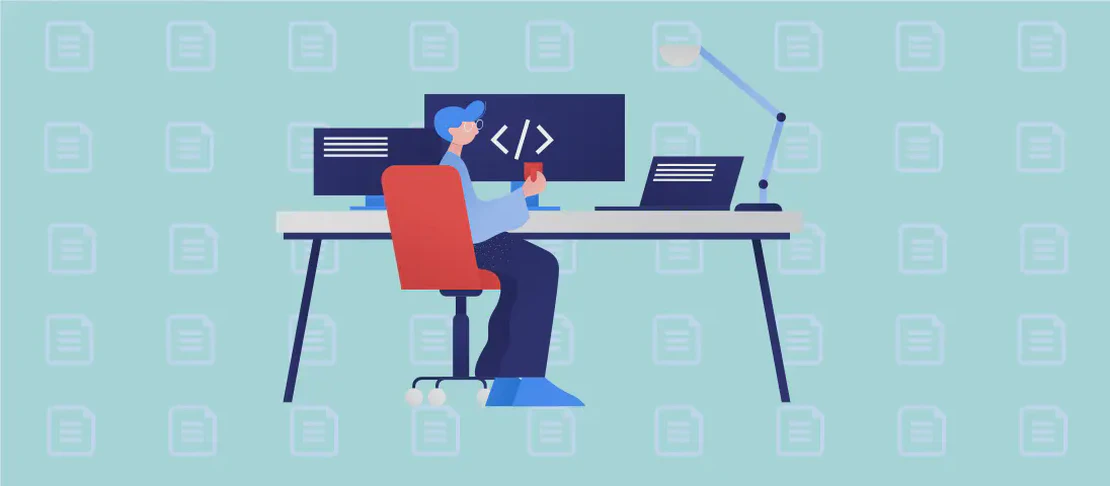
How to Use the Command 'pypy' (with examples)
PyPy is a fast and compliant alternative implementation of the Python language. It aims to provide better performance compared to the standard CPython interpreter, thanks to its Just-In-Time (JIT) compiler, which enhances execution speed for many Python applications. With its compatibility and efficiency in executing Python scripts, PyPy is an invaluable tool for developers seeking to optimize performance in Python programming. Below, we explore various use cases of the pypy
command, demonstrating its versatility and effectiveness through several examples.
Use case 1: Start a REPL (interactive shell)
Code:
pypy
Motivation:
Starting a REPL (Read-Eval-Print Loop) allows developers to write and test small code snippets interactively. This is invaluable for quick experiments, debugging, and learning purposes. Using PyPy’s REPL can also help developers experience the improved performance of their code execution in a more immediate environment compared to other Python interpreters.
Explanation:
The command pypy
without any arguments initiates an interactive PyPy REPL environment, where users can enter Python code line by line and get instant feedback.
Example Output:
Python 3.x.x (ae3e5dsaf, Mar 27 2021, 16:30:40)
[PyPy 7.3.x with GCC 9.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
And now for something completely different: Peace Love and Rockets
>>> print("Hello from PyPy!")
Hello from PyPy!
>>>
Use case 2: Execute script in a given Python file
Code:
pypy path/to/file.py
Motivation:
This command is essential for running Python scripts that are already saved as files. Especially in production or automated environments where you need to run tasks or applications defined within script files, doing so directly with PyPy ensures the scripts benefit from PyPy’s performance enhancements.
Explanation:
pypy
: Invokes the PyPy interpreter.path/to/file.py
: Specifies the file path to the Python script that needs to be executed.
Example Output:
Assuming file.py
contains:
print("Execution with PyPy!")
The output will be:
Execution with PyPy!
Use case 3: Execute script as part of an interactive shell
Code:
pypy -i path/to/file.py
Motivation:
Executing a script and then entering an interactive shell is particularly useful for testing and debugging. It allows the developer to explore and manipulate the program’s environment and variables interactively after the script is executed.
Explanation:
pypy
: Launches the PyPy interpreter.-i
: Tells PyPy to remain in the interactive mode even after the script is executed.path/to/file.py
: Script file to be executed prior to entering interactive mode.
Example Output:
Assuming file.py
is:
message = "Script executed!"
print(message)
Running the command will result in:
Script executed!
>>> print(message)
Script executed!
>>>
Use case 4: Execute a Python expression
Code:
pypy -c "expression"
Motivation:
Executing Python expressions directly from the command line is suited for quick, one-off, and often simple tasks without having to create a separate script file. This is useful for automation scripts or simple calculations performed directly from the terminal.
Explanation:
pypy
: The PyPy interpreter is initiated.-c
: This flag indicates that the following string is a Python expression to be executed."expression"
: A valid Python code snippet, such as a calculation or a function call.
Example Output:
For the expression:
pypy -c "print(3 * 4)"
The output will be:
12
Use case 5: Run library module as a script (terminates option list)
Code:
pypy -m module arguments
Motivation:
Running Python standard library modules as scripts is beneficial when you want to utilize module-specific functionalities directly from the command line. PyPy supports running these modules, which allows for quicker or more efficient execution.
Explanation:
pypy
: The PyPy interpreter.-m
: Indicates that the following module should be run as a script.module
: The library module you wish to execute.arguments
: Additional options or arguments needed by the module.
Example Output:
Running a simple HTTP server using the http.server
module:
pypy -m http.server 8000
Example Output:
Serving HTTP on 0.0.0.0 port 8000 ...
Use case 6: Install a package using pip
Code:
pypy -m pip install package
Motivation:
Using PyPy’s version of pip ensures that any installed packages are compatible with the PyPy environment, which can sometimes differ from CPython in terms of available packages and performance optimizations.
Explanation:
pypy
: Initiates the PyPy interpreter.-m pip
: Runs the pip module as a script.install
: The pip command for installing packages.package
: The specific package you wish to install.
Example Output:
For example, installing requests
:
pypy -m pip install requests
The output might include:
Collecting requests
Downloading requests-2.x.x-py2.py3-none-any.whl (58 kB)
Installing collected packages: requests
Successfully installed requests-2.x.x
Use case 7: Interactively debug a Python script
Code:
pypy -m pdb path/to/file.py
Motivation:
Debugging is a critical part of software development. Using PyPy with pdb
, Python’s built-in debugger, helps developers inspect and modify the state of a program while running, empowering them to better understand and fix issues in their code efficiently.
Explanation:
pypy
: Calls the PyPy interpreter.-m pdb
: Uses the Python debugger module to run the script.path/to/file.py
: The script file you want to debug.
Example Output:
Starting a debugging session for file.py
:
pypy -m pdb path/to/file.py
Produces an interactive debug prompt:
> /path/to/file.py(1)<module>()
-> print("Debugging with PyPy!")
(Pdb)
Conclusion:
PyPy offers a compelling alternative to the standard Python interpreter, especially for performance-focused applications. Through various use cases like running scripts, entering interactive modes, handling packages, and debugging, the pypy
command provides extensive support for improving and simplifying the development process. Whether for quick tasks or comprehensive application execution, PyPy’s integration of speed and compatibility ensures it is a valuable tool for Python developers.