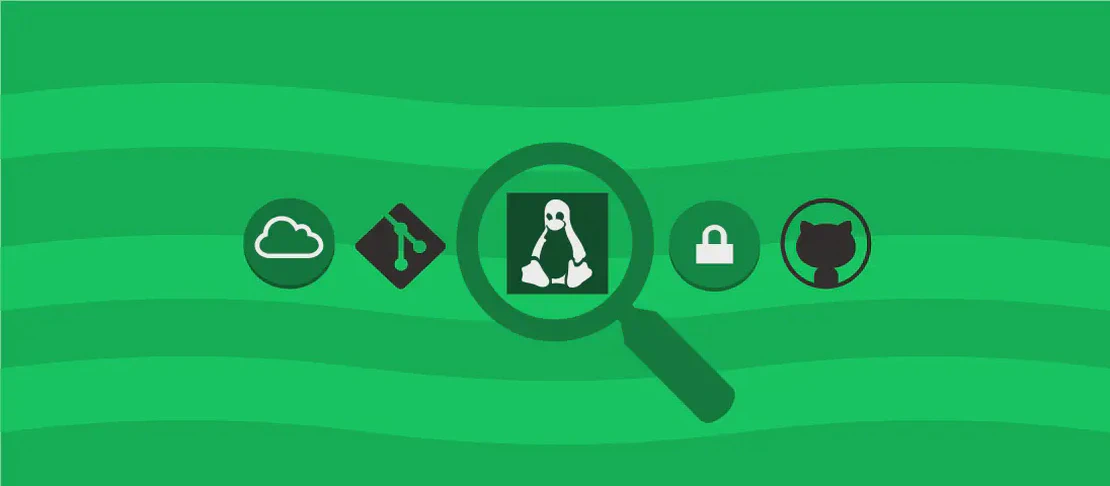
How to use the command psalm (with examples)
Psalm is a static analysis tool for finding errors in PHP applications. It helps developers identify potential issues in their code to ensure better quality and maintainability. In this article, we will explore various use cases of the psalm
command and provide examples for each case.
Use case 1: Generate a Psalm configuration
Code:
psalm --init
Motivation:
Generating a Psalm configuration is the first step before analyzing a PHP project with Psalm. This command creates an initial configuration file named psalm.xml
in the current working directory.
Explanation:
--init
: This flag instructs thepsalm
command to generate a configuration file for the project.
Example output:
Config file created successfully at /path/to/project/psalm.xml
Use case 2: Analyze the current working directory
Code:
psalm
Motivation: Analyzing the current working directory allows you to perform a static analysis on all PHP files within that directory. It helps identify any potential errors or problems in the codebase.
Explanation:
No additional arguments or flags are provided. psalm
with no arguments simply runs the analysis on the current directory.
Example output:
No errors found. Analysis complete.
Use case 3: Analyze a specific directory or file
Code:
psalm path/to/file_or_directory
Motivation: Sometimes, you may want to analyze a specific directory or file rather than the entire project. This can be helpful when you want to focus on a particular part of the codebase or if you want to analyze external libraries separately.
Explanation:
path/to/file_or_directory
: Replace this with the actual path to the file or directory you want to analyze.
Example output:
Analyzing /path/to/file...
7 errors found. Analysis complete.
Use case 4: Analyze a project with a specific configuration file
Code:
psalm --config path/to/psalm.xml
Motivation: Analyzing a project with a specific configuration file allows you to customize the analysis settings according to your project’s requirements. You can define various options and rules specific to your codebase.
Explanation:
--config path/to/psalm.xml
: This flag specifies the path to the configuration file to be used for the analysis.
Example output:
Analyzing project with custom configuration...
14 errors found. Analysis complete.
Use case 5: Include informational findings in the output
Code:
psalm --show-info
Motivation: In addition to errors, Psalm can also provide informational findings about the code. These findings highlight potential improvements or best practices that can be followed. Enabling this option helps improve code quality.
Explanation:
--show-info
: This flag indicates that informational findings should be included in the analysis output.
Example output:
Analyzing project with information findings enabled...
5 info findings found. Analysis complete.
Use case 6: Analyze a project and display statistics
Code:
psalm --stats
Motivation: When analyzing a large project, it can be beneficial to see summary statistics of the analysis. This includes the number of files analyzed, total errors found, types scanned, and more.
Explanation:
--stats
: This flag generates and displays statistics after the analysis completes.
Example output:
Analyzing project and generating statistics...
----------------- STATISTICS -----------------
Files analyzed: 103
Total errors: 22
Types scanned: 3440
----------------------------------------------
Analysis complete.
Use case 7: Analyze a project in parallel with multiple threads
Code:
psalm --threads 4
Motivation: Analyzing a project in parallel using multiple threads can significantly speed up the analysis process. By utilizing the available CPU cores, Psalm can distribute the workload and complete the analysis faster.
Explanation:
--threads 4
: This flag specifies the number of threads to be used for parallel analysis. In this example, we are using 4 threads.
Example output:
Analyzing project with 4 threads...
No errors found. Analysis complete.
Conclusion:
The psalm
command provides several powerful options to analyze PHP applications using static analysis. Whether you want to analyze the entire project or a specific part of it, generate a configuration file, include informational findings, or display statistics, Psalm has you covered. By utilizing the examples and explanations in this article, you can leverage the full potential of Psalm for better code quality and maintainability in your PHP projects.