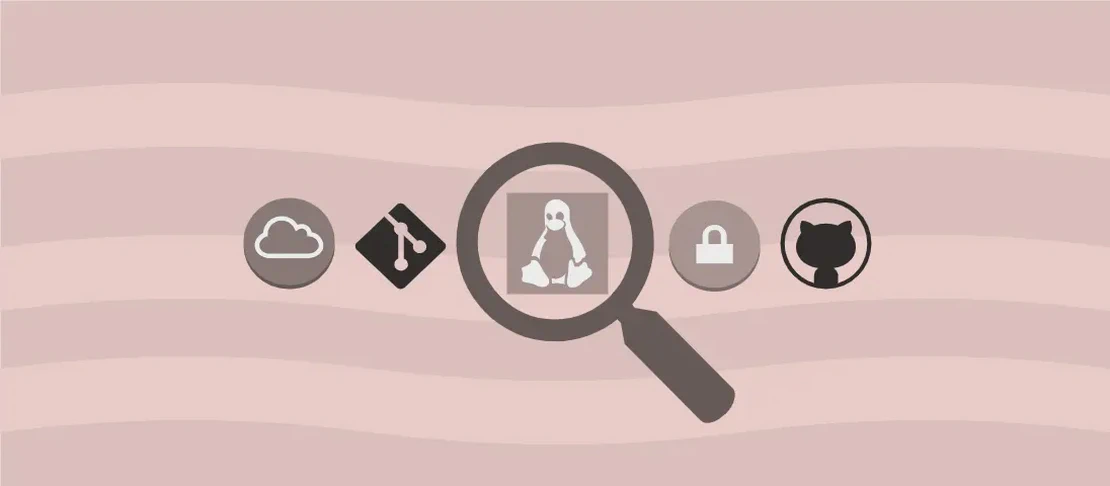
Using 'py-spy' to Profile Python Programs (with examples)
Py-spy is a powerful sampling profiler designed for Python programs. It allows developers to understand which parts of their code consume the most execution time, making it an invaluable tool for performance optimization. By providing real-time monitoring and detailed flame graphs of function execution, py-spy helps programmers pinpoint bottlenecks and optimize their applications efficiently. More information can be found on its GitHub page .
Use case 1: Show a live view of the functions that take the most execution time of a running process
Code:
py-spy top --pid pid
Motivation:
In situations where a Python application seems to be running slower than expected, it’s crucial to identify which parts of the code are responsible for the most CPU usage. This command provides a live view of the functions consuming the most execution time for a specified running process, allowing developers to diagnose problems in real-time without having to stop the ongoing process.
Explanation:
py-spy top
: Initiates a real-time view similar to the Unixtop
command but focused on Python functions.--pid pid
: Specifies the process ID of the running Python application that you wish to profile. The PID can be found using tools likeps
on Unix-based systems. This indicates to py-spy which specific running process it should observe since you may have multiple Python applications running concurrently.
Example Output:
Imagine running py-spy top --pid 1234
on a Python web service process with PID 1234. The output would dynamically update to showcase the functions consuming the most execution time at the top, typically showing the function name, the percentage of total CPU time it’s using, and other related metrics.
Use case 2: Start a program and show a live view of the functions that take the most execution time
Code:
py-spy top -- python path/to/file.py
Motivation:
When starting a Python script for the first time, or during the development phase, it’s helpful to immediately see how different parts of the code behave in terms of execution time. This use case allows developers to start a program and simultaneously view live statistics about function performance from the get-go, leading to more informed coding practices early in the development cycle.
Explanation:
py-spy top
: Just like before, this command starts a live monitoring session.--
: A double dash is used to separate py-spy’s options from the command line invoking the Python script.python path/to/file.py
: This is the command to start the execution of a specific Python script. Replacepath/to/file.py
with the path to your specific Python file.
Example Output:
Running py-spy top -- python my_script.py
would execute my_script.py
while simultaneously showing a real-time update of function execution times on the console. Functions consuming more resources will float to the top, updating dynamically as the script runs.
Use case 3: Produce an SVG flame graph of the function call execution time
Code:
py-spy record -o path/to/profile.svg --pid pid
Motivation:
Flame graphs are a visual representation of profiling data that provide a clear overview of how much CPU time each function and its children are consuming. Using this command can help developers visually identify performance bottlenecks and understand how function calls propagate through the call stack, leading to more effective optimizations.
Explanation:
py-spy record
: This command records profiling data over a period of time rather than providing an instantaneous snapshot.-o path/to/profile.svg
: Specifies the output file for the flame graph in SVG format. You should replacepath/to/profile.svg
with the desired file path and name for the output.--pid pid
: Points to the specific process ID of the application you want to profile.
Example Output:
After running the command, the generated SVG file (profile.svg
) will present a visual flame graph. The width of each box is proportional to the amount of time spent in the function, and navigating through this graph can help identify which calls are the real time sinks.
Use case 4: Dump the call stack of a running process
Code:
py-spy dump --pid pid
Motivation:
Sometimes, you may need to inspect the current state of a Python application to understand how it reached a certain point in its execution. Dumping the call stack provides a snapshot of the frames and the functions currently being called, which is critical for debugging complex applications or understanding unexpected behavior.
Explanation:
py-spy dump
: This command takes a snapshot of the current call stack for the specified process.--pid pid
: Identifies the process ID of the running Python application to examine. Knowing the exact PID ensures that you are looking at the correct application’s state.
Example Output:
Executing py-spy dump --pid 1234
would output the call stack of the process with PID 1234, showing the hierarchy of function calls from the bottom of the stack to the top, along with file names and line numbers. This output is particularly useful for developers seeking to troubleshoot issues with specific parts of their code.
Conclusion:
Py-spy is a versatile tool that offers crucial insights into the performance of Python programs. By enabling real-time monitoring, generating graphical reports, and allowing snapshot dumps of call stacks, py-spy empowers developers to optimize their applications effectively. These examples illustrate how to leverage py-spy for improving code efficiency, understanding program behavior, and ultimately providing a better end-user experience.