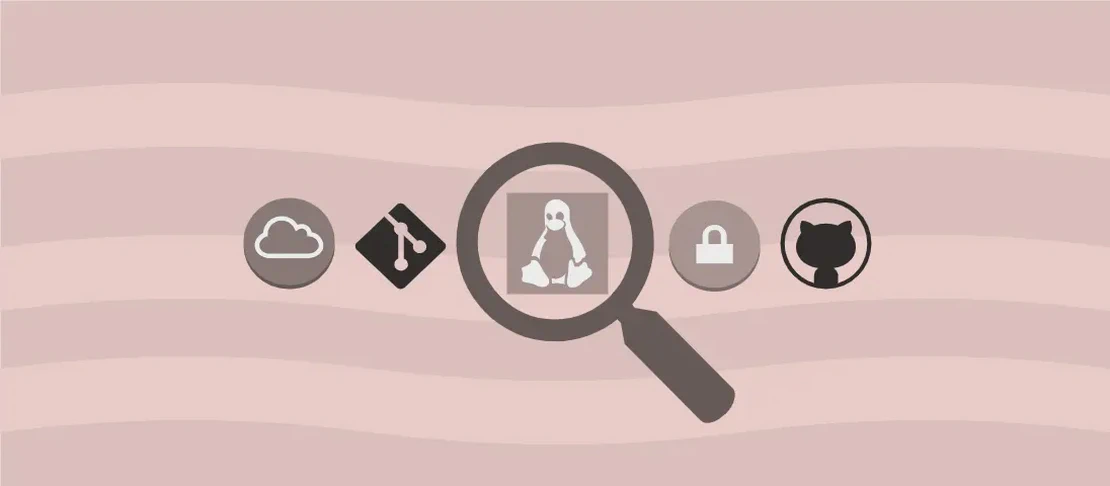
How to Use the Command 'rails db' (with examples)
The command rails db
is a versatile utility in the Ruby on Rails framework that provides a variety of subcommands for managing the database layer of a Rails application. These subcommands simplify complex tasks related to database creation, manipulation, and maintenance, offering developers an efficient workflow to handle data operations seamlessly. The utility acts as a bridge to ensure that database actions can be performed effortlessly without delving into low-level database commands. These commands enable developers to create databases, run migrations, seed data, and troubleshoot database issues with ease.
Use Case 1: Create Databases, Load the Schema, and Initialize with Seed Data
Code:
rails db:setup
Motivation:
The rails db:setup
command is particularly useful when setting up a new development environment or resetting an existing one. By combining multiple database-related tasks into a single command, it helps in quickly creating a clean database state, setting up the necessary structure, and populating it with initial data. This is invaluable during initial project setup or when new team members onboard a project and need to mimic the production database state for testing or development.
Explanation:
rails
: This invokes the Rails command-line interface, which is the primary mechanism for interacting with a Rails application through the terminal.db:setup
: This is a composite task that, under the hood, combines three distinct operations:db:create
,db:schema:load
, anddb:seed
. This means it creates the databases, loads the schema fromdb/schema.rb
, and seeds the database with data fromdb/seeds.rb
.
Example Output:
Created database 'development_database'
Loaded schema for development environment
Seeding development database
Use Case 2: Access the Database Console
Code:
rails db
Motivation: Accessing the database console provides developers with a real-time interface to interact with the database. It allows you to directly issue SQL commands, inspect data, and perform ad-hoc queries or modifications. This is particularly beneficial for troubleshooting, quick data checks, or manually applying small data changes without modifying the application code.
Explanation:
rails
: Initiates the Rails command-line interface.db
: A shorthand command that opens the database console specific to the current Rails environment (e.g., development or production).
Example Output:
psql (PostgreSQL) 12.4
Type "help" for help.
development_database=#
Use Case 3: Create the Databases Defined in the Current Environment
Code:
rails db:create
Motivation:
Utilizing rails db:create
is essential at the beginning of a new project or when switching to a fresh environment where the existing databases have not yet been instantiated. This command automates the process of database creation, which is indispensable when setting up a new application instance or a new environment like testing or production.
Explanation:
rails
: Launches the command-line interface for Rails applications.db:create
: Specifically instructs Rails to create the databases specified in the current environment configuration, as defined inconfig/database.yml
.
Example Output:
Created database 'development_database'
Created database 'test_database'
Use Case 4: Destroy the Databases Defined in the Current Environment
Code:
rails db:drop
Motivation:
The rails db:drop
command is useful when you need to completely remove the existing databases, often as a preparatory step before reset or when ensuring no previous data persists in the environment. This is crucial during clean-up operations or when testing migration setups.
Explanation:
rails
: Engages the Rails command-line interface.db:drop
: Executes the task to delete all databases linked to the current environment setup, effectively reversingdb:create
.
Example Output:
Dropped database 'development_database'
Dropped database 'test_database'
Use Case 5: Run Pending Migrations
Code:
rails db:migrate
Motivation:
Applying migrations with rails db:migrate
is a crucial step in evolving your database schema over time. It ensures all pending migrations are applied sequentially, maintaining consistency between the application’s code and its database schema. This is essential when deploying code to different environments which require consistent database structures.
Explanation:
rails
: Initiates the Rails command framework.db:migrate
: Runs migrations that have not yet been executed in the current environment, applying changes specified indb/migrate/*.rb
.
Example Output:
== 20231015123045 CreateUsers: migrating ======================================
-- create_table(:users)
-> 0.0023s
== 20231015123045 CreateUsers: migrated (0.0024s) =============================
Use Case 6: View the Status of Each Migration File
Code:
rails db:migrate:status
Motivation: Determining which migrations have been applied is critical for both debugging and verifying the consistency of the database schema across different environments. It helps developers ascertain which migrations are pending or if there are discrepancies, thereby facilitating smoother deployment processes.
Explanation:
rails
: Engages the Rails command-line interface.db:migrate:status
: Produces a detailed table showing the status of each migration file, whether pending, up, or down, based on the schema_migrations table.
Example Output:
database: development_database
Status Migration ID Migration Name
--------------------------------------------------
up 20231015123045 CreateUsers
down 20231016124512 AddEmailsToUsers
Use Case 7: Rollback the Last Migration
Code:
rails db:rollback
Motivation: In cases where a migration has had unintended consequences or introduced errors, rolling back is necessary to return the database to a previous state. This functionality supports quick corrective measures in iterative development processes when experimenting with database changes.
Explanation:
rails
: Launches the Rails command interface.db:rollback
: Reverts the most recent migration applied, providing a mechanism for undoing changes housed within a single migration step.
Example Output:
== 20231016124512 AddEmailsToUsers: reverting =================================
-- remove_column(:users, :email)
-> 0.0015s
== 20231016124512 AddEmailsToUsers: reverted (0.0016s) ========================
Use Case 8: Fill the Current Database with Data Defined in db/seeds.rb
Code:
rails db:seed
Motivation: Loading seed data into the database is crucial for testing environments or initial setup processes where a certain data state is necessary to run and test application logic. This ensures that the application has all pre-requisite data, enabling developers to test functionalities with pre-defined conditions.
Explanation:
rails
: Activates the Rails command-line interface.db:seed
: Executes thedb/seeds.rb
file, inserting the predefined data and allowing you to set up initial conditions for your application.
Example Output:
Seeding (20231018) Initial data for User Model
Conclusion:
The rails db
command and its subcommands represent an integral part of streamlining database operations in Rails applications. Whether you’re setting up initial databases, migrating schemas, or seeding test data, mastering these commands can significantly enhance a developer’s ability to manage complex database interactions efficiently. Understanding these use cases aids in the seamless transition between different environments and ensures high consistency and reliability in database-dependent processes within the Rails ecosystem.