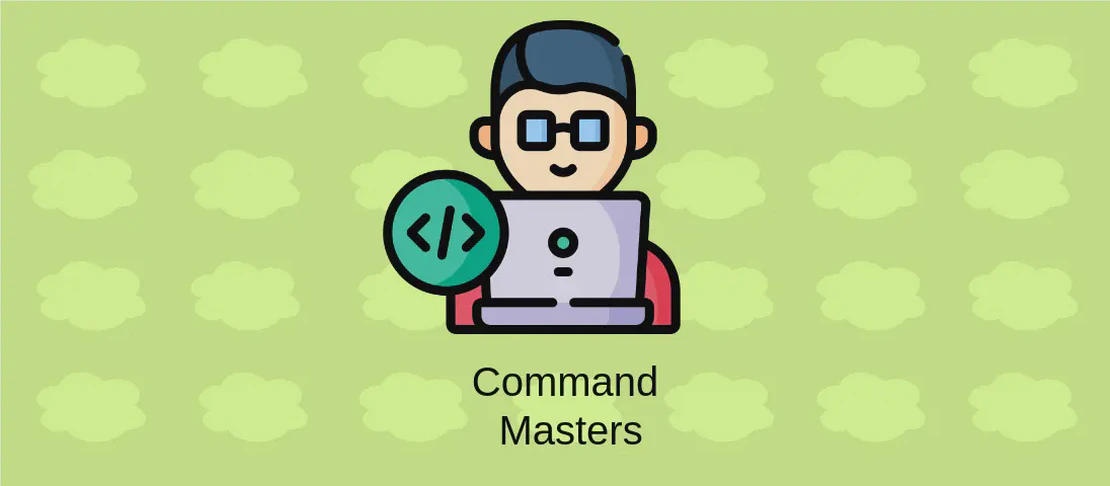
Mastering Poetry for Python Dependency Management (with examples)
Poetry is a vital tool for Python developers seeking a modern and streamlined approach to managing packages and dependencies. It simplifies the process of creating new projects, handling dependencies, and maintaining virtual environments, ensuring consistency and reducing the common pitfalls associated with Python development. Poetry is invaluable for maintaining efficient workflows in both individual and collaborative projects.
Create a new Poetry project in the directory with a specific name
Code:
poetry new project_name
Motivation:
Starting a new Python project often requires setting up a directory structure and initializing project-related files. The poetry new project_name
command automates this process, allowing developers to quickly get started with a new project without manually creating the directory structure and configuration files.
Explanation:
poetry
: The command-line interface for the Poetry tool.new
: A Poetry command to create a new project with a basic directory structure.project_name
: The desired name for the new project directory. This will be the root directory for your new project and will hold basic Python project files likepyproject.toml
and directories for your package code and tests.
Example Output:
Created package project_name in project_name
This output confirms that a new project with the name project_name
was successfully created, complete with the necessary initial files and structure.
Install and add a dependency and its sub-dependencies to the pyproject.toml
file in the current directory
Code:
poetry add dependency
Motivation:
When building Python applications, incorporating third-party libraries can significantly accelerate development by offering pre-built solutions to common challenges. The poetry add dependency
command integrates new dependencies into your project seamlessly, updating your pyproject.toml
configuration file and installing the library along with any required sub-dependencies.
Explanation:
poetry
: Calls the Poetry command-line interface.add
: A command instructing Poetry to add a new package to the project.dependency
: The name of the library or package you wish to include in your project.
Example Output:
Updating dependencies
Resolving dependencies...
Writing lock file
Package operations: 1 install, 0 updates, 0 removals
• Installing dependency (version)
This output shows that the specified dependency was successfully added, including information on any resolved version that was installed.
Install the project dependencies using the pyproject.toml
file in the current directory
Code:
poetry install
Motivation:
poetry install
Explanation:
poetry
: Engages the Poetry interface.install
: Directs Poetry to read from thepyproject.toml
file and install the necessary dependencies listed.
Example Output:
Installing dependencies from lock file
Package operations: X installs, Y updates, Z removals
• Installing example-package (version)
This output indicates the number of packages installed, updated, or removed, reflecting changes in the project’s dependencies.
Interactively initialize the current directory as a new Poetry project
Code:
poetry init
Motivation:
Initializing a new project can often be daunting, especially for beginners. The poetry init
command eases this by interactively guiding users through setting up a new project, allowing customization of project metadata like name, version, and dependencies before populating the pyproject.toml
file.
Explanation:
poetry
: Initiates the Poetry command-line interface.init
: Begins an interactive session to configure and establish a new Poetry project within the existing directory.
Example Output:
This command will guide you through creating your pyproject.toml file.
Package name [current-directory]:
Version [0.1.0]:
Description []:
Author [your-name <your-email>]:
License []:
Compatible Python versions [^3.8]:
Would you like to define your dependencies (require) interactively? (yes/no) [yes]:
The various prompts will request specific information to construct the pyproject.toml
file tailored to the user’s requirements.
Get the latest version of all dependencies and update poetry.lock
Code:
poetry update
Motivation:
Keeping project dependencies up to date can enhance security, introduce new features, and fix bugs. The poetry update
command is essential for maintaining the most recent versions of all dependencies, ensuring the project remains robust and efficient.
Explanation:
poetry
: Activates Poetry’s toolset.update
: Signals Poetry to scan for newer versions of dependencies declared withinpyproject.toml
and modifiespoetry.lock
to reflect these updates.
Example Output:
Updating dependencies
Resolving dependencies...
Writing lock file
Package operations: X installs, Y updates, Z removals
• Updating example-package (old-version -> new-version)
The output provides insight into which packages were upgraded, including the previous and new versions.
Execute a command inside the project’s virtual environment
Code:
poetry run command
Motivation:
Virtual environments are crucial for encapsulating project dependencies and avoiding conflicts with global Python packages. The poetry run command
is a flexible method to execute any command, ranging from scripts to Python interpreters, within the project’s isolated environment.
Explanation:
poetry
: Interfaces with Poetry.run
: Dictates Poetry to execute commands within the isolated environment.command
: Refers to any script or executable that you wish to run. It ensures the command utilizes the dependencies and settings from the project’s virtual environment.
Example Output:
> poetry run python script.py
Running script.py within the virtual environment.
This message confirms that the specified command was executed using the virtual environment’s dependencies and configurations.
Bump the version of the project in pyproject.toml
Code:
poetry version patch|minor|major|prepatch|preminor|premajor|prerelease
Motivation:
Version management is fundamental in software development, enabling salient tracking of changes, features, and error rectifications. The poetry version
command simplifies this process by offering straightforward version incrementation following semantic versioning protocols.
Explanation:
poetry
: Engages with the Poetry interface.version
: Indicates an update to the project’s versioning.patch|minor|major|prepatch|preminor|premajor|prerelease
: Specifies the type of version bump to perform:patch
: For bug fixes, increment the last number.minor
: For backward-compatible features, increment the middle number.major
: For incompatible changes, increment the first number.prepatch
,preminor
,premajor
,prerelease
: Applies pre-release increments.
Example Output:
Bumping version from 0.1.0 to 0.1.1
This output reflects the successful increment of the project version, ensuring continuity in version tracking.
Spawn a shell within the project’s virtual environment
Code:
poetry shell
Motivation:
A virtual environment-specific shell session allows developers to operate within the confines of the project’s dependencies and Python interpreter, ensuring all executed commands leverage the local environment setup. This is particularly useful for testing and debugging.
Explanation:
poetry
: Calls upon Poetry’s suite.shell
: Starts a new shell session that uses the virtual environment tied to the project directory.
Example Output:
Spawning shell within the virtual environment...
Virtual environment activated.
This confirmation that a shell session is running gives users the assurance that all commands executed henceforth will apply within the virtual environment context.
Conclusion:
Poetry is an indispensable tool for Python developers seeking efficiency and reliability in managing dependencies and project environments. Understanding and utilizing each of its command options enhances productivity and ensures that projects maintain optimal operation throughout their development lifecycle.