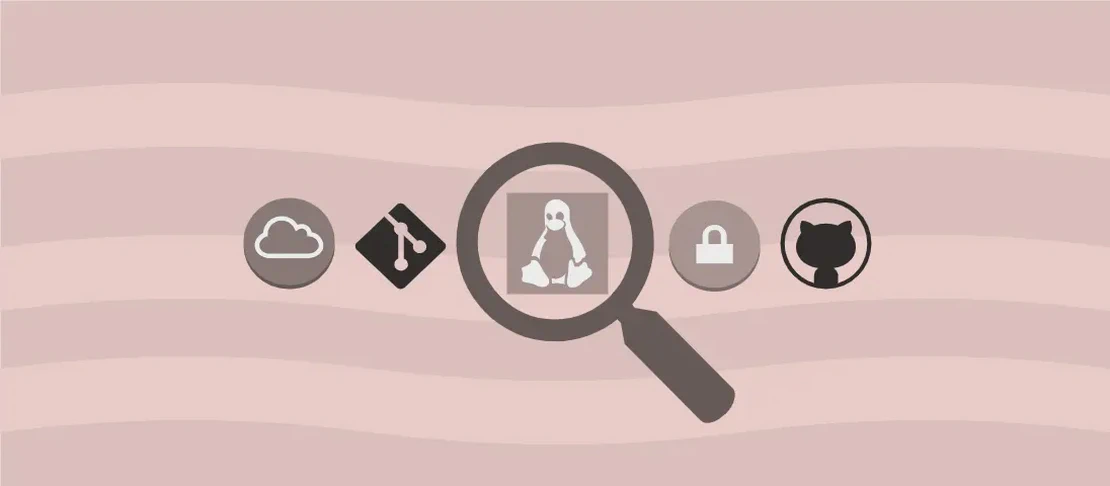
Mastering the Rename Command (with Examples)
- Linux
- December 17, 2024
The rename
command, specifically from the prename
Fedora package, offers a powerful way to manipulate file names in bulk using Perl expressions. It provides flexibility and efficiency, enabling users to perform advanced file renaming tasks quickly. Below, we explore several practical applications of the rename
command, illustrating its versatility and utility.
Use case 1: Rename Multiple Files by Substituting Text
Code:
rename 's/foo/bar/' *
Motivation:
This use case is invaluable when you need to standardize file names or replace portions of filenames across numerous files. For instance, if you have a set of files named foo_document.txt
, foo_image.jpg
, and foo_archive.zip
, and you need to replace the prefix ‘foo’ with ‘bar’, this command simplifies the task significantly, saving time and reducing errors compared to manual renaming.
Explanation:
s/foo/bar/
: This is a Perl Common Regular Expression. Thes
indicates a substitution, where ‘foo’ is replaced by ‘bar’.*
: The asterisk is a wildcard that selects all files in the current directory.
Example Output:
Before: foo_document.txt
, foo_image.jpg
, foo_archive.zip
After: bar_document.txt
, bar_image.jpg
, bar_archive.zip
Use case 2: Perform a Dry Run to Preview Changes
Code:
rename -n 's/foo/bar/' *
Motivation:
Conducting a dry run is crucial when changes to a large number of files are involved. It prevents unintended modifications by allowing you to verify the proposed changes beforehand. This is particularly useful in production environments where mistakes could lead to data loss.
Explanation:
-n
: This flag indicates a dry run, displaying the changes that would occur without actually executing them.s/foo/bar/
: The substitution pattern remains the same, changing ‘foo’ to ‘bar’.*
: The wildcard targets all files in the directory.
Example Output:
This wouldn’t change any files but output what changes would occur if the command were executed without -n
.
Preview: foo_document.txt -> bar_document.txt
, foo_image.jpg -> bar_image.jpg
Use case 3: Force Rename Even if Destination Exists
Code:
rename -f 's/foo/bar/' *
Motivation:
Sometimes, file renaming operations encounter conflicts with existing filenames at the destination. By forcing the rename, this command enables overwriting without manual intervention, streamlining workflows where high volumes of files necessitate expedited processing.
Explanation:
-f
: The force flag allows overwriting of pre-existing files with the new names.s/foo/bar/
: The standard substitution expression.*
: Wildcard for all files.
Example Output:
If bar_document.txt
already exists when renaming foo_document.txt
, this command will overwrite it.
Use case 4: Convert Filenames to Lower Case
Code:
rename 'y/A-Z/a-z/' *
Motivation:
File systems often require consistent case conventions, and converting filenames to lowercase can prevent errors and ensure uniformity. This is especially important in environments where case sensitivity can lead to broken links or inaccessible data.
Explanation:
y/A-Z/a-z/
: Translates all uppercase letters (A-Z) to lowercase (a-z).*
: Targets all files.
Example Output:
Before: Document.TXT
, Image.JPG
, Archive.ZIP
After: document.txt
, image.jpg
, archive.zip
Use case 5: Replace Whitespace with Underscores
Code:
rename 's/\s+/_/g' *
Motivation:
Whitespace in filenames can cause issues in scripts and when transferring files between systems. Replacing whitespace with underscores improves compatibility and readability, making filenames more manageable.
Explanation:
s/\s+/_/g
: Substitutes one or more whitespace characters (\s+
) with an underscore (_
). Theg
at the end ensures all instances are replaced.*
: Wildcard for all files.
Example Output:
Before: My File.txt
, Holiday Photos.jpg
After: My_File.txt
, Holiday_Photos.jpg
Conclusion:
The rename
command from the prename
Fedora package offers significant versatility and control over file naming tasks, covering a variety of use cases that meet the diverse needs of users. By leveraging regular expressions, users can confidently perform complex file renaming operations, enhancing productivity and ensuring consistency across their systems.