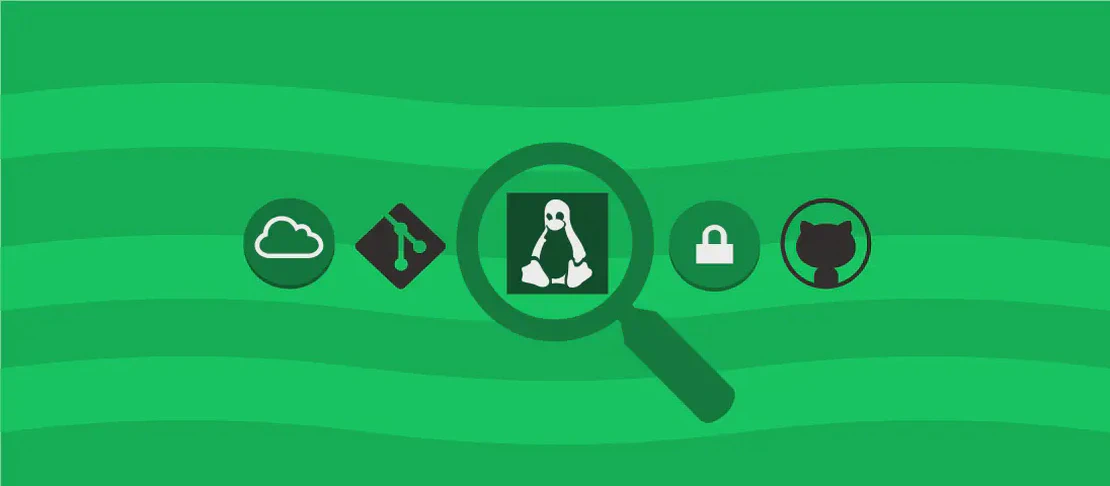
How to Use the Command 'pygmentize' (with Examples)
Pygmentize is a powerful command-line tool part of the Pygments library, specifically designed to highlight source code syntax in various programming languages. As a Python-based syntax highlighter, it brings color and additional formatting clarity to code scripts, making them more readable and presentable. It supports a multitude of languages and output formats, offering versatile options for developers and writers.
Use case 1: Highlight File Syntax and Print to stdout
Code:
pygmentize file.py
Motivation:
This use case is particularly useful when a developer wants to quickly view the syntax-highlighted version of their code directly in the terminal. This is often useful for debugging or code reviews where formatting errors need to be efficiently spotted without necessarily opening an IDE or a sophisticated text editor.
Explanation:
pygmentize
: The command-line tool that initiates the process of syntax highlighting.file.py
: The Python file whose syntax will be highlighted. The file extension helps pygmentize automatically infer the coding language and apply appropriate syntax highlight.
Example Output:
When you run this command, the terminal output will include the Python code with keywords, strings, and various constructs highlighted. For instance, def, class, and other Python keywords might appear in a different color than strings or comments.
Use case 2: Explicitly Set the Language for Syntax Highlighting
Code:
pygmentize -l javascript input_file
Motivation:
There are instances where the file extension might not correspond to the language actually being used, or a file might not have an extension at all. By specifying the language, users ensure that pygmentize accurately highlights the syntax based on the correct language rules.
Explanation:
-l javascript
: This option explicitly sets the language for syntax highlighting to JavaScript, overriding any inference that might be wrong due to misleading file extensions.input_file
: The file containing the source code that needs to be highlighted according to JavaScript syntax rules.
Example Output:
On executing, the tool outputs the file content with JavaScript-specific syntax highlighting, such as reserved words like function or var in distinct colors.
Use case 3: List Available Lexers
Code:
pygmentize -L lexers
Motivation:
This is an informative feature for users who wish to understand what programming languages and formats are supported by pygmentize. With numerous programming languages today, this feature helps in confirming or discovering support for lesser-known languages.
Explanation:
-L lexers
: Lists all available lexers, which are responsible for processing input languages. This flag essentially allows you to identify all the languages that pygmentize can comprehend and highlight.
Example Output:
The command results in a long list output to the terminal, where each entry specifies a language or a file extension that pygmentize can process. For instance, entries like Python
, JavaScript
, and HTML
that illustrate its versatile supporting of languages.
Use case 4: Save Output to a File in HTML Format
Code:
pygmentize -f html -o output_file.html input_file.py
Motivation:
When sharing code snippets as part of documentation or blogs, having them in HTML format allows for easy integration into web pages with formatting intact. This use case enables users to generate an HTML representation of their code, complete with syntax highlighting.
Explanation:
-f html
: Specifies the output format as HTML, which is essential for web-related content creation.-o output_file.html
: Directs the output to a file instead of stdout, useful for saving the results permanently.input_file.py
: Gives the input Python file whose contents need conversion and storage in HTML format.
Example Output:
The specified HTML file named output_file.html
will be created, containing the highlighted code. When this file is opened in a browser, you’ll see the beautifully formatted Python code with colors demonstrating different syntax elements.
Use case 5: List Available Output Formats
Code:
pygmentize -L formatters
Motivation:
This command is beneficial for users who wish to explore different formats in which they might want to render their syntax-highlighted code. From HTML to RTF or LaTeX, understanding the options available helps in selecting the right format for each use case.
Explanation:
-L formatters
: Lists all available output formats that pygmentize can support. This enables you to choose how the syntax-highlighted content should be structured in terms of file types and display styles.
Example Output:
A comprehensive list of all possible output format types is shown, indicating choices such as html
, latex
, rtf
, which can be utilized for rendering the code in various environments and styles.
Use case 6: Output an HTML File, with Additional Formatter Options
Code:
pygmentize -f html -O "full,linenos=True" -o output_file.html input_file
Motivation:
This command offers advanced customization for HTML output files, making them not only aesthetically pleasing but also more functional by marking line numbers and creating complete web pages. Useful for developers and writers who wish to enhance clarity in presentations or documentation.
Explanation:
-f html
: Designates the output format as HTML.-O "full,linenos=True"
: Modifier options to enhance the HTML, creating a full web page (full
) and adding line numbers (linenos=True
) to the code.-o output_file.html
: Specifies the target output file for saving the HTML.input_file
: The source file to be highlighted.
Example Output:
The output_file.html
is generated, structured as a complete HTML page with the requisite line numbers integrated. You will observe a well-organized, readable HTML document when opened in a browser.
Conclusion:
The pygmentize
command-line tool proves to be a versatile and invaluable utility for developers who handle a plethora of programming languages and require efficient syntax highlighting for different purposes. Whether you need immediate visualization in the terminal or a polished HTML page for public sharing, pygmentize
offers a straightforward yet powerful array of features to cater to such needs.