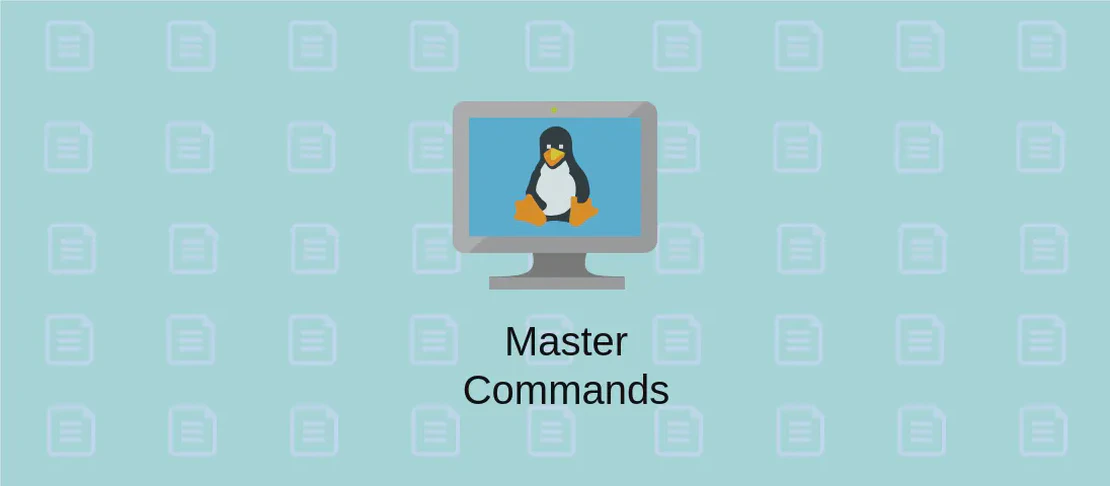
Exploring 'pycodestyle' for Python Code Style Checking (with examples)
Pycodestyle is a crucial tool for Python developers aiming to maintain clean and readable code. This command-line tool analyses Python code against PEP 8, the style guide for Python code, ensuring adherence to best practices in coding style. By using pycodestyle, programmers can automate style checks and maintain consistency in codebases, promoting collaboration and avoiding potential errors associated with style deviations.
Use case 1: Check the style of a single file
Code:
pycodestyle file.py
Motivation:
The primary motivation for checking a single file is to ensure that an individual Python script adheres to PEP 8 standards. This might be the case during development when you’re working on a standalone script or feature in isolation. Running pycodestyle on a single file can quickly highlight areas that deviate from the prescribed style, allowing you to make the necessary adjustments before integrating your code with a larger project.
Explanation:
pycodestyle
: This is the command-line tool invoked to check Python file styles.file.py
: This argument specifies the target file for the style check. Substitutefile.py
with the path of your specific Python file you wish to check.
Example output:
file.py:10:1: E302 expected 2 blank lines, found 1
file.py:15:10: E225 missing whitespace around operator
Use case 2: Check the style of multiple files
Code:
pycodestyle file1.py file2.py ...
Motivation:
When working in larger projects, consistency across multiple Python files is vital. Running pycodestyle simultaneously on several files ensures that all scripts within a project adhere to PEP 8 conventions, thereby decreasing discrepancies and enhancing cooperative coding efforts across teams. This bulk check is particularly useful during code reviews or prior to code merges.
Explanation:
pycodestyle
: Initiates the style check.file1.py file2.py ...
: Specifies multiple files for analysis. Replace these placeholders with the actual file names you intend to check.
Example output:
file1.py:5:2: E111 indentation is not a multiple of four
file2.py:22:20: W291 trailing whitespace
Use case 3: Show only the first occurrence of an error
Code:
pycodestyle --first file.py
Motivation:
In certain situations, especially when dealing with a file that has numerous style errors, output can become overwhelming. By focusing on just the first occurrence of each type of error, pycodestyle helps prioritize the most pressing issues first. This approach simplifies the process of incremental improvement, making it manageable to fix specific issues one at a time.
Explanation:
pycodestyle
: The tool used for style checking.--first
: This flag limits the display to only the first instance of each unique error, preventing redundant output for similar mistakes.file.py
: The specific file you are analyzing.
Example output:
file.py:5:2: E111 indentation is not a multiple of four
file.py:3:19: E231 missing whitespace after ','
Use case 4: Show the source code for each error
Code:
pycodestyle --show-source file.py
Motivation:
Visualizing the source code associated with style errors can provide immediate context, enabling faster and more efficient troubleshooting. For learners and developers unfamiliar with specific PEP 8 guidelines, it’s particularly helpful to see the offending code highlighted alongside its corresponding error message.
Explanation:
pycodestyle
: Executes the style analysis.--show-source
: This option displays the offending line of code for each error detected, making it easier to identify and understand the issue at a glance.file.py
: The input file being validated.
Example output:
file.py:10:1: E302 expected 2 blank lines, found 1
def example_function():
^
Use case 5: Show the specific PEP 8 text for each error
Code:
pycodestyle --show-pep8 file.py
Motivation:
Understanding the reasoning behind each PEP 8 rule is invaluable, especially for educational purposes or when debating style standards within a team. By displaying the specific PEP 8 guideline text for each error, developers can better appreciate the rationale behind each “violation”, forming a deeper understanding of Python’s stylistic choices and improving their coding discipline.
Explanation:
pycodestyle
: The command initiated for analysis.--show-pep8
: An option that presents the relevant passage from the PEP 8 document related to each detected error, providing a clear explanation of why an element is flagged.file.py
: The Python script subjected to the style check.
Example output:
file.py:15:10: E225 missing whitespace around operator
Section: 'Whitespace in Expressions and Statements'
Write: x = x + 1 instead of x=x+1. Add a single space on both sides of the binary operator.
Conclusion:
Pycodestyle serves as a robust tool to promote high-quality, standardized Python code. By employing its versatile command options, developers can systematically address style issues, understand PEP 8 guidelines deeply, and foster cohesive collaboration within teams, thus facilitating more readable and maintainable codebases.