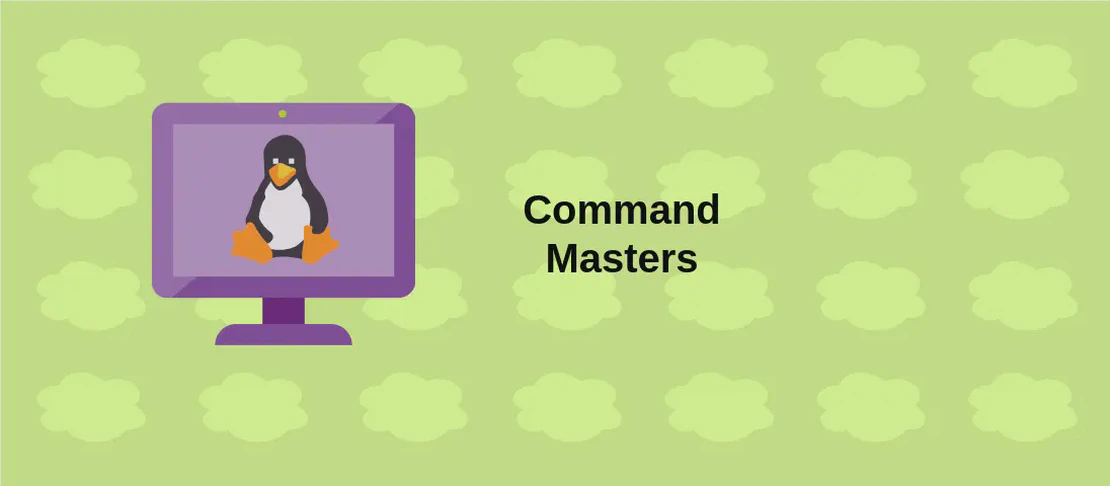
How to Use the Command 'protoc' (with Examples)
Protocol Buffers, or Protobuf, is an efficient and flexible method for serializing structured data, developed by Google. The protoc
command is a compiler for Google’s Protocol Buffers, which translates .proto
files into language-specific code that can be used to encode and decode your data. This tool is highly useful in scenarios involving cross-language communication, where you need to ensure data consistency across different systems.
Use case 1: Generate Python Code from a .proto
File
Code:
protoc --python_out=path/to/output_directory input_file.proto
Motivation:
When developing applications in Python that require communication over network or storage, Protocol Buffers can be very beneficial due to their speed and efficiency. By generating Python code from a .proto
file, developers create Python classes that handle the serialization and deserialization of the defined data structures, ensuring seamless data translation and communication between systems.
Explanation:
protoc
: This is the command-line tool that compiles.proto
files into code for various programming languages.--python_out=path/to/output_directory
: This argument specifies the output directory where the generated Python code should be placed. The--python_out
flag tells the compiler to generate Python classes from the.proto
file.input_file.proto
: This is the Protocol Buffer file that contains your message types and data structure definitions. The compiler reads this file to generate the necessary code.
Example Output:
Using this command creates a .py
file in the specified output directory that contains Python classes corresponding to the messages defined in input_file.proto
. These classes include methods for serializing the message to a binary format and parsing the binary format back into a Python object.
Use case 2: Generate Java Code from a .proto
File that Imports Other .proto
Files
Code:
protoc --java_out=path/to/output_directory --proto_path=path/to/import_search_path input_file.proto
Motivation:
In complex applications, it’s common to organize Protocol Buffer definitions across multiple .proto
files for better modularity and maintainability. When a .proto
file imports definitions from others, all imports need to be resolved during code generation. Generating Java code from such files ensures that all dependencies are correctly included, maintaining the integrity and consistency of your Java application’s data structures.
Explanation:
protoc
: Executes the Protocol Buffers compiler.--java_out=path/to/output_directory
: Indicates that the output code should be Java and specifies the directory where this code will be saved.--proto_path=path/to/import_search_path
: This sets the directory where the compiler will look for imported.proto
files. This path ensures that all imported dependencies are located and correctly integrated into the generated code.input_file.proto
: The main.proto
file being compiled, which may include import statements for other.proto
files.
Example Output:
This command will generate .java
files, which correspond to each of the message types defined in input_file.proto
and any other messages in imported files. These files will include Java classes with methods to serialize and parse messages, leveraging Protocol Buffers’ efficiency and speed.
Use case 3: Generate Code for Multiple Languages
Code:
protoc --csharp_out=path/to/c#_output_directory --js_out=path/to/js_output_directory input_file.proto
Motivation:
In today’s interconnected world, software systems often involve components written in different programming languages. By generating code for multiple languages from a single .proto
file, protoc
facilitates cross-language data exchange. This use case is particularly crucial for projects involving microservices or multi-platform applications, allowing each component to interact seamlessly despite language differences.
Explanation:
protoc
: The protobuf compiler is being executed to process.proto
files into specific language bindings.--csharp_out=path/to/c#_output_directory
: This option directs the compiler to generate C# code, placing it in the specified directory, thus enabling use in .NET environments.--js_out=path/to/js_output_directory
: Similar to the C# directive, this instructs the compiler to produce JavaScript code, saving it in a given directory for use in web applications or Node.js.input_file.proto
: The file containing the protocol buffer definitions to be compiled into both C# and JavaScript.
Example Output:
Executing this command results in C# and JavaScript files, each containing classes and methods for managing data structures defined in input_file.proto
. These generated files facilitate serialization and deserialization, ensuring the structured data can be seamlessly shared across different components of a multi-language system.
Conclusion:
The protoc
command serves as an essential tool for developers dealing with systems where communication between different platforms or languages is crucial. By generating language-specific code from .proto
files efficiently, it ensures that data can be serialized and parsed across varied programming environments, enhancing systems interoperability and performance. With examples like Python, Java, C#, and JavaScript, protoc
demonstrates its versatility and indispensability in modern software architecture.