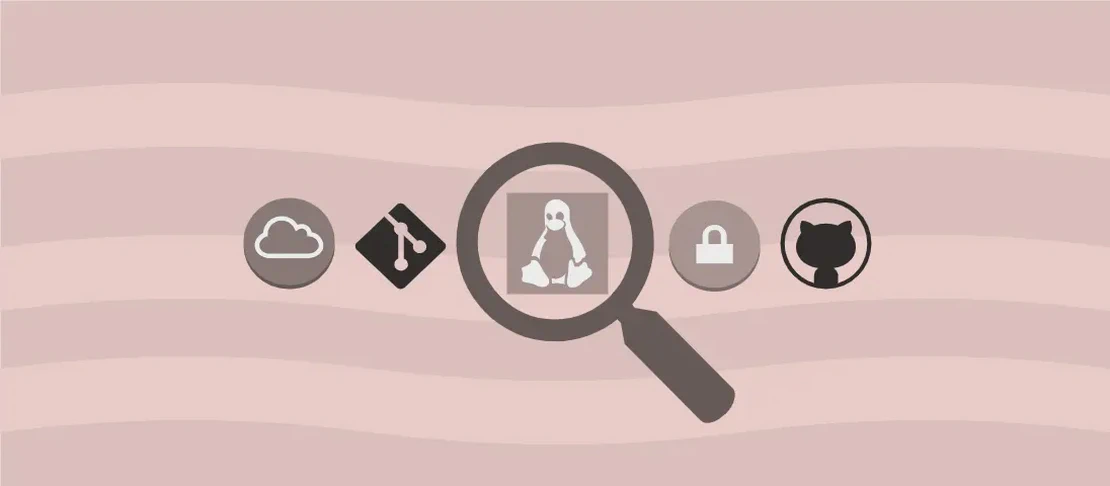
Harnessing the Power of 'ruff check' Command for Python Linting (with examples)
‘Ruff check’ is an extraordinarily fast Python linter designed to enhance code quality by analyzing Python files for potential errors, coding inconsistencies, and style issues. This tool is an indispensable part of the developer’s toolkit for maintaining clean, readable, and efficient code. The command offers a broad array of functionalities including the automatic application of fixes, live linting during code changes, and highly configurable rule settings, making it adaptable to a variety of development needs. Below, we explore several use cases of the ‘ruff check’ command with practical examples and detailed explanations.
Use Case 1: Run the Linter on the Given Files or Directories
Code:
ruff check path/to/file_or_directory1 path/to/file_or_directory2 ...
Motivation:
When working on a project with multiple files and directories, it is vital to identify coding issues across the entire codebase. Running ‘ruff check’ on specific files or directories helps in quickly pinpointing issues that need attention, facilitating a focused code review and improving overall code quality.
Explanation:
ruff check
: Invokes the ruff linter.path/to/file_or_directory1 path/to/file_or_directory2 ...
: These placeholders represent the specific files or directories that you wish to lint. Including one or multiple paths directs ruff to only analyze those specified locations.
Example Output:
Analyzing path/to/file_or_directory1...
No issues detected!
Analyzing path/to/file_or_directory2...
10 issues found:
path/to/file_or_directory2/file.py:2:1: E402 module level import not at top of file
...
Use Case 2: Apply the Suggested Fixes, Modifying the Files In-place
Code:
ruff check --fix
Motivation:
When working on a large codebase with numerous stylistic issues and known errors, manually fixing each issue can be time-consuming. The --fix
option automates this process by applying suggested corrections directly within the files, thus saving both time and effort while ensuring adherence to best coding practices.
Explanation:
ruff check
: Executes the ruff linter.--fix
: This flag instructs ruff to automatically rectify the identified issues within the source files, updating them in real-time.
Example Output:
Applying fixes...
5 files modified.
Use Case 3: Run the Linter and Re-lint on Change
Code:
ruff check --watch
Motivation:
During active development, as code is constantly being written and modified, ensuring continual code quality can be challenging. The --watch
feature allows developers to catch potential issues immediately as code changes, facilitating an agile development process with immediate feedback.
Explanation:
ruff check
: Initiates the linter.--watch
: This option continuously monitors the designated files and directories. When any change is detected, it automatically triggers a fresh linting process.
Example Output:
Watching for file changes...
Linting complete. No issues found.
Detected changes in path/to/file.py...
Re-linting...
2 issues detected:
path/to/file.py:5:10: F841 local variable 'x' is assigned but never used
...
Use Case 4: Only Enable the Specified Rules (or All Rules), Ignoring the Configuration File
Code:
ruff check --select ALL|rule_code1,rule_code2,...
Motivation:
In some scenarios, developers may need to focus only on specific types of issues or enforce certain rules temporarily, overriding any pre-specified configuration settings. The --select
option allows for precise control of which rules are applied, thereby aligning linting results with immediate project requirements.
Explanation:
ruff check
: Runs the ruff command.--select ALL|rule_code1,rule_code2,...
: This parameter enables the specified linting rules. UsingALL
will apply every rule uniformly, but listing specific rule codes restricts the analysis to those rules alone.
Example Output:
Linting with selected rules: E501, F841...
path/to/file.py:6:80: E501 line too long (85 > 79 characters)
path/to/file.py:8:5: F841 local variable 'z' is assigned but never used
Use Case 5: Additionally Enable the Specified Rules
Code:
ruff check --extend-select rule_code1,rule_code2,...
Motivation:
There might be times when the default or configured set of linting rules needs to be expanded with additional checks without discarding existing settings. This option allows the rule set to be extended, offering flexibility to customize lint requirements dynamically as the project’s needs evolve.
Explanation:
ruff check
: Initiates the linting.--extend-select rule_code1,rule_code2,...
: This flag appends additional rule checks to the current set, enhancing the linting scope.
Example Output:
Extending selected rules with: E402...
path/to/another_file.py:1:1: E402 module level import not at top of file
Use Case 6: Disable the Specified Rules
Code:
ruff check --ignore rule_code1,rule_code2,...
Motivation:
In scenarios where specific linting issues are deemed non-critical or irrelevant for a particular project phase, enforcing strict compliance with all rules might be counterproductive. By using the --ignore
option, developers can selectively bypass certain rules, allowing the team to focus on more critical concerns.
Explanation:
ruff check
: Runs the linter.--ignore rule_code1,rule_code2,...
: This parameter deactivates the specified linting rules, preventing them from affecting the lint results.
Example Output:
Ignoring selected rules: W293...
No issues found after ignoring specified rules.
Use Case 7: Ignore All Existing Violations of a Rule by Adding # noqa
Directives
Code:
ruff check --select rule_code --add-noqa
Motivation:
For legacy codebases or temporary exemptions, developers might need to suppress specific rule violations to prevent them from appearing in lint results. The --add-noqa
option automatically inserts # noqa
comments in offending lines, effectively silencing these violations across the codebase.
Explanation:
ruff check
: Executes the linting.--select rule_code
: Specifies the rule to focus on for the adding of# noqa
.--add-noqa
: Instructs ruff to append# noqa
to lines with rule violations, suppressing specified lint errors.
Example Output:
Ignoring rule F841 by adding # noqa...
Modifying path/to/file.py...
All violations suppressed.
Conclusion:
The ‘ruff check’ command provides a versatile and efficient approach to Python code linting, offering developers the tools necessary for maintaining high-quality code. Whether it’s running standard checks, applying automatic fixes, or customizing linting rules, ‘ruff check’ flexibly adapts to a project’s evolving needs. By incorporating these use cases into your development workflow, you can ensure that your code remains clean, compliant, and error-free.