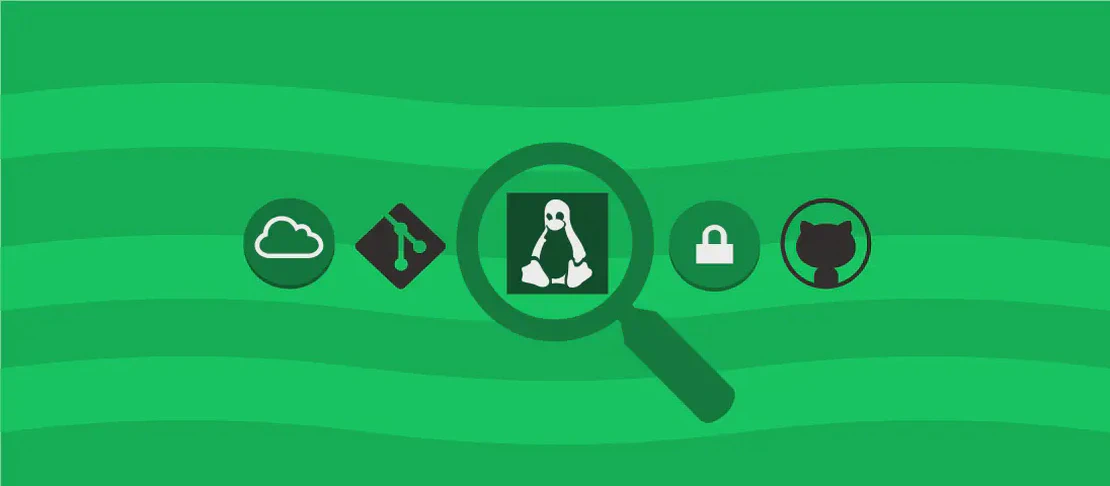
How to utilize the `printf` command (with examples)
The printf
command is a powerful tool derived from the C programming language adapted into the Unix shell environment. It enables users to print text in a formatted fashion, offering more control and precision than the standard echo
command. With printf
, you can format strings, numbers, and even incorporate special characters or text formats. It’s particularly useful in scripting where precise output manipulation is necessary. Below, we illustrate several use cases of the printf
command to exhibit its versatility and functionality.
Use case 1: Print a text message
Code:
printf "%s\n" "Hello world"
Motivation:
The need to display messages to users is common across scripts and command-line operations. Printf
provides the structure needed to format these messages consistently, increasing the clarity and readability of output.
Explanation:
%s
: This is the string format specifier, indicating we want to print a string.\n
: A newline character to ensure the output continues on a new line after the given text."Hello world"
: The message to be printed.
Example output:
Hello world
Use case 2: Print an integer in bold blue
Code:
printf "\e[1;34m%.3d\e[0m\n" 42
Motivation:
In many scenarios, such as logging or highlighting important information in outputs, emphasizing specific numbers or text is valuable. The printf
command allows you to apply text properties like color and boldness, supporting better readability.
Explanation:
\e[1;34m
: This is an ANSI escape code to start setting the text style.1
signifies bold text, and34
represents blue color.%.3d
: This is the integer format specifier where.3
means the integer should be zero-padded to at least three digits.\e[0m
: ANSI escape code to reset the text formatting back to default.\n
: Ends the line for cleaner output.42
: The integer value being displayed.
Example output:
[The terminal output will show the number 042 in bold blue.]
Use case 3: Print a float number with the Unicode Euro sign
Code:
printf "\u20AC %.2f\n" 123.4
Motivation:
Displaying currency values in scripts might involve needing to attach symbols such as the Euro sign. This capability supports financial software development or any script needing monetary representations.
Explanation:
\u20AC
: Unicode representation of the Euro symbol (€).%.2f
: The float format specifier, indicating the desire to print a float with two decimal places.123.4
: The floating-point number to be formatted and displayed.
Example output:
€ 123.40
Use case 4: Print a text message composed with environment variables
Code:
printf "var1: %s\tvar2: %s\n" "$VAR1" "$VAR2"
Motivation:
Often during shell scripting, you need to print current values of various environment variables to understand the current state or debugging purposes. Printing a message that merges static text with dynamic variables is a frequent necessity.
Explanation:
var1: %s\tvar2: %s
: Template string where%s
placeholders represent where environment variable values will be injected.\t
provides a tab space for alignment."$VAR1" "$VAR2"
: The respective environment variables whose values will replace%s
placeholders.
Example output if VAR1
is “value1” and VAR2
is “value2”:
var1: value1 var2: value2
Use case 5: Store a formatted message in a variable (does not work on Zsh)
Code:
printf -v myvar "This is %s = %d\n" "a year" 2016
Motivation:
There are situations where formatted text must be stored in a variable for subsequent processing or reuse. This approach can be pivotal in dynamic scripting where output might need further manipulation before final display or use.
Explanation:
-v myvar
: Tellsprintf
to store the output in the variablemyvar
.This is %s = %d\n
: The format string where%s
is replaced by “a year” and%d
by 2016."a year"
,2016
: Arguments replace%s
and%d
respectively.
Example output (stored in myvar
):
This is a year = 2016
Use case 6: Print a hexadecimal, octal, and scientific number
Code:
printf "hex=%x octal=%o scientific=%e" 0xFF 0377 100000
Motivation:
Numerical data often needs representation in various formats like hexadecimal or octal for better insight, especially in areas like computer science or electronics. Scientific format helps in representing large or small numbers conveniently.
Explanation:
hex=%x
andoctal=%o
: Format specifiers for hexadecimal and octal output respectively.scientific=%e
: Specifies the scientific notation.0xFF
,0377
,100000
: Values to be formatted as specified.
Example output:
hex=ff octal=377 scientific=1.000000e+05
Conclusion:
The printf
command is a versatile and robust tool for formatting outputs in shell environments. Its power lies in detailed specification options that allow handling complex formatting tasks that would be cumbersome with simpler commands. Each use case above demonstrates practical scenarios where printf
enhances script functionality by making outputs more efficient, readable, and tailored to specific needs. Whether you are script writing for automation, data processing, or user interfacing, mastery of printf
commands can be a significant asset.