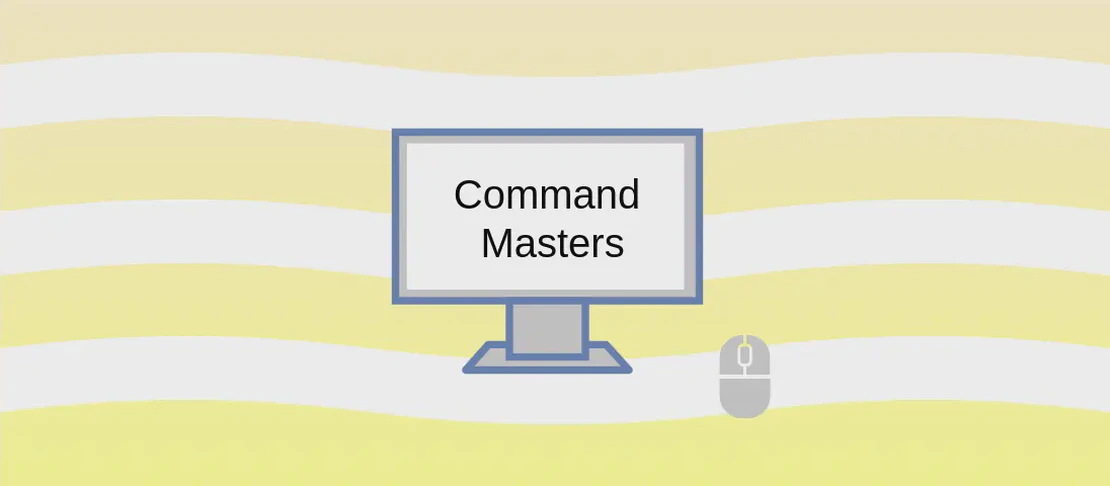
Using Pyflakes for Python Error Checking (with Examples)
Pyflakes is a command-line tool designed to detect errors in Python source code files without executing them. It focuses on identifying syntax errors and ensuring code cleanliness by highlighting potential issues like unused imports, undefined variables, and misused modules. This makes it an invaluable tool for developers who wish to maintain clean, efficient, and error-free code. Below are various use cases illustrating how Pyflakes can be used effectively for different purposes.
Use Case 1: Check a Single Python File
Code:
pyflakes check path/to/file.py
Motivation:
When working on a Python project, it is common to make changes to a single file. Before running the script or deploying the application, you want to ensure that there are no apparent mistakes in your code. Pyflakes allows developers to check a single Python file for syntax and logical errors, ensuring that coding errors are caught early in the development process.
Explanation:
pyflakes
: This is the command to invoke the Pyflakes tool.check
: An instruction to Pyflakes to perform a check on the specified file.path/to/file.py
: The path to the specific Python file you want to check. Replace this with the actual path to your file.
Example Output:
path/to/file.py:3: 'os' imported but unused
path/to/file.py:5: undefined name 'unkown_variable'
In this example, Pyflakes has identified that the module os
is imported but not used anywhere in the file. It also detected an undefined variable named unkown_variable
, indicating a potential typo or missed initialization.
Use Case 2: Check Python Files in a Specific Directory
Code:
pyflakes checkPath path/to/directory
Motivation:
When working on larger projects, multiple Python files might reside within a single directory. It’s efficient to check all these files at once rather than checking them individually. Pyflakes facilitates easy batch checking of files within a directory, enabling developers to detect errors across files quickly.
Explanation:
pyflakes
: This is the command to invoke the Pyflakes tool.checkPath
: A command to prompt Pyflakes to perform checks on each Python file within the specified directory.path/to/directory
: The directory path containing the Python files to be checked. Replace with your directory path.
Example Output:
path/to/directory/script1.py:7: undefined name 'functionA'
path/to/directory/module1.py:15: redefinition of unused 'variableX' from line 8
This output shows errors found in multiple files within the specified directory, such as an undefined function and an unused variable redefinition.
Use Case 3: Check Python Files in a Directory Recursively
Code:
pyflakes checkRecursive path/to/directory
Motivation:
In large projects, Python files are often organized in a hierarchical directory structure. Checking each directory individually can be tedious. Pyflakes allows developers to recursively check files in all subdirectories, providing a comprehensive review of the entire project’s codebase for errors in one go.
Explanation:
pyflakes
: This is the command to invoke the Pyflakes tool.checkRecursive
: A command to instruct Pyflakes to recursively check all Python files in the specified directory and its subdirectories.path/to/directory
: The parent directory path whose files and subdirectories you want to check.
Example Output:
path/to/directory/subdir1/fileA.py:23: 'math' imported but unused
path/to/directory/subdir2/fileB.py:9: undefined name 'undeclaredVariable'
Pyflakes reports errors from Python files across various subdirectories, providing an exhaustive check of the codebase.
Use Case 4: Check All Python Files Found in Multiple Directories
Code:
pyflakes iterSourceCode path/to/directory_1 path/to/directory_2
Motivation:
Sometimes, different modules or components of a project are housed in separate directories. Checking these directories individually can be cumbersome. By allowing multiple directories to be checked in a single command, Pyflakes simplifies this process, enabling efficient error detection across disparate code sections.
Explanation:
pyflakes
: This is the command to invoke the Pyflakes tool.iterSourceCode
: The command tells Pyflakes to iterate through and check all Python files within the specified directories.path/to/directory_1
: The first directory containing Python files to be checked.path/to/directory_2
: An additional directory for checking. You can include more directories as needed.
Example Output:
path/to/directory_1/utility.py:5: redefinition of unused 'logger' from line 2
path/to/directory_2/handler.py:12: undefined name 'process_data'
In this example, Pyflakes highlights issues like unused redefinitions and undefined names across multiple specified directories, providing a comprehensive view of potential errors.
Conclusion
Pyflakes is a powerful yet straightforward tool for maintaining error-free Python code. Whether you’re checking an individual file or an entire codebase across multiple directories, Pyflakes offers potent solutions to efficiently detect potential issues, facilitating cleaner and more reliable software development. This command can become an integral part of your code quality practices, helping you catch errors before they propagate into more significant problems.