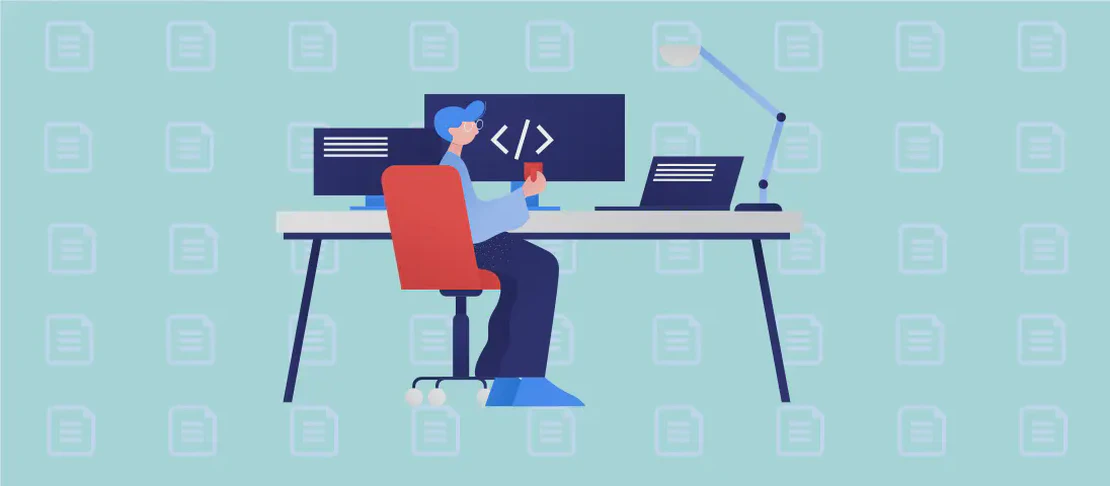
Understanding the 'readonly' Command in Shell Scripting (with examples)
The readonly
command is a powerful utility in shell scripting used to set shell variables as immutable. Once a variable has been set as read-only, its value cannot be changed or unset during the lifetime of the shell. This characteristic is particularly useful for maintaining the integrity of certain critical variables whose values should not be altered accidentally or intentionally during script execution.
Setting a Read-Only Variable
Code:
readonly variable_name=value
Motivation:
In shell scripting, there might be scenarios where a variable holds critical configuration or settings that should remain constant throughout the script’s execution. For example, setting a constant API endpoint in a script that performs data fetching tasks. By making this variable read-only, you ensure that inadvertent changes do not occur, which could potentially result in faulty execution or security vulnerabilities.
Explanation:
readonly
: This is the command indicating that the following variable will be immutable.variable_name
: This refers to the name of the variable you want to make read-only. It acts as an identifier that will hold a specific value.value
: This is the content or value that you assign to thevariable_name
. This assignment happens at the time the variable is declared as read-only.
Example Output:
Setting the variable API_ENDPOINT
to a constant value and then attempting to change it will result in an error indicating that the variable is read-only.
$ readonly API_ENDPOINT="https://api.example.com"
$ API_ENDPOINT="https://api.changed.com"
-bash: API_ENDPOINT: readonly variable
Marking an Existing Variable as Read-Only
Code:
readonly existing_variable
Motivation:
In cases where you have already defined a variable and possibly used it several times in your script, but realize that it should remain unchanged henceforth, marking it as read-only can prevent any further modifications. This is especially relevant in collaborative environments, where different developers might modify shared scripts, potentially altering crucial values unintentionally.
Explanation:
readonly
: The command used to set the specified variable as immutable.existing_variable
: The variable name that has been previously defined and is now being marked as read-only to ensure its value is not altered.
Example Output:
Assume DATA_PATH
has been previously set. Trying to modify it after marking it read-only results in an error.
$ DATA_PATH="/var/data"
$ readonly DATA_PATH
$ DATA_PATH="/new/data/path"
-bash: DATA_PATH: readonly variable
Printing the Names and Values of All Read-Only Variables
Code:
readonly -p
Motivation:
While working with scripts, especially in debugging or reviewing processes, it becomes essential to have a clear overview of all the variables that have been set to read-only. With many variables in a complex script, listing all the immutable ones quickly can help in understanding the constants present and how the script architecture is intended to function.
Explanation:
readonly
: The base command for creating or managing read-only variables.-p
: The flag used to trigger the printing of all read-only variables, displaying their names and current values.
Example Output:
Executing the command will show a list of variables that are currently set as read-only, along with their assigned values.
$ readonly -p
declare -r API_ENDPOINT="https://api.example.com"
declare -r DATA_PATH="/var/data"
Conclusion:
The readonly
command provides essential control over variable immutability in shell scripting, ensuring critical script values remain consistent and secure. By setting variables as read-only, marking existing ones for the same, and viewing all immutable variables, developers can ensure greater stability and protection against unintended or unauthorized changes.