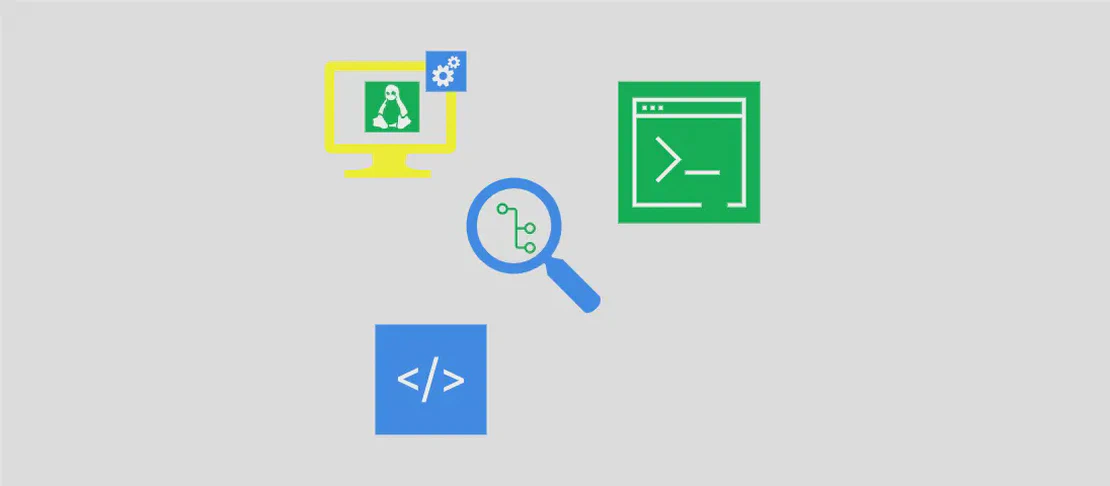
How to use the command 'pwsh' (with examples)
PowerShell, invoked using the pwsh
command, is a command-line shell and scripting language designed primarily for system administration. It provides a robust framework for automating tasks, managing systems at scale, and executing scripts across multiple platforms. The pwsh
command specifically refers to PowerShell 6 and above, often known as PowerShell Core, which is cross-platform and can be run on Windows, macOS, and Linux. This contrasts with the legacy Windows PowerShell, which is specific to Windows.
Use case 1: Start an interactive shell session
Code:
pwsh
Motivation: Starting an interactive shell session allows a user to execute commands and scripts dynamically, inspect variables, and manipulate data in real-time. This interactive mode is essential for tasks that require iterative testing and experimentation.
Explanation: The pwsh
command with no additional arguments launches an interactive shell session. This mode allows for live execution of commands where users can type in commands one at a time and receive instant feedback.
Example Output:
PS /home/user> _
Use case 2: Start an interactive shell session without loading startup configs
Code:
pwsh -NoProfile
Motivation: Sometimes, users want a clean session without any preloaded scripts or configuration settings that might alter the shell environment. Using the -NoProfile
option is crucial for diagnosing issues or running scripts in a controlled environment without interference from user profiles.
Explanation: The -NoProfile
argument tells PowerShell not to load profile scripts. This keeps your environment pure and prevents any potential conflicts from personalized settings or scripts that automatically run on startup.
Example Output:
PS /home/user> _
Use case 3: Execute specific commands
Code:
pwsh -Command "echo 'powershell is executed'"
Motivation: This use case is perfect for users who wish to execute a single or a series of PowerShell commands on the fly without entering the full shell session. It helps automate small tasks directly from the command line or scripts of another language.
Explanation: The -Command
argument allows the execution of commands as a string. In this example, the echo command outputs the phrase ‘powershell is executed’, demonstrating how simple tasks can be automated efficiently.
Example Output:
powershell is executed
Use case 4: Execute a specific script
Code:
pwsh -File path/to/script.ps1
Motivation: By executing a script file directly, users can automate repetitive tasks and complex workflows outlined in PowerShell scripts. This approach simplifies the execution process and ensures consistency and reproducibility of tasks.
Explanation: The -File
argument is used to specify a path to a PowerShell script file (.ps1
). PowerShell will run the commands stored in this script file, making it an effective way to handle pre-written automation tasks.
Example Output:
<Output contingent on script contents>
Use case 5: Start a session with a specific version of PowerShell
Code:
pwsh -Version version
Motivation: As new versions of PowerShell are released, some scripts or commands may need to be executed with specific versions to ensure compatibility. This command is essential for environments where version control is critical.
Explanation: The -Version
argument specifies that the session should be started with a particular version of PowerShell. This helps ensure that any version-specific features or syntax are supported during execution.
Example Output:
<Launches specified version or errors if unavailable>
Use case 6: Prevent a shell from exit after running startup commands
Code:
pwsh -NoExit
Motivation: Running startup commands and then staying in the shell can be useful for testing startup behavior or for automating setups followed by manual interaction.
Explanation: The -NoExit
argument keeps the PowerShell session open even after executing initial startup commands. This facilitates continued interactive use after initial setup.
Example Output:
PS /home/user> _
Use case 7: Describe the format of data sent to PowerShell
Code:
pwsh -InputFormat Text|XML
Motivation: Specifying the input format ensures the shell interprets input data correctly, especially when receiving complex data structures from other processes or applications.
Explanation: The -InputFormat
argument determines the format of the incoming data. Either Text
or XML
can be chosen depending on the data source and requirements, ensuring accurate data interpretation.
Example Output:
<Depends on whether Text or XML data is processed>
Use case 8: Determine how an output from PowerShell is formatted
Code:
pwsh -OutputFormat Text|XML
Motivation: Manipulating the output format ensures that data is presented in an understandable or required format for further processing or reporting.
Explanation: Similar to input, the -OutputFormat
argument determines whether the PowerShell commands’ output should be in plain Text
or XML
format, aiding in integration with various other systems and processes.
Example Output:
<Formatted output contingent on Script or Command>
Conclusion:
PowerShell via the pwsh
command offers diverse capabilities for executing scripts and managing systems across platforms. Understanding the various command options and arguments enhances users’ ability to automate, test, and optimize tasks effectively, making PowerShell an indispensable tool in the realm of modern IT and DevOps practices.