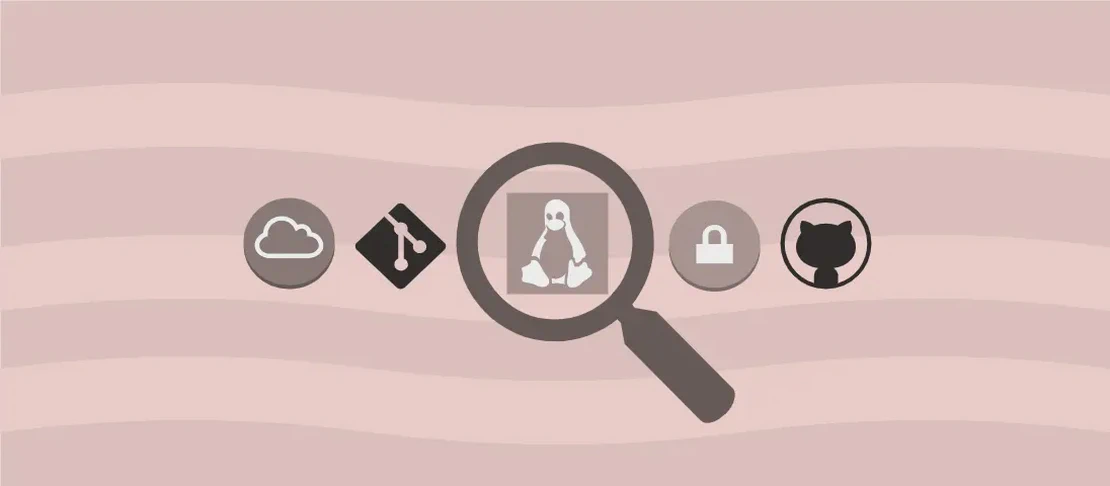
How to Use the Command 'podman run' (with Examples)
Podman, a popular tool for managing containers, offers several commands for interacting with containerized environments. One of its key commands, podman run
, allows users to create and run containers based on specified images. This command is versatile, supporting a variety of options to tailor container execution to specific needs. Below, we explore different usage scenarios for the podman run
command to demonstrate its flexibility and usefulness.
Use case 1: Run Command in a New Container from a Tagged Image
Code:
podman run image:tag command
Motivation: This simplest form of the podman run
command allows you to execute a command in a new container based on a specific image and its tag. Tags are crucial as they specify the version or variant of an image, ensuring that the desired environment or version is used.
Explanation:
podman run
: Initiates the Podman command to create and execute a new container.image:tag
: Specifies the container image and its tag from which the container will be created.command
: The command you wish to run inside the container.
Example Output: If the image and tag exist, Podman will create a container and execute the command inside it, displaying any output from the command on the terminal.
Use case 2: Run Command in a New Container in Background and Display Its ID
Code:
podman run --detach image:tag command
Motivation: Running a container in the background is useful for long-running processes or applications that do not require constant interaction, such as web services or background batch jobs.
Explanation:
podman run
: Starts a new container.--detach
: Runs the container in the background, allowing for terminal use while the container operates.image:tag
: Indicates the image and tag to use for the container.command
: The specific command or application to run in the container.
Example Output: Podman outputs the container ID, which can be used for tracking or managing the running container instance.
Use case 3: Run Command in a One-off Container in Interactive Mode and Pseudo-TTY
Code:
podman run --rm --interactive --tty image:tag command
Motivation: Interactive mode is beneficial when you need to execute commands interactively, similar to a shell session, which is helpful for debugging or direct interaction with a container environment.
Explanation:
podman run
: Launches a new container.--rm
: Automatically removes the container after it stops, ensuring no residual data remains.--interactive
: Keeps the standard input open for interaction.--tty
: Allocates a pseudo-TTY, providing a terminal interface.image:tag
: Specifies the image to create the container from.command
: The command executed interactively within the container.
Example Output: Opens an interactive session, showing command results in real-time and closing once the session ends.
Use case 4: Run Command in a New Container with Passed Environment Variables
Code:
podman run --env 'variable=value' --env variable image:tag command
Motivation: Passing environment variables is necessary for configuring applications within containers, such as defining runtime configurations or sensitive data like API keys.
Explanation:
podman run
: Begins a new container.--env 'variable=value'
: Sets environment variables within the container, allowing multiple key-value pairs.image:tag
: Selects the container’s base image.command
: Runs the command using the specified environment variables.
Example Output: Upon execution, the command outputs results influenced by the environment variables, speficially configured for the container’s operations.
Use case 5: Run Command in a New Container with Bind Mounted Volumes
Code:
podman run --volume /path/to/host_path:/path/to/container_path image:tag command
Motivation: Binding host directories with containers is essential for persistent storage, sharing files between the host and container, or using host resources like prebuilt datasets or configurations.
Explanation:
podman run
: Initiates the command to create a container.--volume /path/to/host_path:/path/to/container_path
: Mounts a volume from the host to the container, linking paths.image:tag
: Provides the image details for container creation.command
: The command operating with access to the host’s volume.
Example Output: The command interacts with the host file system, utilizing shared resources and displaying outputs accordingly.
Use case 6: Run Command in a New Container with Published Ports
Code:
podman run --publish host_port:container_port image:tag command
Motivation: Publishing ports ensures that services within the container are accessible externally, crucial for applications like web servers and databases that need external connections.
Explanation:
podman run
: Begins the creation process of a container.--publish host_port:container_port
: Maps a container’s port to an external host port, facilitating communication.image:tag
: Identifies the image used for the container.command
: The command that will run, offering services via the mapped ports.
Example Output: Displays service start status and allows access through the specified host port.
Use case 7: Run Command in a New Container Overwriting the Entrypoint of the Image
Code:
podman run --entrypoint command image:tag
Motivation: Overwriting the default entrypoint is useful for customized functionality or debugging to ensure that different processes or commands are run at container startup.
Explanation:
podman run
: Initiates a new container.--entrypoint command
: Replaces the default entrypoint command in the image with the specified one.image:tag
: Details of the image to be used.command
: Specify the new entrypoint to run in place of the image’s default command.
Example Output: Executes the provided command as the main entrypoint, showcasing its output on the terminal.
Use case 8: Run Command in a New Container Connecting It to a Network
Code:
podman run --network network image:tag command
Motivation: Connecting containers to specific networks is necessary for communication between multiple containers or for integration into existing network setups, supporting complex applications and ecosystems.
Explanation:
podman run
: Creates and runs a new container.--network network
: Connects the container to a specified network, facilitating network-specific configurations.image:tag
: Describes the image to construct the container.command
: Executes the command within the environment linked to the network.
Example Output: Runs the command with network connectivity, displaying network-related interactions or responses.
Conclusion:
The podman run
command is a powerful and robust tool for containerizing applications in a tailored manner. Its wide range of configurations ensures that containers can be run interactively or as persistent services, with necessary access to host resources, networks, and runtime environments. Each use case demonstrates the adaptability of podman run
, making it an ideal choice for diverse container management needs.