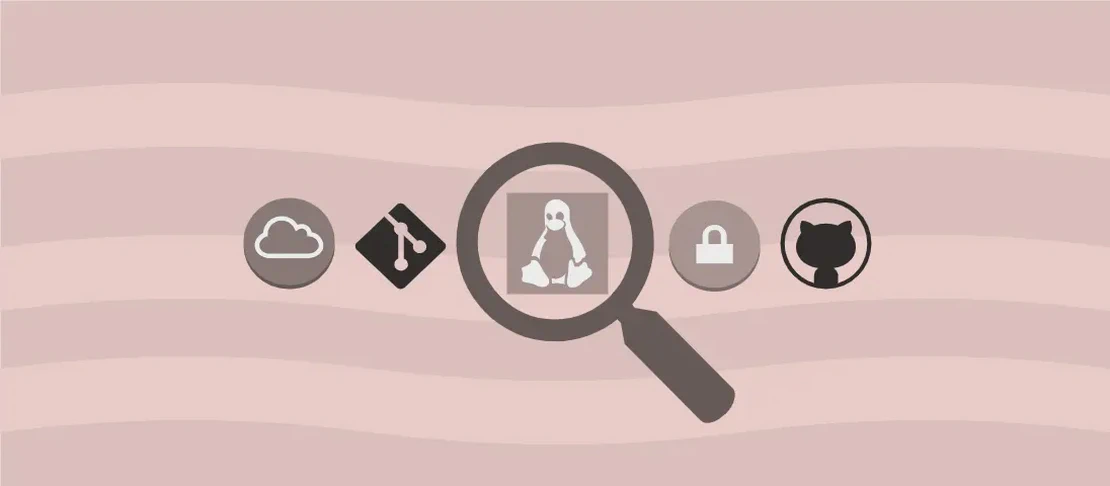
How to use the command 'rspec' (with examples)
RSpec is a robust behavior-driven development (BDD) testing framework for Ruby. It is utilized to write clear and expressive tests for Ruby code, promoting better software design and function. RSpec allows developers to create comprehensive test suites, which contribute significantly to the reliability and maintainability of applications by identifying bugs early in the development process.
Use case 1: Initialize an .rspec configuration and a spec helper file
Code:
rspec --init
Motivation:
Initializing an RSpec configuration is the first step when you set up a new testing environment for your Ruby project. By doing so, you create an organized framework that will help manage and run your test cases efficiently. The .rspec
configuration file and spec/spec_helper.rb
are critical components; they store various default settings and helper methods that are needed across all your tests.
Explanation:
rspec
: The base command used to interact with the RSpec framework.--init
: This flag tells RSpec to set up an initial configuration in your project directory. It generates the necessary configuration files which help lay the groundwork for writing and organizing test cases.
Example Output:
create .rspec
create spec/spec_helper.rb
Use case 2: Run all tests
Code:
rspec
Motivation:
Running all tests in a project is a crucial step in the development cycle to ensure that recent changes have not inadvertently broken anything elsewhere in your codebase. This comprehensive approach helps maintain a stable application by ensuring that existing features work as expected.
Explanation:
rspec
: When used alone, this command runs all test files found in the standardspec
directory of your project. It’s straightforward and effective for a full test suite evaluation.
Example Output:
....
Finished in 0.032 seconds (files took 0.086 seconds to load)
4 examples, 0 failures
Use case 3: Run a specific directory of tests
Code:
rspec path/to/directory
Motivation:
You might want to run tests for a specific module or feature without executing the entire suite, especially when working in a large codebase where running all tests could be time-consuming. This use case allows for focused testing, significantly speeding up the feedback process during development.
Explanation:
rspec
: The command to invoke the testing process.path/to/directory
: Replace this with the actual path to the directory containing the subset of tests you wish to run. This directs RSpec to only execute the tests found in the specified directory.
Example Output:
..
Finished in 0.005 seconds (files took 0.018 seconds to load)
2 examples, 0 failures
Use case 4: Run one or more test files
Code:
rspec path/to/file1 path/to/file2 ...
Motivation:
Sometimes, you need to run individual test files, for instance, when you are working on or debugging particular test cases. By specifying files directly, you save time focusing only on relevant parts of your test suite.
Explanation:
rspec
: Invokes the RSpec testing framework.path/to/file1 path/to/file2 ...
: These are paths to the specific test files you want to run. RSpec will execute the tests contained within these files.
Example Output:
.
Finished in 0.003 seconds (files took 0.012 seconds to load)
1 example, 0 failures
Use case 5: Run a specific test in a file
Code:
rspec path/to/file:83
Motivation:
There are scenarios where you need to quickly verify or debug a specific test, especially during development or when a particular test is failing. Running a single example from a file provides immediate feedback without the overhead of running all tests.
Explanation:
rspec
: The command to run test cases.path/to/file
: The file containing the test.:83
: This specifies the line number where the test you want to run is defined. RSpec locates and executes the test starting at this line.
Example Output:
.
Finished in 0.002 seconds (files took 0.010 seconds to load)
1 example, 0 failures
Use case 6: Run specs with a specific seed
Code:
rspec --seed seed_number
Motivation:
Using a specific seed allows you to reproduce the exact order of test execution from a previous run, which is useful for debugging purposes. If tests fail only under certain conditions or sequences, the seed provides a way to consistently recreate that environment, enabling more effective problem diagnosis.
Explanation:
rspec
: Base command to run the tests.--seed
: Flag to specify the seed value.seed_number
: A numerical value that initializes RSpec’s pseudo-random number generator, ensuring that the test order remains consistent across runs with the same seed.
Example Output:
...
Randomized with seed 12345
Finished in 0.043 seconds (files took 0.089 seconds to load)
3 examples, 1 failure
Failed examples:
rspec ./path/to/failing/spec.rb:42 # Example description
Conclusion:
Understanding how to effectively use the RSpec command line options can greatly enhance your workflow when testing Ruby applications. Whether you’re configuring a new project with rspec --init
, focusing on specific tests or test files, or needing to re-run tests with a particular order, these examples illustrate powerful techniques that enable efficient and comprehensive software testing.