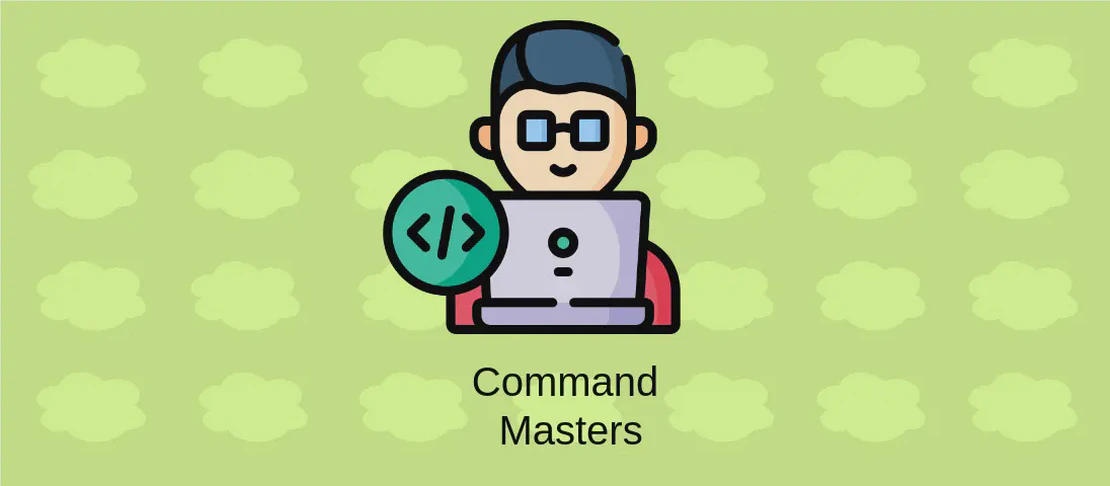
How to Use the PowerShell Command 'Remove-Item' (with examples)
- Windows
- December 17, 2024
The ‘Remove-Item’ command in PowerShell is a versatile command used for deleting files, folders, and even registry keys and subkeys in Windows operating systems. This command is particularly powerful because it allows for the removal of items with specific attributes, supports interactive removal, and offers a recursive deletion ability. Understanding how to use ‘Remove-Item’ effectively can be highly beneficial for system administrators and power users who often need to manage files and system configurations.
Use case 1: Removing Specific Files or Registry Keys (without Subkeys)
Code:
Remove-Item path\to\file_or_key1 , path\to\file_or_key2
Motivation:
This use case is particularly useful when you need to quickly and efficiently delete specific files or registry keys without worrying about any subkeys. For instance, if you are cleaning up a set of temporary files or deleting specific registry entries as part of a software uninstallation process.
Explanation:
Remove-Item
: The command used to delete files, folders, or registry keys.path\to\file_or_key1 , path\to\file_or_key2
: The paths to the specific files or registry keys that you wish to remove. You can specify multiple items by separating them with commas.
Example Output:
No output is returned when a file is successfully deleted, but if an error occurs, such as if a file is not found, PowerShell will return an error message indicating the issue.
Use case 2: Removing Hidden or Read-Only Files
Code:
Remove-Item -Force path\to\file1 , path\to\file2
Motivation:
Sometimes, files or directories are hidden or set to read-only, making them resistant to standard delete operations. This scenario often arises when dealing with system files or files from installed applications. Using the -Force
parameter allows these files to be deleted regardless of their attributes.
Explanation:
-Force
: This parameter is used to forcefully remove items, ignoring any read-only or hidden attributes.path\to\file1 , path\to\file2
: Specifies the paths to the files you want to delete forcefully.
Example Output:
Upon execution, the files are deleted without any prompt or output, unless there is an error (e.g., if the file is in use). Any errors encountered will be outputted to the console.
Use case 3: Removing Items with Interactive Confirmation
Code:
Remove-Item -Confirm path\to\file_or_key1 , path\to\file_or_key2
Motivation:
When there is uncertainty about whether to delete certain files or registry keys, interactive confirmation ensures that each deletion is intentional. This is especially valuable when making changes that might affect system stability or data integrity.
Explanation:
-Confirm
: Triggers a prompt before each item is deleted, asking for user confirmation.path\to\file_or_key1 , path\to\file_or_key2
: The paths to the files or keys for which confirmation is needed before deletion.
Example Output:
Before each item is deleted, PowerShell will prompt: Are you sure you want to perform this action?
followed by details of the item. You must input Y
or N
to proceed or cancel.
Use case 4: Recursive Deletion of Files and Directories
Code:
Remove-Item -Recurse path\to\file_or_directory1 , path\to\file_or_directory2
Motivation:
In situations where entire directories and their contents need to be removed—such as clearing out old log directories or removing a directory structure from a project—recursive deletion provides an efficient way to achieve this in one command.
Explanation:
-Recurse
: Enables removal of directories and all their contents, working through all subdirectories recursively.path\to\file_or_directory1 , path\to\file_or_directory2
: The paths to files or directories to be recursively deleted.
Example Output:
The specified directories and their contents are deleted, with no output unless errors occur. Errors will be displayed if a file is locked or if there are permission issues.
Use case 5: Removing Registry Keys and All Subkeys
Code:
Remove-Item -Recurse path\to\key1 , path\to\key2
Motivation:
When completely removing registry keys and their subkeys, such as during a thorough removal of a software application, recursive deletion ensures all related configuration is cleared without leaving residual entries.
Explanation:
-Recurse
: This allows for the removal of the specified registry key and all its subkeys, ensuring a comprehensive deletion.path\to\key1 , path\to\key2
: The registry key paths to be deleted.
Example Output:
The specified keys and subkeys are deleted without additional output, unless there is an error—such as insufficient permissions—which will then display relevant error messages.
Use case 6: Performing a Dry Run of the Deletion Process
Code:
Remove-Item -WhatIf path\to\file1 , path\to\file2
Motivation:
Before executing potentially destructive operations, it’s prudent to verify what the impact will be. The -WhatIf
parameter simulates the command’s effects without making actual changes, thereby providing a preview of the command’s impact on the system.
Explanation:
-WhatIf
: This parameter tells PowerShell to show what would happen if the command were executed, without making any changes.path\to\file1 , path\to\file2
: The paths for files or directories you’re considering for deletion.
Example Output:
PowerShell will display messages describing what actions would have occurred, such as “What if: Removing file path\to\file1,” confirming the potential actions without actual deletion.
Conclusion:
The ‘Remove-Item’ command is remarkably flexible, catering to a variety of file and registry management needs. By comprehensively understanding each use case—from basic item removal to more complex scenarios like recursive or forced deletions—users can safely and effectively manage their Windows environment. Whether you need to ensure proper confirmations, preview the impact of deletions, or manage hidden and read-only files, ‘Remove-Item’ provides the necessary tools to tailor the command to your specific needs.