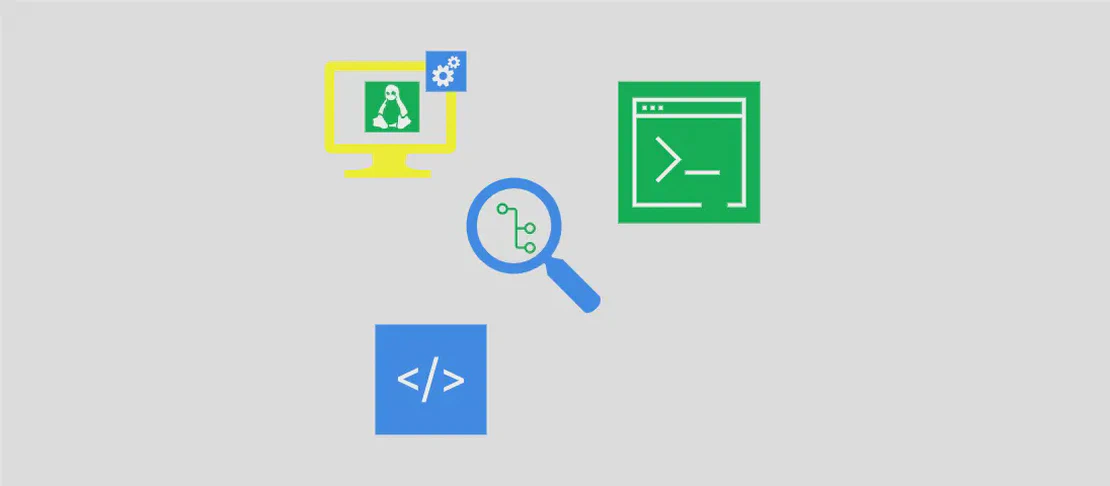
How to use the command 'rails generate' (with examples)
The rails generate
command is a versatile tool in the Ruby on Rails framework that helps developers scaffold various components of a Rails application. It significantly speeds up development by automating the creation of necessary files and code structure, thereby allowing developers to focus on the application logic rather than boilerplate code. This command can generate models, controllers, views, migrations, and even entire scaffolds with defined attributes, thus providing a foundational framework for new features or modifications.
Use case 1: List all available generators
Code:
rails generate
Motivation:
Before diving into specific tasks within a Rails application, it can be highly beneficial to understand what generators are available by default or through installed gems. Knowing the available options allows developers to explore and harness the full potential of the Rails framework, thus optimizing their workflow and leveraging existing tools efficiently.
Explanation:
rails
: This is the command-line tool used to interface with Ruby on Rails.generate
: This command is used to create new files from available templates. By not specifying further arguments, it lists all generators available for use in the current Rails environment.
Example Output:
Running the rails generate
command without any additional arguments will output a comprehensive list of available generators. This typically includes built-in generators like “model,” “controller,” “scaffold,” and any additional generators provided by other gems:
Rails:
controller
generator
helper
install
integration_test
mailer
migration
model
resource
scaffold
scaffold_controller
system_test
task
[...]
Use case 2: Generate a new model named Post with attributes title and body
Code:
rails generate model Post title:string body:text
Motivation:
Creating a model with specific attributes is one of the fundamental steps when setting up the database layer of an application. This command facilitates the creation of a new model which represents a table in the database, thereby defining the schema with specified fields. Automating this process ensures consistency and accuracy, especially in complex applications with numerous models.
Explanation:
rails
: Invokes the Rails command-line tool.generate
: Initiates the file generation process.model
: Specifies that we want to create a new model.Post
: This is the name given to the new model, representing a table in the database namedposts
.title:string
: Defines an attributetitle
of typestring
, which typically stores short pieces of text.body:text
: Adds an attributebody
of typetext
, suitable for larger blocks of text content.
Example Output:
Executing the command will generate several files that constitute the Post model, including:
invoke active_record
create db/migrate/20231010123456_create_posts.rb
create app/models/post.rb
invoke test_unit
create test/models/post_test.rb
create test/fixtures/posts.yml
Use case 3: Generate a new controller named Posts with actions index, show, new and create
Code:
rails generate controller Posts index show new create
Motivation:
Controllers are pivotal in Rails applications as they handle the application’s requests and responses. By specifying actions during generation, this command not only creates the necessary controller but sets up starter methods for each action to direct the application’s flow. This helps streamline the development process by reducing manual setup.
Explanation:
rails
: Initiates the use of Rails tools.generate
: Triggers the creation of specified components.controller
: Signals that a new controller is to be created.Posts
: The name of the controller, which, by convention, is pluralized.index show new create
: These are the actions, or methods, set up in the controller that correspond to common CRUD operations and provide a basic framework for handling web requests.
Example Output:
The command generates a controller file along with routes and view templates for the specified actions:
create app/controllers/posts_controller.rb
invoke erb
create app/views/posts
create app/views/posts/index.html.erb
create app/views/posts/show.html.erb
create app/views/posts/new.html.erb
create app/views/posts/create.html.erb
invoke test_unit
create test/controllers/posts_controller_test.rb
invoke helper
create app/helpers/posts_helper.rb
invoke assets
invoke scss
create app/assets/stylesheets/posts.scss
Use case 4: Generate a new migration that adds a category attribute to an existing model called Post
Code:
rails generate migration AddCategoryToPost category:string
Motivation:
As applications evolve, there is often a need to modify existing database schemas. This command enables developers to create a migration file that adds a new category
attribute to an existing Post
model, ensuring that changes are tracked and versioned properly, which is crucial for maintaining database consistency and facilitating smooth deployment processes.
Explanation:
rails
: Utilizes the Rails command-line interface.generate
: Signals the initiation of a file creation.migration
: Specifies that a migration file should be generated.AddCategoryToPost
: This descriptive name indicates the change being made, which helps maintain clarity in migration history.category:string
: Indicates that a new column,category
, of typestring
, will be added to theposts
table.
Example Output:
The command creates a migration file that can be reviewed, edited if necessary, and then run to alter the database schema:
create db/migrate/20231010123556_add_category_to_post.rb
Use case 5: Generate a scaffold for a model named Post, predefining the attributes title and body
Code:
rails generate scaffold Post title:string body:text
Motivation:
Scaffolding is a powerful Rails feature that sets up an entire framework for basic operations around a model. When models require full CRUD functionality, the scaffold generator quickly builds out a complete set of MVC components and routes, which is invaluable for rapid prototyping and iterative development processes.
Explanation:
rails
: Uses the command suite provided by Ruby on Rails.generate
: Engages the generator function to create necessary components.scaffold
: This directive initiates a comprehensive creation of the model, controller, views, and more, based on specified attributes.Post
: Indicates the name of the model and related resources to be scaffolded.title:string body:text
: Defines two attributes,title
andbody
, for the model, establishing columns in the database and form fields in views.
Example Output:
The scaffold generator will produce a substantial amount of code, creating everything from model files to views and even setting up RESTful routes within the application:
invoke active_record
create db/migrate/20231010123656_create_posts.rb
create app/models/post.rb
invoke test_unit
create test/models/post_test.rb
create test/fixtures/posts.yml
invoke resource_route
route resources :posts
invoke scaffold_controller
create app/controllers/posts_controller.rb
invoke erb
create app/views/posts
create app/views/posts/index.html.erb
create app/views/posts/edit.html.erb
create app/views/posts/show.html.erb
create app/views/posts/new.html.erb
create app/views/posts/_form.html.erb
invoke test_unit
create test/controllers/posts_controller_test.rb
invoke helper
create app/helpers/posts_helper.rb
invoke jbuilder
create app/views/posts/index.json.jbuilder
create app/views/posts/show.json.jbuilder
invoke assets
invoke scss
create app/assets/stylesheets/posts.scss
invoke scss
create app/assets/stylesheets/scaffolds.scss
Conclusion:
The rails generate
command is an essential tool in every Rails developer’s toolkit, significantly enhancing efficiency by automating the creation of common components within a Rails application. By understanding each of its use cases, developers can leverage Rails’ powerful convention-over-configuration philosophy, developing robust applications faster and with fewer errors. Each command serves a unique purpose, aiding in the structuring and scaling of applications by streamlining repetitive tasks and promoting best practices through generated code.