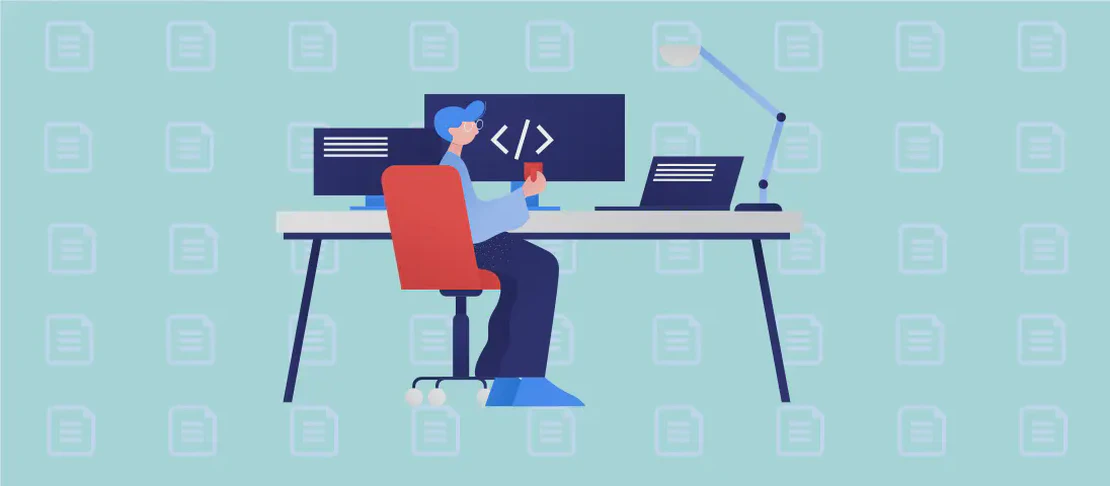
How to Use the Command 'prettier' (with examples)
Prettier is an opinionated code formatter designed to enforce a consistent coding style across many programming languages, including JavaScript, JSON, CSS, and YAML. By parsing code, Prettier reprints it with a standardized style, which ensures that teams collaborate more effectively and that code is more readable and easier to manage.
Use case 1: Format a file and print the result to stdout
Code:
prettier path/to/file
Motivation:
Using this command allows developers to see the formatted output of a file directly in the terminal. This can be particularly useful for quick checks or when you don’t want to modify the file immediately but still need to preview what the formatted output would look like.
Explanation:
prettier
: This is the command that triggers Prettier to format your code.path/to/file
: This argument specifies the path to the file that you wish to format. Prettier will read this file, format it according to its rules, and print the output tostdout
.
Example Output:
Suppose the input file contains the JavaScript code function foo(){console.log('Hello world');}
, then the output shown in the terminal might be:
function foo() {
console.log("Hello world");
}
Use case 2: Check if a specific file has been formatted
Code:
prettier --check path/to/file
Motivation:
This command is beneficial when you need to verify if a file is already formatted according to Prettier’s rules. It’s often used in CI/CD pipelines to enforce code style guidelines before code is merged or deployed.
Explanation:
prettier
: Launches the Prettier tool to be run on the specified file.--check
: This option instructs Prettier to check whether the file is already formatted. It will return an appropriate exit code—0 if the file is correctly formatted, 1 if not.path/to/file
: The path to the file you wish Prettier to check.
Example Output:
If the file is already formatted, there will be no output, and the exit code will be 0. If not, the output might resemble:
path/to/file
Code style issues found in the above file(s). Forgot to run Prettier?
Use case 3: Run with a specific configuration file
Code:
prettier --config path/to/config_file path/to/file
Motivation:
This is useful when you have specific formatting requirements that differ from the default Prettier settings. By using a custom configuration file, you can enforce specific rules and settings across multiple projects or to cater to individual project requirements.
Explanation:
prettier
: The command to initiate the Prettier formatting process.--config
: Specifies that a custom configuration file should be used for formatting settings instead of the default.path/to/config_file
: Path to the custom configuration file used for formatting options.path/to/file
: The file to be formatted using the custom configurations.
Example Output:
Using a custom configuration might adjust output style specifics, such as line lengths and indentation. Without direct programmatic effects like stdout
, the impact would primarily be seen in the structure and presentation marked by the settings defined in the configuration file.
Use case 4: Format a file or directory, replacing the original
Code:
prettier --write path/to/file_or_directory
Motivation:
This command is essential for ensuring that all code adheres to the formatting rules enforced by Prettier across a codebase. By writing back to the file or files, teams can maintain consistent style and avoid manual formatting.
Explanation:
prettier
: Begins the process of code formatting.--write
: This instructs Prettier to replace the content of the original file(s) with the formatted content. This modifies the file directly, reflecting the influence of Prettier’s rules.path/to/file_or_directory
: Specifies the file or entire directory to be formatted.
Example Output:
After running this command, the files will be silently modified in place, with their formatting updated according to Prettier rules. For example, messy or non-standard spacing will be corrected, improving code readability substantially.
Use case 5: Format files or directories recursively using single quotes and no trailing commas
Code:
prettier --single-quote --trailing-comma none --write path/to/file_or_directory
Motivation:
For projects with style guides that prefer single quotes and no trailing commas, this command variant allows adherence to those specific conventions. Teams who opt for particular styles due to language features or personal preference will find this very useful.
Explanation:
prettier
: Invokes the Prettier formatting tool for the task.--single-quote
: Determines that Prettier should use single quotes instead of double quotes in strings.--trailing-comma none
: Specifies that no trailing commas should be added in objects or arrays.--write
: Indicates that the files should be modified directly.path/to/file_or_directory
: Designates the path where formatting should be applied.
Example Output:
While no direct output is seen, formatted files will reflect choices such as:
const example = {
a: 'Alpha',
b: 'Beta'
}
Instead of using double quotes or having trailing commas, maintaining the style consistency preferred in some JavaScript projects.
Use case 6: Format JavaScript and TypeScript files recursively, replacing the original
Code:
prettier --write "**/*.{js,jsx,ts,tsx}"
Motivation:
In large codebases with multiple JavaScript and TypeScript files, this command allows for efficient and widespread application of Prettier formatting rules across the entire project. Consistency in code style is thus enforced at scale, saving time and reducing manual overhead.
Explanation:
prettier
: Executes the formatting process.--write
: Directs Prettier to make changes directly on files."**/*.{js,jsx,ts,tsx}"
: Uses a glob pattern to target all.js
,.jsx
,.ts
, and.tsx
files recursively within a directory and its subdirectories.
Example Output:
Again, there’s no direct stdout
output; however, all the targeted files will be modified to adhere to Prettier’s formatting. The advantage is consistency across diverse file types in the same project.
Conclusion:
Prettier is an invaluable tool for developers seeking consistent code formatting across their entire codebase. By automating the process of applying a standardized style, it facilitates better collaboration, enhances readability, and significantly reduces the scope for purely stylistic disagreements in code reviews. These examples demonstrate varying use cases, each tailored to specific needs, from checking formatting to applying customized settings, all the while emphasizing uniformity and maintainability.