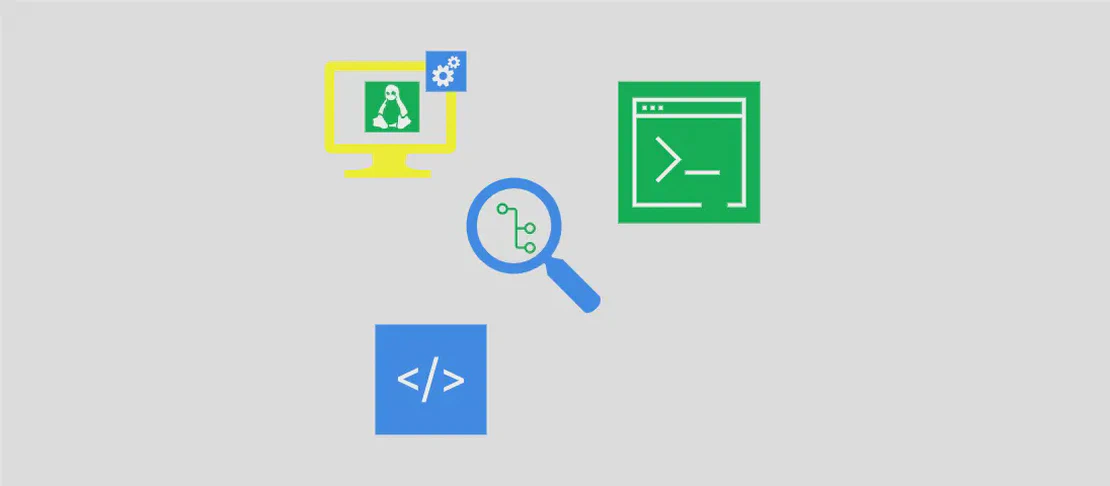
A Guide to Using the 'pre-commit' Command (with examples)
Pre-commit is a framework for managing and maintaining multi-language pre-commit hooks. These hooks are scripts that run automatically at certain points in a Git workflow, most commonly before a commit is made. By using pre-commit, developers can ensure that code undergoes necessary checks (like linting, formatting, or testing) before it gets committed to a repository. This helps maintain code quality and consistency across teams and projects.
Use case 1: Install pre-commit into your Git hooks
Code:
pre-commit install
Motivation:
The primary motivation for using this command is to integrate pre-commit hooks into your Git workflow. By running this command, you ensure that every time a git commit
is attempted, the hooks defined in your pre-commit configuration file (typically .pre-commit-config.yaml
) are executed. This automatic checking helps in catching errors and enforcing standards before the code is committed. This not only saves time spent on reviewing and debugging but also helps maintain a certain level of code quality within the team.
Explanation:
pre-commit
: This is the command-line tool that interfaces with the pre-commit package to manage hooks.install
: This argument tells the pre-commit tool to install the configured hooks into your Git repository. It essentially sets up the hooks so they will run before every commit.
Example Output:
pre-commit installed at .git/hooks/pre-commit
Here, the installation is reported as successful, indicating that any subsequent git commit
will trigger the pre-configured hooks.
Use case 2: Run pre-commit hooks on all staged files
Code:
pre-commit run
Motivation: You might need to ensure that only the files you are about to commit are subject to pre-commit checks. This command is particularly useful when your focus is solely on verifying the changes that are being prepared for commit. It helps in faster feedback because it runs hooks only on the staged changes and not the entire codebase. This will ensure that the specific changes conform to your project’s coding standards and guidelines.
Explanation:
pre-commit
: Initiates the pre-commit tool to handle the command.run
: This command is used to execute the hooks defined in the configuration. By default, it runs on the files that are staged for commit.
Example Output:
[INFO] Installing environment for https://github.com/psf/black.
[INFO] Once installed this environment will be reused.
[INFO] This may take a few minutes...
black....................................................................Passed
flake8...................................................................Passed
This illustrates a successfully passed set of hooks for staged files, with tools like black
(a code formatter) and flake8
(a linting tool) having run without issues.
Use case 3: Run pre-commit hooks on all files, staged or unstaged
Code:
pre-commit run --all-files
Motivation: Sometimes, you need reassurance that an entire codebase is in compliance with your project’s standards, not just the parts that are currently staged for commit. This is particularly useful after making large-scale changes or merging branches. Running pre-commit hooks on all the files allows you to verify the integrity and quality of the whole codebase, ensuring that no piece of code is left unchecked.
Explanation:
pre-commit
: Invokes the tool for managing Git pre-commit hooks.run
: Executes the specified hooks for code evaluation.--all-files
: This flag modifies the command to run hooks on every file in the repository, regardless of their staging status. It’s a comprehensive check beyond just the staged alterations.
Example Output:
black....................................................................Failed
- hook id: black
- exit code: 1
Files were modified by this hook. Additional output:
reformatted test.py
In this case, the output indicates that black
has reformatted test.py
to meet coding standards, reflecting the need for a check across the entire codebase.
Use case 4: Clean pre-commit cache
Code:
pre-commit clean
Motivation: As pre-commit hooks are used, various environments are set up and cached to improve performance when running hooks multiple times. Over time, these environments might require cleaning to reclaim space or fix potential corruption issues. Using this command clears all cached environments, making room for new, fresh environments to be set up during the next run. This can be essential for resolving issues stemming from outdated or corrupted cache.
Explanation:
pre-commit
: References the command-line tool associated with managing Git hooks.clean
: This command clears the cache of environments that pre-commit has set up. Removing these cached environments can help resolve conflicts and outdated configurations.
Example Output:
Cleaned 5 cached repositories in /Users/username/.cache/pre-commit/
This output confirms the action was successful, detailing the number of repositories whose cached environments were cleared.
Conclusion:
Pre-commit is a powerful tool designed to improve code quality by automating the execution of various checks through Git hooks before commits are made. Through methods like installing hook configurations, running hooks on specific portions or entire codebases, and cleaning the cache, developers can significantly streamline their workflow. With the examples above, users can clearly understand how to utilize the pre-commit
command effectively to maintain high coding standards and better manage their collaborative coding environments.