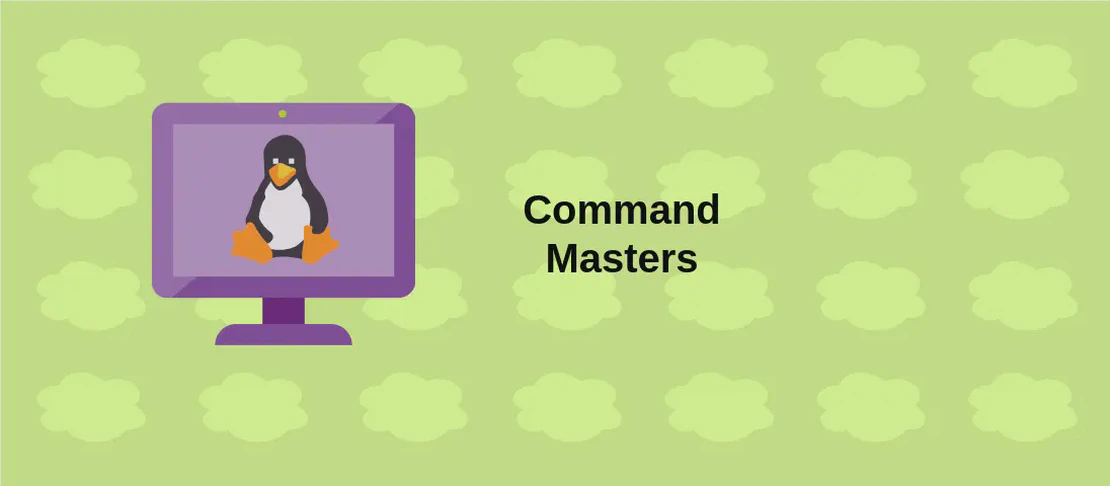
Shell Command "read" (with examples)
- Linux
- November 5, 2023
The read
command is a shell builtin that allows you to retrieve data from stdin
, which is usually input from the keyboard. It is a versatile command that can be used in various ways to capture user input, process data, and perform actions on each line read.
1: Storing Data from Keyboard Input
read variable
Motivation: This use case is useful when you need to prompt the user for input and store it in a shell script for further processing. It allows you to interact with the user and capture their response.
Explanation: The read
command takes a user input and assigns it to the variable
specified. The input is read until a newline character is encountered.
Example Output:
$ read name
John Doe
$ echo "Hello, $name!"
Hello, John Doe!
In this example, the user enters their name, and it is stored in the name
variable. The script then echoes a personalized greeting using the entered name.
2: Storing Lines as Array Elements
read -a array
Motivation: When you want to process multiple inputs and store them as separate elements of an array, this use case is helpful. It allows you to quickly read multiple lines of input and access them individually.
Explanation: The -a
flag tells read
to store each subsequent line of input in an array called array
.
Example Output:
$ read -a numbers
10
20
30
$ echo "${numbers[0]}"
10
$ echo "${numbers[1]}"
20
$ echo "${numbers[2]}"
30
Here, the script prompts the user for three numbers, and each number is stored in a separate element of the numbers
array. The script then echoes each element of the array individually.
3: Specifying the Maximum Character Count
read -n character_count variable
Motivation: When you want to limit the number of characters read from input, this use case is useful. It allows you to control the length of the input entered by the user.
Explanation: The -n
flag specifies the maximum number of characters to read before stopping. It appends the input to the variable
specified.
Example Output:
$ read -n 5 code
1a2b3c
$ echo "$code"
1a2b3
In this example, the script prompts the user to enter a code. However, only the first 5 characters are read and stored in the code
variable. The script then echoes the truncated code.
4: Using a Custom Delimiter
read -d new_delimiter variable
Motivation: When you want to use a custom delimiter instead of the default newline character, this use case is handy. It allows you to split the input into multiple values based on the specified delimiter.
Explanation: The -d
flag changes the delimiter used by read
to parse the input. The new_delimiter
argument specifies the custom delimiter to use, and the parsed values are assigned to the variable
.
Example Output:
$ read -d ',' fruits
Apple,Orange,Banana
$ echo "${fruits[0]}"
Apple
$ echo "${fruits[1]}"
Orange
$ echo "${fruits[2]}"
Banana
Here, the user enters a list of fruits separated by commas. The read
command uses the comma delimiter specified by the -d
flag to split the input into separate elements of the fruits
array. The script then echoes each element of the array individually.
5: Disabling Backslash (\) Escape Character
read -r variable
Motivation: When you want to preserve backslashes (\) as literal characters and not interpret them as escape characters, this use case is essential. It allows you to handle input in a more reliable and predictable manner.
Explanation: The -r
flag tells read
to disable the interpretation of backslash escapes. This ensures that backslashes are treated as ordinary characters.
Example Output:
$ read -r path
C:\Program Files
$ echo "$path"
C:\Program Files
In this example, the user enters a file path that includes backslashes. By using the -r
flag, the script preserves the backslashes as literal characters, allowing for correct processing and printing of the entered path.
6: Displaying a Prompt
read -p "Enter your input here: " variable
Motivation: When you want to provide a prompt to the user indicating what kind of input is expected, this use case is helpful. It improves the user experience by providing clear instructions.
Explanation: The -p
flag followed by a prompt message in quotes displays the specified message as a prompt before accepting the input. The input is stored in the variable
specified.
Example Output:
$ read -p "Enter your name: " name
Enter your name: John Doe
$ echo "Hello, $name!"
Hello, John Doe!
Here, the prompt message “Enter your name: " is displayed before the user enters their name. The name is then stored in the name
variable, and the script echoes a personalized greeting using the entered name.
7: Silent Mode - Suppressing Typed Characters
read -s variable
Motivation: When you want to accept sensitive information, such as passwords, without displaying the typed characters, this use case is vital. It ensures that the input remains hidden from view.
Explanation: The -s
flag tells read
to operate in silent mode, where the typed characters are not echoed to the screen. This is commonly used for password inputs to maintain security.
Example Output:
$ read -s password
Enter your password: *******
$ echo "Password: $password"
Password: *******
In this example, the user enters their password, but the characters they type are not echoed to the screen. The password is stored in the password
variable, and the script echoes a masked representation of the password for verification.
8: Reading stdin
and Performing an Action on Each Line
while read line; do
echo "$line"
done
Motivation: When you want to process each line of input read from stdin
and perform specific actions on them, this use case is valuable. It enables you to work with input streams containing multiple lines.
Explanation: The while read line
syntax reads each line from stdin
and assigns it to the line
variable. The code block between do
and done
is then executed for each line read, allowing you to perform custom actions.
Example Output:
$ cat file.txt
Hello
World
$ cat file.txt | while read line; do
echo "$line!"
done
Hello!
World!
In this example, the contents of a file named “file.txt” are piped to stdin
. The while read line
loop reads each line from the input stream, and the script echoes the line with an exclamation mark appended. Each line from the file is processed and printed with the added exclamation mark.