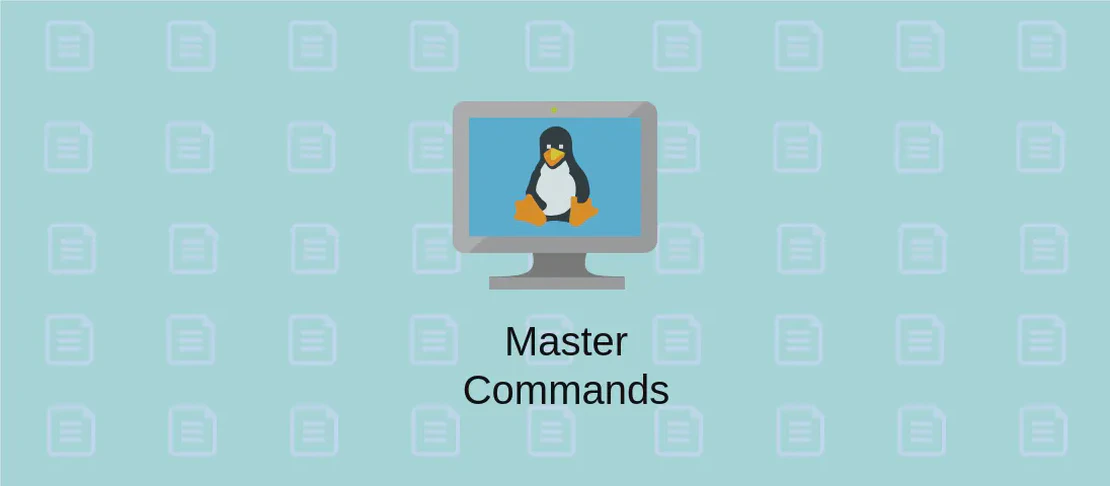
How to Use the Command 'rubocop' (with examples)
Rubocop is a popular tool in the Ruby community, designed for code linting and formatting. It ensures that Ruby files adhere to a set of style guidelines known as ‘cops.’ This command-line tool not only checks code against these guidelines but can also auto-correct detected issues. Rubocop helps developers maintain a consistent codebase, making it easier to read and reducing potential errors.
Use case 1: Check All Files in the Current Directory (Including Subdirectories)
Code:
rubocop
Motivation:
You might want to perform a comprehensive code quality check throughout your entire project. Running rubocop
without any additional arguments checks every Ruby file in the current directory and its subdirectories. It’s a quick way to catch style violations or issues in your codebase.
Explanation:
rubocop
: This command by itself scans all.rb
files in the current directory and any files located in subdirectories. It’s the default operation of Rubocop, meant for situations where you want a thorough review of your project.
Example Output:
Inspecting 5 files
.CC..
Offenses:
file1.rb:3:1: C: Layout/IndentationConsistency: Inconsistent indentation detected.
file2.rb:7:9: C: Naming/VariableName: Use snake_case for variable names.
5 files inspected, 2 offenses detected
Use case 2: Check One or More Specific Files or Directories
Code:
rubocop path/to/file_or_directory1 path/to/file_or_directory2 ...
Motivation:
You may have made recent changes to certain files or directories and wish to limit your check to those specific areas rather than your entire codebase. This use case is ideal for quickly validating changes before committing them to a shared code repository.
Explanation:
rubocop
: Initiates the linting process.path/to/file_or_directory1
: Specifies the path of the file or directory you intend to check. You can add multiple paths if you want to check more than one specific area.
Example Output:
Inspecting 3 files
..C
Offenses:
path/to/file1.rb:10:3: C: Layout/LineLength: Line is too long. [105/80]
3 files inspected, 1 offense detected
Use case 3: Write Output to File
Code:
rubocop --out path/to/file
Motivation:
In some scenarios, you might need to document the results of your Rubocop run, either for future reference or to share with team members. Directing the output to a file provides a tangible record of your code quality at a specific point in time.
Explanation:
rubocop
: Initiates the linting process.--out
: Directs the output to a file instead of the console.path/to/file
: Specifies the file path where the output will be saved, allowing you to review or share the results whenever necessary.
Example Output:
No console output since the results are saved in the specified file. However, if you open the specified file, it may contain:
Inspecting 5 files
....C
Offenses:
path/to/file2.rb:4:5: C: Metrics/MethodLength: Method has too many lines. [22/10]
5 files inspected, 1 offense detected
Use case 4: View List of Cops (Linter Rules)
Code:
rubocop --show-cops
Motivation:
Understanding the rules that Rubocop enforces is paramount for aligning your code with its style guidelines. Viewing the list of cops helps you know exactly what styling expectations exist, allowing you to adhere to or customize those rules accordingly.
Explanation:
rubocop
: Starts the Rubocop command.--show-cops
: Displays a complete list of style checks (‘cops’) that Rubocop can enforce. This information is valuable for tailoring the linter to your project’s specific needs.
Example Output:
# Supports multiple test patterns
Layout/AccessModifierIndentation:
Description: 'Checks the indentation of protected, private.'
Enabled: true
Lint/AmbiguousBlockAssociation:
Description: 'Checks for ambiguous block association...'
Enabled: true
Use case 5: Exclude a Cop
Code:
rubocop --except cop1 cop2 ...
Motivation:
There might be specific styling rules that you or your team do not wish to enforce. For such cases, excluding certain cops from the Rubocop check allows you to bypass those rules without affecting the rest of the linting process.
Explanation:
rubocop
: Initiates the lint process.--except
: Tells Rubocop to ignore the specified cops.cop1 cop2 ...
: Represents the cops you want to exclude from your linting. Each cop has an identifier, like ‘Metrics/MethodLength,’ which you use to exempt it.
Example Output:
Inspecting 4 files
....
4 files inspected, no offenses detected
Use case 6: Run Only Specified Cops
Code:
rubocop --only cop1 cop2 ...
Motivation:
If you want to focus on particular areas of your code, running only specific cops can make your audit more efficient. This is beneficial for phased code reviews where you might handle different group rules at different times.
Explanation:
rubocop
: Begins the Rubocop linting process.--only
: Instructs Rubocop to enforce only the specified cops.cop1 cop2 ...
: Lists the specific cops you wish to run, providing focused output on particular styling issues.
Example Output:
Inspecting 2 files
.C
Offenses:
file3.rb:8:1: C: Metrics/CyclomaticComplexity: Cyclomatic complexity for method is too high.
2 files inspected, 1 offense detected
Use case 7: Auto-correct Files (Experimental)
Code:
rubocop --auto-correct
Motivation:
When you want to quickly fix minor style violations, the auto-correct feature is incredibly handy. Though still experimental, it saves time by automatically correcting some typical issues according to the defined rules.
Explanation:
rubocop
: Initiates the linting operation.--auto-correct
: Triggers the automatic correction feature, where Rubocop attempts to fix offenses for specific, minor issues.
Example Output:
Inspecting 6 files
...... (auto-corrected some offenses)
6 files inspected, no offenses remaining
Conclusion:
Rubocop is a versatile tool for Ruby programmers, offering multiple modes for code inspection and correction. Whether you are managing an entire project or just a few files, Rubocop ensures your code remains clean, readable, and consistent with your desired style guidelines. By understanding each use case, you can tailor Rubocop’s extensive functionality to suit both personal and team coding practices.