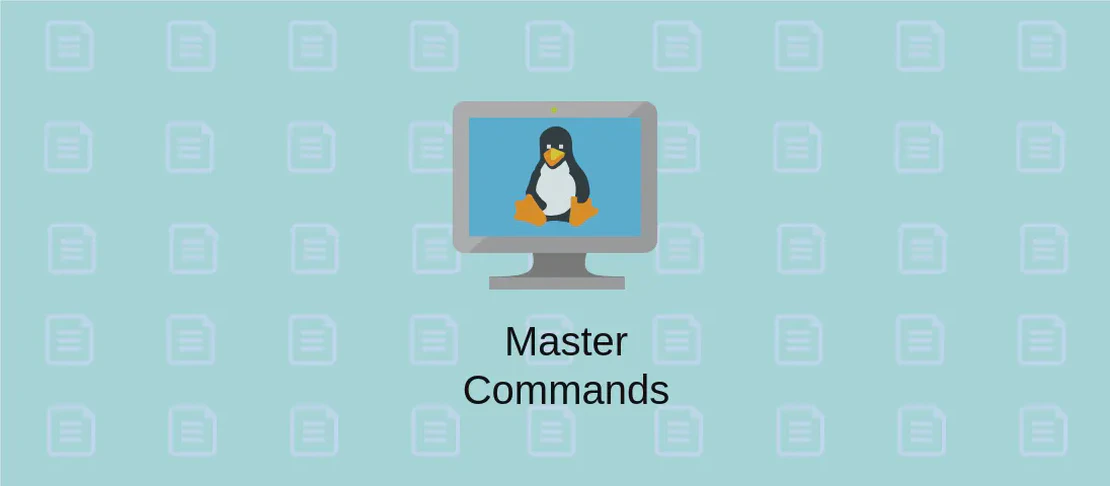
How to use the command 'pyenv virtualenv' (with examples)
The ‘pyenv virtualenv’ command is used to create and manage virtual environments based on the installed Python distributions. It allows users to easily switch between different Python versions and sets of installed packages.
Use case 1: Create a new Python 3.6.6 virtual environment
Code:
pyenv virtualenv 3.6.6 virtualenv_name
Motivation: This use case is useful when you want to create a new virtual environment with a specific Python version, such as Python 3.6.6.
Explanation:
pyenv
: The command to manage Python versions and virtual environments.virtualenv
: The sub-command used to create virtual environments.3.6.6
: The version of Python to base the virtual environment on.virtualenv_name
: The name given to the virtual environment.
Example output:
created virtual environment CPython 3.6.6 in /path/to/virtualenv_name
Use case 2: List all existing virtual environments
Code:
pyenv virtualenvs
Motivation: This use case is helpful when you want to see a list of all virtual environments created using pyenv.
Explanation:
pyenv
: The command to manage Python versions and virtual environments.virtualenvs
: The sub-command used to list all existing virtual environments.
Example output:
virtualenv_name (created from /path/to/python3.6.6)
another_virtualenv (created from /path/to/python3.7.4)
Use case 3: Activate a virtual environment
Code:
pyenv activate virtualenv_name
Motivation: This use case is necessary when you want to activate a specific virtual environment and use it as the default Python environment.
Explanation:
pyenv
: The command to manage Python versions and virtual environments.activate
: The sub-command used to activate a virtual environment.virtualenv_name
: The name of the virtual environment to activate.
Example output: None.
Use case 4: Deactivate the virtual environment
Code:
pyenv deactivate
Motivation: This use case is used when you want to deactivate the currently activated virtual environment and switch back to the system Python installation.
Explanation:
pyenv
: The command to manage Python versions and virtual environments.deactivate
: The sub-command used to deactivate the currently activated virtual environment.
Example output: None.
Conclusion:
In this article, we explored the various use cases of the ‘pyenv virtualenv’ command. This command is useful for creating, listing, activating, and deactivating virtual environments based on different Python distributions. It provides a convenient way to manage Python environments and switch between them effortlessly.