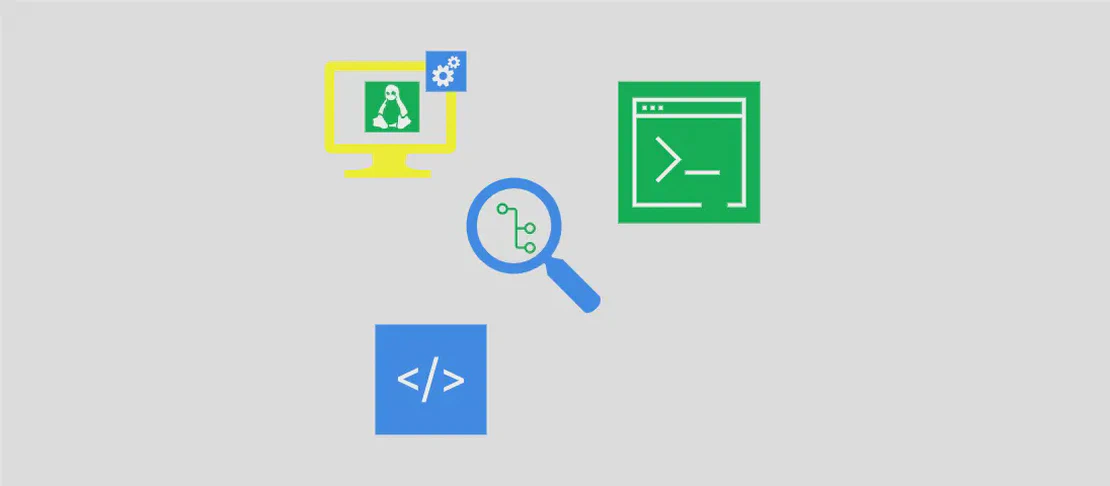
How to use the command 'racket' (with examples)
Racket is a dynamic, multi-paradigm programming language that belongs to the Lisp-Scheme family of languages. It is well-known for its powerful macro system, which makes Racket a great tool for language creation and experimentation. The Racket language interpreter provides the capacity to write, execute, and test Racket code in various ways. This article illustrates some of the common use cases of the racket
command, helping you understand how to utilize its functionalities effectively.
Use case 1: Starting a REPL (interactive shell)
Code:
racket
Motivation: A REPL (Read-Eval-Print Loop) is an interactive environment that allows a programmer to experiment with code snippets, perform calculations, and receive immediate feedback. This is particularly useful for learning, debugging, or rapid prototyping, where you might want to test individual Racket expressions or functions quickly without the need to write a complete script or program.
Explanation: The command racket
without any additional arguments launches the interactive shell. This is the environment where you can input Racket expressions line-by-line, and each input will be processed instantaneously with its result displayed below. It is a quick way to interface directly with the compiler and interpreter of Racket.
Example Output:
Welcome to Racket v8.3.0.1.
> (+ 2 3)
5
> (define (square x) (* x x))
> (square 5)
25
Use case 2: Executing a Racket script
Code:
racket path/to/script.rkt
Motivation: Script execution is essential for running complete Racket programs that are stored in files. This is especially useful for longer, more complex projects that are beyond the scope of exploratory scripting in a REPL, involving multiple definitions and interactions that form a cohesive program.
Explanation: The racket
command followed by the path to the script file tells the interpreter to read and execute the Racket code contained in the specified file. The file extension .rkt
denotes a Racket script file. This is how you would run standalone Racket programs that have already been written and saved.
Example Output:
Hello, Racket World!
The result of calculation is 42.
Use case 3: Executing a Racket expression
Code:
racket --eval "(+ 2 2)"
Motivation: There are scenarios where you might want to quickly evaluate a single Racket expression from the command line without opening a REPL or running a full script. This could be for automated scripts, inline execution in a larger automation pipeline, or just checking the result of an expression quickly.
Explanation: The argument --eval
is used to directly evaluate the next string as a Racket expression from the command line. It requires the expression following it in quotation marks. This inline evaluation is useful for scripting and should be used for relatively simple Racket expressions.
Example Output:
4
Use case 4: Running a module as a script
Code:
racket --lib module_name --main arguments
Motivation: Racket’s module system allows you to organize code into reusable components. Running a module directly as a script can be helpful when testing or deploying modular components independently, or when interacting with larger systems that include modules.
Explanation: The --lib
flag is used to specify a library module that should be loaded, and --main
indicates to treat the module as an executable script, often followed by any necessary arguments. The option list is terminated before --main
, so any subsequent command line arguments are passed to the module’s main procedure.
Example Output:
Module loaded successfully.
Program executed with arguments: arg1, arg2
Use case 5: Starting a REPL for the typed/racket
hashlang
Code:
racket -I typed/racket
Motivation: Typed Racket is an extension of Racket that adds static type checking, enhancing the robustness and reliability of your program by catching type-related errors at compile-time rather than run-time. Testing code with Typed Racket in a REPL allows you to ensure that the type system is being correctly satisfied and to explore the interplay of types in your code.
Explanation: The -I
option specifies the language pack to be used, in this case, typed/racket
. This starts the REPL in the context of Typed Racket, giving you the ability to write type-checked Racket code interactively.
Example Output:
Welcome to Typed Racket REPL.
> (: square (Integer -> Integer))
> (define (square x) (* x x))
> (square 5)
25
Conclusion
The Racket command offers multiple ways to interact with the Racket programming language, each fitting different development needs from quick experimentation to running full applications. These use cases help illustrate how flexible and powerful the racket
command can be, creating an engaging environment both for novices delving into the language and seasoned developers seeking powerful language development tools.