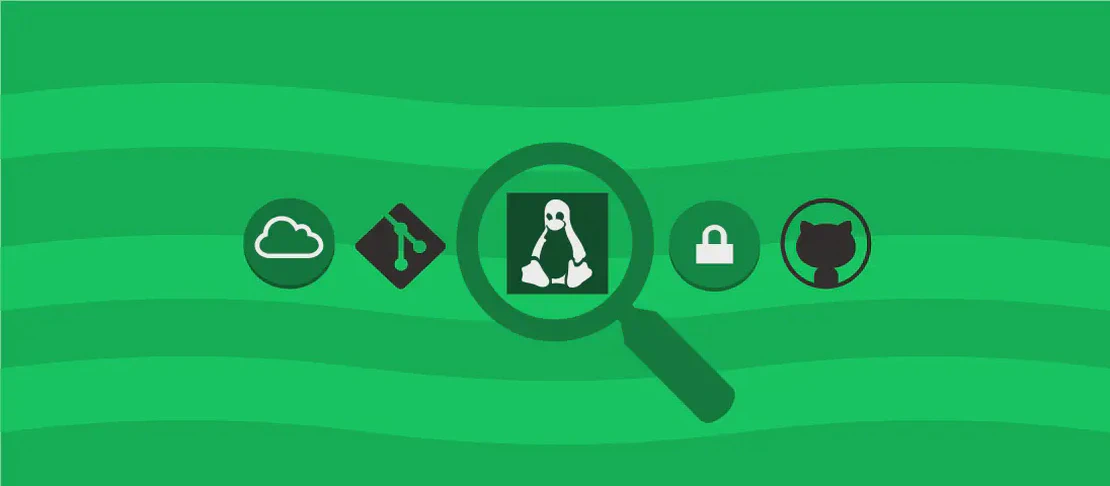
How to Use the Command 'rails destroy' (with Examples)
In the world of Ruby on Rails, the rails destroy
command is an invaluable tool for developers looking to efficiently manage and remove unwanted resources from their applications. This command is effectively the reverse of rails generate
, allowing you to undo the creation of models, controllers, migrations, and even entire scaffolds. As projects evolve, removing outdated or unnecessary components is crucial for maintaining clean, efficient, and manageable codebases. Below are several use cases demonstrating how to use the rails destroy
command effectively.
Use Case 1: List All Available Generators to Destroy
Code:
rails destroy
Motivation:
Sometimes, developers may not remember the exact element or resource they wish to destroy. By executing rails destroy
without any arguments, you can list all possible generators that can be reversed using the destroy command. This provides a comprehensive view of what’s available for removal and ensures that all actions align with project needs.
Explanation:
Here, rails destroy
is used without any additional arguments. Running it plain will prompt Rails to display all the generators it can reverse. This includes generators for models, controllers, scaffolds, etc., acting as a point of reference for the developer.
Example Output:
On executing this command, you’ll receive a list of generators, typically structured similarly to the ones in use by rails generate
. This helps verify potential undo actions and plan subsequent development steps effectively.
Use Case 2: Destroy a Model Named Post
Code:
rails destroy model Post
Motivation:
Models are core components of a Rails application, responsible for data representation and logic. However, project requirements change, and certain models may become redundant. Using rails destroy model Post
helps cleanly remove the Post model and its associated files, ensuring the codebase only contains necessary components.
Explanation:
rails destroy
: Initiates the destroy action.model
: Specifies that the type of resource to be destroyed is a model.Post
: The specific model name that is being targeted for destruction.
Example Output:
Executing this command will remove app/models/post.rb
, the migration file that created the posts table, test files and possibly fixtures related to the Post model. The output will list each removed file as a confirmation of successful action.
Use Case 3: Destroy a Controller Named Posts
Code:
rails destroy controller Posts
Motivation:
Controllers serve as intermediaries between models and views. As applications are refactored or redesigned, some controllers, like the Posts controller, might become obsolete. Utilizing this command ensures these no longer needed controllers are removed, helping maintain a well-structured and efficient Rails application.
Explanation:
rails destroy
: Commands Rails to trigger a reverse generator process.controller
: Indicates that the resource being removed is a controller.Posts
: Specifies which controller should be destroyed.
Example Output:
On running the command, Rails will delete app/controllers/posts_controller.rb
, associated view files, and test files pertaining to Posts. It will log each deletion as confirmation for the developer.
Use Case 4: Destroy a Migration that Creates Posts
Code:
rails destroy migration CreatePosts
Motivation:
Database tables and structure evolve with project needs. Occasionally, a migration might be unnecessary or in need of replacement. This command can efficiently remove the migration file, such as CreatePosts
, preventing any undesired schema changes from persistent execution in the future.
Explanation:
rails destroy
: Activates the destroy sequence within Rails.migration
: Specifies that a database migration is the target.CreatePosts
: The name or identifier of the specific migration to reverse.
Example Output:
After running this command, the migration file responsible for creating the posts table (db/migrate/XXX_create_posts.rb
) will be removed. Rails will verify and display the removal of this file.
Use Case 5: Destroy a Scaffold for a Model Named Post
Code:
rails destroy scaffold Post
Motivation:
Scaffolds in Rails provide a rapid way to set up views, controllers, models, and even tests for a resource. Upon re-evaluation, some of these auto-generated units, such as a complete scaffold for the Post model, might prove unnecessary. Destroying a scaffold helps revert these changes effortlessly, promoting a clutter-free development environment.
Explanation:
rails destroy
: Initiates the reversed action of generating scaffold components.scaffold
: Indicates a comprehensive teardown, encompassing model, views, controller, migrations, and tests.Post
: Identifies which resource scaffold to dismantle.
Example Output:
The command will result in simultaneous removal of app/models/post.rb
, app/views/posts
, app/controllers/posts_controller.rb
, associated tests, and migration files. Each step is logged, confirming the scaffold’s successful removal.
Conclusion:
The rails destroy
command is a robust mechanism for safely reversing components in a Ruby on Rails application. Each example here demonstrates its utility in real-world scenarios, aiding developers in maintaining lean, manageable, and optimized Rails projects through efficient removal of unnecessary code.