a one-time command inside a specified service. It does not start the other services defined in the configuration, focusing only on the necessary tasks within the selected service.
Example Output:
Starting app_db_1 ...
Running migration scripts ...
Migration completed successfully.
Conclusion
Podman-compose is a powerful tool that significantly eases the management of containerized applications using Podman. By automating many repetitive tasks through its command suite, podman-compose supports developers in managing various aspects of their containerized applications efficiently and effectively. Whether listing running containers, starting and stopping them, or performing maintenance tasks, podman-compose offers a versatile and adaptable solution.