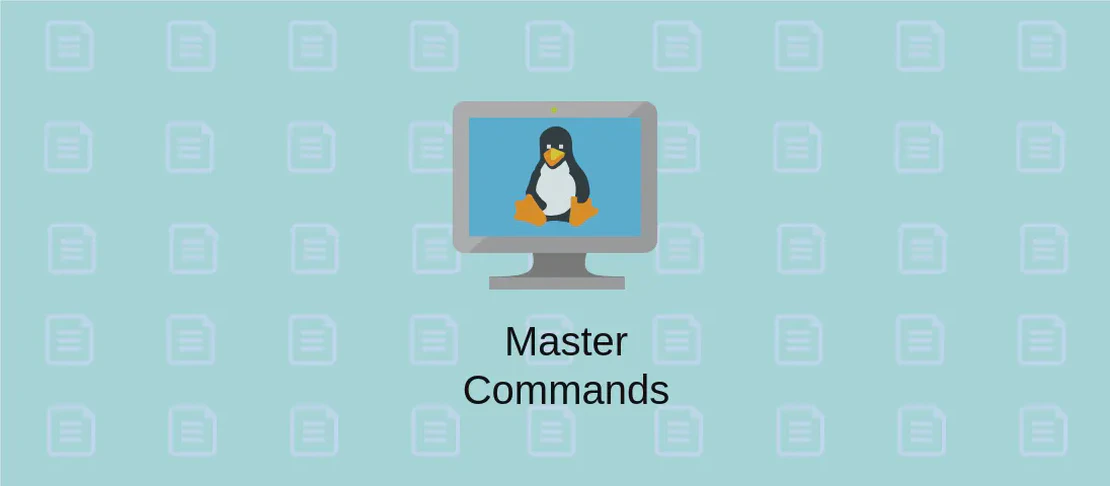
How to Use the Command 'pylint' (with Examples)
Pylint is a popular static code analysis tool in the Python ecosystem. It is used to check for errors in Python code, enforce a coding standard, and look for code smells. Pylint can help improve code quality by highlighting potential issues and enforcing best practices, making it an essential tool for Python developers who want to maintain code quality and consistency in their projects.
Use Case 1: Show Lint Errors in a File
Code:
pylint path/to/file.py
Motivation:
When developing or maintaining a Python script, it’s crucial to ensure that the code adheres to a certain coding standard without any syntactic or logical errors. Running pylint on a specific file helps identify potential errors and issues in that file, allowing developers to address them proactively. This practice is especially beneficial during the development phase to catch mistakes early on.
Explanation:
pylint
: This is the command for invoking the pylint tool.path/to/file.py
: This argument specifies the path to the Python file you wish to analyze. Pylint will run checks on this specific file.
Example Output:
************* Module example
C: 1,0: Missing module docstring (missing-module-docstring)
F: 4,0: Undefined variable 'undefined_var' (undefined-variable)
The output provides a list of issues found in file.py
, including missing documentation and the use of undefined variables, thereby guiding the user to improve their code quality.
Use Case 2: Lint a Package or Module
Code:
pylint package_or_module
Motivation:
When developing larger projects structured as packages or modules, it’s important to ensure that the entire package adheres to coding standards and is error-free. Running pylint on an entire package or module offers a comprehensive overview of potential issues across multiple files, thus giving developers insight into systemic issues rather than just isolated errors.
Explanation:
pylint
: This is the command for invoking the pylint tool.package_or_module
: This refers to the name of the package or module you want to lint. It must be importable in your Python environment and should not have a.py
extension. This allows pylint to consider the package or module comprehensively rather than as a single script.
Example Output:
************* Module mypackage.module
W: 12,4: Redefining built-in 'id' (redefined-builtin)
R: 25,0: Too many return statements (15/6) (too-many-return-statements)
The output provides feedback on problematic parts of code, checking for issues like redefinitions of built-in variables and suggesting improvements for code structure.
Use Case 3: Lint a Package from a Directory Path
Code:
pylint path/to/directory
Motivation:
For projects organized into directories, especially those representing extensive applications or libraries, it is necessary to ensure consistency in convention and logic across all included Python scripts. Using pylint with a directory path allows developers to validate the entirety of a package defined by directories, ensuring that the package’s design and implementation follow best practices collectively.
Explanation:
pylint
: This is the command for invoking the pylint tool.path/to/directory
: This specifies the directory containing the package you want to lint. The directory should contain an__init__.py
file, which tells Python that this directory should be treated as a package.
Example Output:
************* Module mypackage.submodule
C: 5,0: Missing class docstring (missing-docstring)
E: 9,2: Method could be a function (no-self-use)
Output showcases identified areas for improvement within the specified package, highlighting concerns such as documentation absence or method design issues, which are important for keeping the package maintainable and readable.
Use Case 4: Lint a File and Use a Configuration File
Code:
pylint --rcfile path/to/pylintrc path/to/file.py
Motivation:
Every project can have its own unique coding style or specific rules that need to be enforced. By using a pylint configuration file, developers can customize pylint’s behavior to accommodate specific project requirements, allowing for flexibility in how errors and coding standards are handled and enforced across different projects.
Explanation:
pylint
: This is the command for invoking the pylint tool.--rcfile path/to/pylintrc
: This option lets you specify the path to a custom configuration file, commonly namedpylintrc
. This file can override the default pylint settings, offering tailored linting rules that suit the project’s needs.path/to/file.py
: Indicates the specific file you intend to lint under the settings defined by thepylintrc
file.
Example Output:
************* Module config_example
W: 8,4: Redefining built-in 'print' (redefined-builtin)
C: 2,0: Line too long (120/80) (line-too-long)
The output demonstrates issues reported based on the custom rules specified in the pylintrc
file, showcasing how specific rules or tolerances can be customized per project requirements.
Use Case 5: Lint a File and Disable a Specific Error Code
Code:
pylint --disable C,W,no-error,design path/to/file.py
Motivation:
There are situations where certain pylint checks might not be necessary or relevant for the given project or script, such as temporary workarounds during development. Disabling specific error codes allows developers to focus on relevant warnings and errors while ignoring others, thereby making the linting output more manageable and focused.
Explanation:
pylint
: This is the command for invoking the pylint tool.--disable C,W,no-error,design
: This option allows you to disable certain message categories or specific pylint checks. In this context, categories C (convention), W (warning), and specific codes likeno-error
ordesign
are suppressed from the output.path/to/file.py
: This specifies the file you want to check while ignoring the specified pylint categories or messages.
Example Output:
************* Module myscript
R: 10,4: Attribute name "myVariable" doesn't conform to snake_case naming style (invalid-name)
F: 12,4: Undefined variable 'x' (undefined-variable)
Here, the output only reports on issues outside the disabled categories, such as refactoring suggestions and fatal errors, which helps streamline attention to the most critical parts that need developer action.
Conclusion
The examples demonstrated above illustrate various ways to use the pylint command to validate and improve Python code. From assessing single files to entire packages to customizing lint output, pylint stands as a robust tool in a developer’s toolkit aimed at maintaining code quality and adhering to coding standards. Each use case highlights the versatility of pylint in adapting to different project requirements and development workflows.