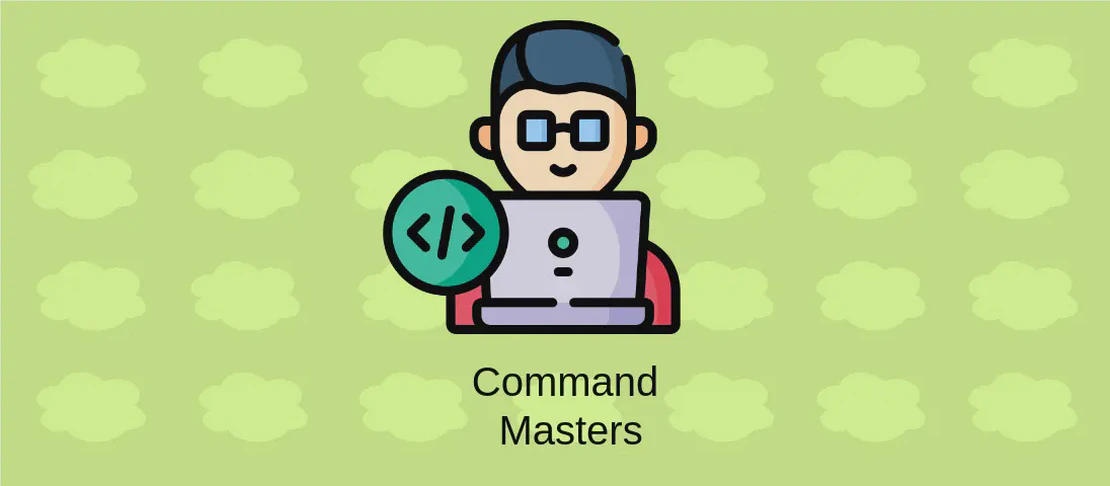
How to use the Ruby Command (with examples)
Ruby is a dynamic, open-source programming language with a focus on simplicity and productivity. Its elegant syntax is natural to read and easy to write. The ruby
command serves as the interpreter for the language, allowing users to execute Ruby scripts, run individual commands, check for syntax errors, start a lightweight HTTP server, and more.
Use case 1: Execute a Ruby script
Code:
ruby script.rb
Motivation:
Executing scripts is one of the core functionalities of any programming language interpreter. With the ruby
command, you can run any Ruby script file, which could contain anything from simple arithmetic operations to complex algorithms and web server mechanisms. This makes Ruby a versatile tool in both development and scripting environments.
Explanation:
ruby
: Invokes the Ruby interpreter.script.rb
: Represents the Ruby script file you wish to execute. The.rb
extension is conventionally used for Ruby script files.
Example Output:
Suppose script.rb
contains:
puts "Hello, World!"
Executing ruby script.rb
would output:
Hello, World!
Use case 2: Execute a single Ruby command in the command-line
Code:
ruby -e 'command'
Motivation:
Sometimes you only need to execute a small snippet or line of Ruby code without the need to create a whole script file. The -e
option allows for quick testing of code snippets directly in the command line, which can be extremely helpful for prototyping code or learning Ruby.
Explanation:
ruby
: Calls the Ruby interpreter.-e
: Allows execution of code provided as an argument.'command'
: The Ruby code you wish to execute. Wrapped in quotes if it contains spaces or special characters.
Example Output:
For the command:
ruby -e 'puts 1 + 1'
The output would be:
2
Use case 3: Check for syntax errors on a given Ruby script
Code:
ruby -c script.rb
Motivation:
Checking for syntax errors is crucial for debugging and development. A syntax check ensures that all the structural elements of your code are correct before execution, helping you catch mistakes early in the development process.
Explanation:
ruby
: Utilizes the Ruby interpreter to perform the action.-c
: Requests a syntax check without executing the script.script.rb
: The Ruby script to be checked for syntax errors.
Example Output:
For a script with no syntax errors:
Syntax OK
If there are errors, it will output descriptive error messages indicating the problems.
Use case 4: Start the built-in HTTP server on port 8080 in the current directory
Code:
ruby -run -e httpd . -p 8080
Motivation:
Sometimes you need to serve files or test web applications locally. With Ruby’s built-in HTTP server, you can quickly spin up a server without any additional configurations, making it easy to share files over a local network or test how your web applications perform in a server-like environment.
Explanation:
ruby
: Starts the Ruby interpreter.-run -e httpd
: Uses Ruby’s-run
library, with-e
specifying thehttpd
command to start an HTTP server..
: Specifies the current directory as the root of the HTTP server.-p 8080
: Specifies port 8080 for the server to listen on. Port numbers can be adjusted as needed.
Example Output:
When the server starts successfully, you might see:
[2023-10-01 14:05:09] INFO WEBrick 1.7.0
[2023-10-01 14:05:09] INFO WEBrick::HTTPServer#start: pid=12345 port=8080
Use case 5: Locally execute a Ruby binary without installing the required library it depends on
Code:
ruby -I path/to/library_folder -r library_require_name path/to/bin_folder/bin_name
Motivation:
When dealing with Ruby binaries that have specific library dependencies, it can be cumbersome to install all required libraries system-wide. This command allows developers to specify a library load path and require any necessary library during execution, providing flexibility when testing or using specific versions.
Explanation:
ruby
: Starts the Ruby interpreter.-I path/to/library_folder
: Adds the specified library path to Ruby’s load path.-r library_require_name
: Requires the specified library explicitly. This makes the library available for the execution of the binary.path/to/bin_folder/bin_name
: Executes the specified Ruby binary located in a given folder.
Example Output:
The exact output depends on the execution and function of the specific binary being run.
Use case 6: Display Ruby version
Code:
ruby -v
Motivation:
Knowing the version of Ruby you’re working with is vital, especially when managing multiple Ruby projects that might require different versions. This command provides a quick way to check which version of Ruby is currently being used, ensuring compatibility and assisting in troubleshooting.
Explanation:
ruby
: Calls the Ruby interpreter.-v
: Requests the version information of the currently installed Ruby interpreter.
Example Output:
ruby 2.7.0 (2019-12-25 revision 647ee6f091) [x86_64-linux]
Conclusion:
The ruby
command provides a powerful yet user-friendly interface for executing a wide array of tasks within the Ruby ecosystem. Whether you’re running scripts, testing small snippets, checking for syntax errors, creating a local server, or verifying your Ruby setup, understanding these use cases can greatly enhance the efficiency and flexibility of your development workflow.