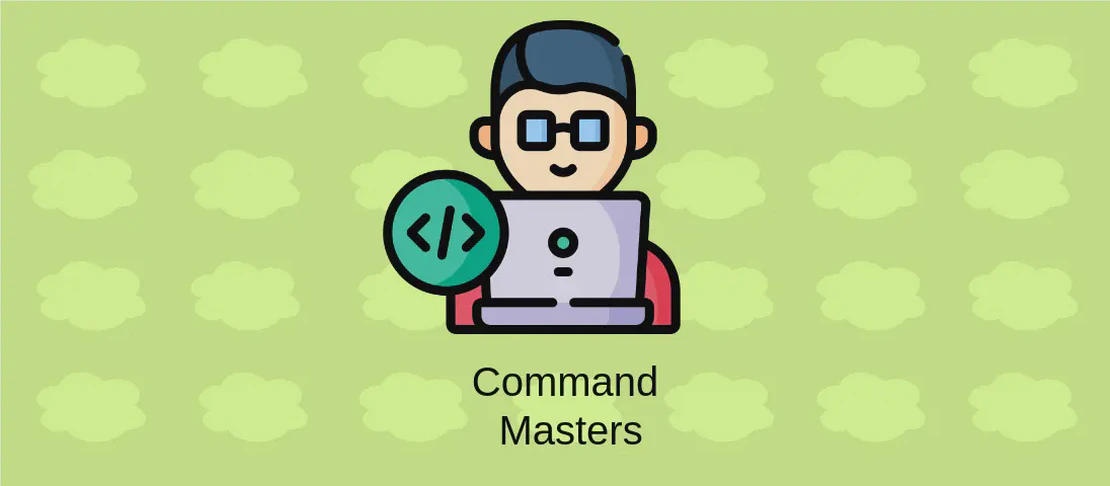
Exploring 'rails routes' in Rails Applications (with examples)
The rails routes
command in Ruby on Rails is a powerful tool for web developers. This command allows them to easily list, format, and match routes within a Rails application, providing invaluable insights into how different parts of the application interact. By leveraging this command, you can streamline your development workflow by quickly identifying routes, understanding routing configurations, and debugging issues. Below, we explore different use cases of this command with detailed examples and explanations.
Use case 1: List all routes
Code:
rails routes
Motivation:
Listing all routes in a Rails application is crucial for developers to understand which endpoints are available, how they are structured, and how they interact with controllers and views. This is especially useful during development or maintenance phases, allowing developers to verify that routes are set up correctly and identify any missing or incorrect routes.
Explanation:
rails
: This is the command-line tool for Ruby on Rails, used to execute various Rails tasks.routes
: This subcommand is used to display the routing information of the application.
Example Output:
Prefix Verb URI Pattern Controller#Action
root GET / pages#home
posts GET /posts(.:format) posts#index
POST /posts(.:format) posts#create
new_post GET /posts/new(.:format) posts#new
Use case 2: List all routes in an expanded format
Code:
rails routes --expanded
Motivation:
Listing routes in an expanded format is beneficial for developers aiming to gain more insights into how path parameters and requirements are structured in each route. This detailed view can help debug complex routing issues, provide clarity when dealing with nested routes, and offer a comprehensive understanding of routing constraints and options.
Explanation:
rails
: Executes Rails commands.routes
: Invokes the display of routing information.--expanded
: This option presents the routes in an expanded mode, displaying more detailed information such as the route name, HTTP verb, URI pattern, controller, action, and the ability to see additional constraints or defaults.
Example Output:
Prefix Verb URI Pattern Controller#Action
root GET / pages#home
posts_index GET /posts(.:format) posts#index
posts POST /posts(.:format) posts#create
new_post GET /posts/new(.:format) posts#new
Use case 3: List routes partially matching URL helper method name, HTTP verb, or URL path
Code:
rails routes -g posts_path|GET|/posts
Motivation:
Filtering routes using partial matches, whether by URL helper method name, HTTP verb, or URL path, is beneficial when dealing with large applications where hundreds of routes may exist. This targeted approach allows developers to quickly find the specific route they need to inspect or debug, drastically improving efficiency and focus when dealing with complex routing issues.
Explanation:
rails
: The Rails command-line tool.routes
: Initiates the route listing.-g
: The-g
(short for –grep) flag allows for filtering routes using a regular expression pattern match.posts_path|GET|/posts
: A regex pattern to match any routes involving either the URL helperposts_path
, the verbGET
, or the path/posts
.
Example Output:
Prefix Verb URI Pattern Controller#Action
posts GET /posts posts#index
Use case 4: List routes that map to a specified controller
Code:
rails routes -c posts|Posts|Blogs::PostsController
Motivation:
Listing routes that map to a specified controller aids developers in understanding which actions within a particular controller are exposed through routes. This insight is crucial when adjusting controller logic or when refactoring application structure, allowing developers to ensure all necessary routes are preserved and operational after changes.
Explanation:
rails
: The command-line interface for Rails.routes
: Displays routing information.-c
: (short for –controller) is used to filter routes associated with a particular controller or set of controllers.posts|Posts|Blogs::PostsController
: Represents a regular expression that matches controllers namedposts
,Posts
, or nested controllers underBlogs
likePostsController
.
Example Output:
Prefix Verb URI Pattern Controller#Action
posts GET /posts(.:format) posts#index
POST /posts(.:format) posts#create
Conclusion
The rails routes
command provides a crucial view into the configuration and structure of a Rails application’s routing system. By allowing developers to list routes in detail, filter based on specific criteria, and align routes with controllers, it enhances productivity and eases maintenance tasks. These use cases demonstrate not just the flexibility of the tool but also its importance in effective Rails application development and management.