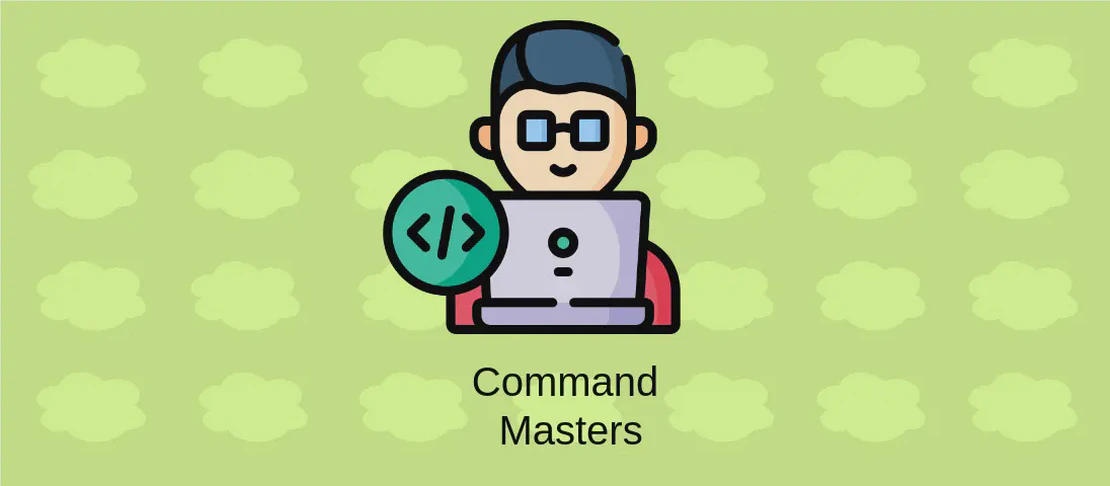
How to Use the Python Command (with Examples)
The Python command is a versatile tool that allows users to interact with the Python language’s capabilities directly from the command line. It is used for executing Python scripts, starting interactive sessions, and performing various tasks associated with Python programming. Python’s command-line interface offers a broad range of applications, ranging from starting a simple session to running a full-fledged debugging process. More information about Python can be found at Python’s official website .
Use case 1: Start a REPL (interactive shell)
Code:
python
Motivation:
The Read-Eval-Print Loop (REPL) provides an interactive environment where you can execute Python commands one at a time, observe their effects, and quickly test bits of code or explore new libraries. It’s an excellent tool for learning Python, debugging, or trying out new algorithms and data structures on the fly.
Explanation:
Executing the command python
without any additional arguments opens an interactive shell. This shell evaluates user input, executes the corresponding Python code, and prints the output. The interactive prompt typically presents itself as >>>
.
Example Output:
Python 3.9.1 (default, Dec 8 2020, 20:11:12)
[GCC 8.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>>
Use case 2: Execute a specific Python file
Code:
python path/to/file.py
Motivation:
Executing a Python file directly from the command line allows developers to run their written scripts or applications quickly. This eliminates the need to open an editor or integrated development environment (IDE) for running the code and is particularly useful for automated scripts and programs scheduled via task schedulers or cron jobs.
Explanation:
In this command, path/to/file.py
represents the path to the Python script you wish to execute. Python reads the file, executes the contents as a script, and then exits.
Example Output:
If file.py
contained a simple print statement like print("Hello, World!")
, running the command would produce:
Hello, World!
Use case 3: Execute a specific Python file and start a REPL
Code:
python -i path/to/file.py
Motivation:
Sometimes, after executing a script, you may want to continue working in an interactive environment, inspecting variables or testing further code. Using the -i
option, you can run a script and immediately enter the REPL with the script’s environment still in memory.
Explanation:
The -i
flag tells Python to enter interactive mode after running the script. This allows you to access and manipulate any variables, functions, or other constructs defined in the script.
Example Output:
Considering that file.py
contains x = 10; print("Running script")
, executing the command yields:
Running script
>>> x
10
>>>
Use case 4: Execute a Python expression
Code:
python -c "expression"
Motivation:
Using the -c
option is helpful for quick one-off scripts or tests where you don’t want to create a separate file just for a short command or script. It facilitates running a piece of Python code directly from the command line.
Explanation:
The -c
flag makes Python execute the command given as a string rather than running a file. The string can include any Python expression or block of code.
Example Output:
If run with the expression print(2 + 2)
, it returns:
4
Use case 5: Run the script of the specified library module
Code:
python -m module arguments
Motivation:
Running a module as a script is particularly useful for executing library-maintained scripts, such as running test suites or convenience scripts included within a package. This method ensures the module is invoked in the correct context and with necessary libraries.
Explanation:
The -m
option allows you to run a library module directly, making it behave like a script. You can also pass additional arguments to the module, which are accessible via sys.argv
.
Example Output:
For instance, using the timeit
module to measure execution time, you might see:
python -m timeit -r 5 "for i in range(1000): pass"
10000 loops, best of 5: 30 usec per loop
Use case 6: Install a package using pip
Code:
python -m pip install package
Motivation:
Managing packages is one of Python’s strengths, allowing you to add extra functionality to your applications easily. pip
is the package installer for Python, and this command installs a specified package from the Python Package Index (PyPI).
Explanation:
Here, pip
is run as a module with -m
. The install
command specifies that pip should download and install the package specified by package
. This is helpful when managing Python environments where pip
is not installed globally.
Example Output:
For installing requests
, you might see:
Collecting requests
...
Successfully installed requests-2.25.1
Use case 7: Interactively debug a Python script
Code:
python -m pdb path/to/file.py
Motivation:
Debugging is an essential part of software development. The Python debugger (pdb
) allows developers to execute a script step-by-step, inspect variables, and set breakpoints, providing fine control over the execution process.
Explanation:
By using -m pdb
, you run the pdb
module as a script, which starts it in the debugging mode with your script. This begins running your script and pauses on the first line for inspection.
Example Output:
Running this on a script prints initialization messages and shows the first line of the script:
> /path/to/file.py(1)<module>()
-> x = 10
(Pdb)
Use case 8: Start the built-in HTTP server on port 8000 in the current directory
Code:
python -m http.server
Motivation:
A built-in HTTP server is convenient for previewing websites, sharing files quickly in a network, or serving simple APIs during development without needing a full-fledged web server like Apache or Nginx.
Explanation:
The command runs the http.server
module, which sets up an HTTP server on the default port 8000. The server serves files and directories within the current directory.
Example Output:
Starting this server from a terminal at a directory might show:
Serving HTTP on 0.0.0.0 port 8000 (http://0.0.0.0:8000/) ...
Conclusion
The Python command-line interface is a flexible tool catering to various requirements ranging from interactive coding sessions, executing scripts, installing packages, debugging, and even serving files over HTTP. Its versatility is one of Python’s many strengths, making it an essential tool for both beginners and seasoned developers.