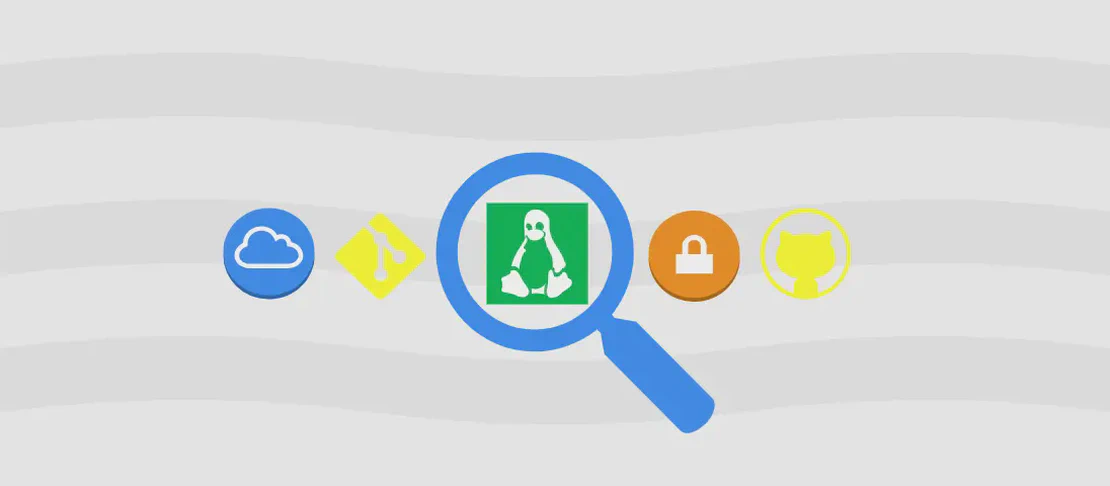
How to Use the Command 'read' in Shell Scripting (with Examples)
The read
command is a fundamental component in shell scripting, designed to retrieve input from the standard input (stdin). This command facilitates user interaction, data manipulation, and real-time data processing, allowing scripts to become dynamic and responsive to user data. The versatility of read
lies in its ability to handle standard input and map the data into variables or arrays, followed by specified manipulation.
Use case 1: Store data that you type from the keyboard
Code:
read variable
Motivation:
In many scripting scenarios, it becomes essential to interact with users to obtain input that can drive the script’s logic. This command provides a straightforward way to capture user input and store it into a variable for subsequent use, such as configuration settings or dynamic content.
Explanation:
read
: Invokes the command to read from the standard input.variable
: The name of the variable where the user’s input is stored.
Example Output:
$ read variable
Hello
$ echo $variable
Hello
Use case 2: Store each of the next lines you enter as values of an array
Code:
read -a array
Motivation:
Sometimes, you need to gather multiple pieces of input at once and manipulate them collectively. By storing these inputs in an array, batch processing can be efficiently executed, which is particularly useful in data analysis or listing files.
Explanation:
read
: Reads input from the standard input.-a
: Flag indicating the input should be stored as elements of an array.array
: The name of the array variable to store the input.
Example Output:
$ read -a array
one two three
$ echo ${array[0]} ${array[1]} ${array[2]}
one two three
Use case 3: Specify the number of maximum characters to be read
Code:
read -n character_count variable
Motivation:
There are occasions when it’s necessary to restrict the number of characters a user can input, such as in password settings or any field with a character limit. This functionality can ensure data integrity and simplify validation processes.
Explanation:
read
: The command to initiate input reading.-n
: Option specifying the number of characters to read.character_count
: The maximum number of characters allowed.variable
: The variable to hold the limited input data.
Example Output:
$ read -n 5 variable
HelloWorld
$ echo $variable
Hello
Use case 4: Assign multiple values to multiple variables
Code:
read _ variable1 _ variable2 <<< "The surname is Bond"
Motivation:
During data processing, it is often advantageous to deconstruct a structured string into distinct components for individual manipulation, such as in data parsing or log file analysis. Using read in this way simplifies extracting specific segments.
Explanation:
read
: Initiates the input reading process._
: Placeholder for data not intended for storage, allowing bypass of unwanted parts.variable1
,variable2
: Variables intended to store particular segments of input data.<<<
: Here-string, feeding a string toread
as if it were inputted from stdin.
Example Output:
$ echo "$variable1 $variable2"
surname Bond
Use case 5: Do not let backslash (\) act as an escape character
Code:
read -r variable
Motivation:
In scripts where raw input is critical, preserving backslashes is essential to maintaining the integrity of file paths or regex patterns. This command variation ensures every character is read literally without interpretation, supporting robust data handling.
Explanation:
read
: Triggers the input reading.-r
: Prevents backslashes from being interpreted as escape characters.variable
: The variable designed to store the unaltered input data.
Example Output:
$ read -r variable
path\to\directory
$ echo $variable
path\to\directory
Use case 6: Display a prompt before the input
Code:
read -p "Enter your input here: " variable
Motivation:
User interaction can be significantly enhanced through prompts, providing clarity on expected input. This approach is critical in user-centric scripts where guidance improves the user experience and reduces errors.
Explanation:
read
: Initiates the read process.-p
: Provides a prompt message to the user before reading input."Enter your input here: "
: The prompt message displayed to the user, guiding input.variable
: Where the prompted user input is stored.
Example Output:
Enter your input here: John Doe
Use case 7: Do not echo typed characters (silent mode)
Code:
read -s variable
Motivation:
Security-sensitive operations, such as password entry, require input concealment to ensure privacy. This function suppresses the display of entered characters, protecting confidential information from potential onlookers.
Explanation:
read
: Undertakes the input reading process.-s
: Suppresses the terminal echo of user input for confidentiality.variable
: The variable that keeps the sensitive input secure.
Example Output:
$ read -s variable
$ echo $variable
secret
Use case 8: Read stdin
and perform an action on every line
Code:
while read line; do echo|ls|rm|... "$line"; done < /dev/stdin|path/to/file|...
Motivation:
Automating repetitive tasks such as processing text files requires reading input line-by-line to perform actions. This construct allows efficient iteration and processing in scripts, perfect for log handling and batch processing.
Explanation:
while read line
: Begins a loop reading each line into the variableline
.do ... "$line"
: Executes actions for each input line, passing it as an argument.done < /dev/stdin|path/to/file
: Indicates where to read the input from, being typically a file or stdin, providing an input stream for processing.
Example Output:
Log entry 1
Processed
Log entry 2
Processed
Conclusion:
Understanding and employing the read
command is essential for shell scripting proficiency. Its varied use cases adapt to diverse needs, from simple user inputs to complex file manipulations, allowing scripts to gain functionality, efficiency, and security. By mastering these techniques, developers can create more interactive and process-intelligent scripts.