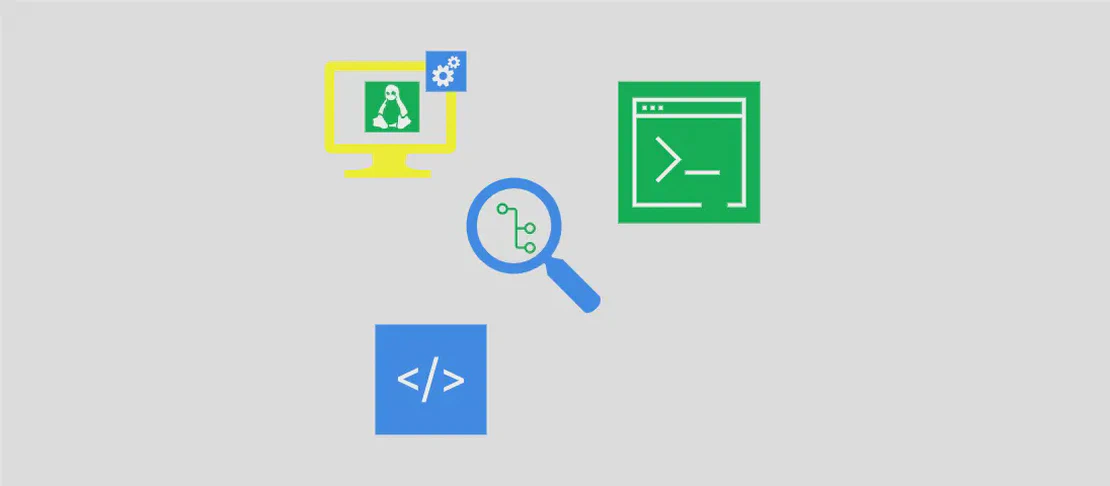
How to Use the Rust Compiler 'rustc' (with examples)
The rustc
command is the Rust compiler that transforms Rust source code into executable binaries. While Rust projects commonly utilize cargo
for building and managing projects, rustc
remains a vital tool for compiling specific source files with custom settings and configurations. This command offers direct interaction with the compilation process, allowing for advanced customization and experimentation.
Use case 1: Compile a binary crate
Code:
rustc path/to/main.rs
Motivation:
Compiling a binary crate directly is essential when you need to quickly compile a standalone Rust source file without building an entire project with Cargo. This use case is particularly useful in learning environments, quick testing scenarios, or when dealing with single-file scripts.
Explanation:
rustc
: The command to invoke the Rust compiler.path/to/main.rs
: This specifies the file path to the Rust file you want to compile. The file should contain amain
function as the entry point of the binary program.
Example Output:
Typically, executing this command will create a binary executable (named after the source file without the extension) in the same directory as the source file. If the file is main.rs
, the output might be ./main
or main.exe
on Windows. Running this binary will execute the Rust program.
Use case 2: Compile with optimizations
Code:
rustc -C lto -C opt-level=s path/to/main.rs
Motivation:
Optimization is crucial for improving the performance and efficiency of compiled binaries, especially in production environments. In this use case, focusing on optimizing for binary size makes it ideal for resource-constrained environments like embedded systems or applications where binary distribution size is crucial.
Explanation:
-C
: Stands for “codegen” options, which modify how the Rust compiler generates the output.lto
: Link Time Optimization, which combines the Rust compiler’s codegen process with link-time analysis to optimize the entire program further.opt-level=s
: This specifies the level of optimization, focusing on reducing the binary size. Thes
level is a balance between performance and binary size, whilez
would reduce size more aggressively.path/to/main.rs
: As before, this is the path to the Rust source file you are compiling.
Example Output:
The optimized binary will be smaller than the default compilation size. This can be validated by using tools like du
or ls
with the -lh
flag to check the file size difference compared to a non-optimized build.
Use case 3: Compile with debugging information
Code:
rustc -g path/to/main.rs
Motivation:
Including debugging information in the compiled binary is invaluable during development. Debugging allows developers to examine the state of the program and diagnose issues more effectively using tools like gdb
or lldb
. This is especially useful when stepping through code line by line or when analyzing core dumps.
Explanation:
-g
: Includes debugging information in the binary output, which allows symbol information to be available when using a debugger.path/to/main.rs
: Specifies the Rust source file path for compilation as before.
Example Output:
The resulting binary will be larger due to the embedded debugging metadata. When run under a debugger, the additional symbol information allows for line-level debugging and the ability to inspect variables, stack frames, and more.
Use case 4: Explain an error message
Code:
rustc --explain E0425
Motivation:
Understanding compiler error messages is crucial for debugging and improving code. Every Rust error message is assigned a unique error code, and the --explain
flag provides detailed information about these errors, aiding developers in understanding and resolving issues more quickly and effectively.
Explanation:
--explain
: Requests a detailed explanation of the specified error code from the Rust compiler.E0425
: An example of a Rust error code, typically corresponding to “cannot find value in this scope.” The error code can be any valid Rust compiler error identifier.
Example Output:
The output will be an extensive explanation of the error E0425, often including possible causes and strategies for resolution. This aids developers in understanding not only what the error means but also how they might address it.
Use case 5: Compile with architecture-specific optimizations
Code:
rustc -C target-cpu=native path/to/main.rs
Motivation:
Compiling with optimizations specific to the architecture of the target machine can lead to performance gains by leveraging CPU-specific instructions and features. This is particularly advantageous when the compiled code is intended for local use, ensuring it runs as efficiently as possible on the given hardware.
Explanation:
-C target-cpu=native
: Directs the compiler to optimize the generated code for the host machine’s CPU, taking advantage of specific hardware features and instruction sets.path/to/main.rs
: As usual, this is the file path for the Rust source code being compiled.
Example Output:
The compiled binary may demonstrate improved performance benchmarks when executed on the target machine. It could benefit from CPU-specific features like AVX, SSE, or other instruction sets unique to the local processor architecture.
Use case 6: Display the target list
Code:
rustc --print target-list
Motivation:
Accessing the list of supported compilation targets is essential for cross-compilation tasks. It allows developers to plan and configure their builds for different architectures and operating systems, facilitating deployment on diverse platforms.
Explanation:
--print target-list
: Instructs the compiler to output a list of supported compilation targets, each represented by a unique target triple.
Example Output:
The output will include numerous target triples, such as x86_64-unknown-linux-gnu
or aarch64-apple-darwin
, indicating the various architectures and OS combinations Rust can compile for by default or with additional setup.
Use case 7: Compile for a specific target
Code:
rustc --target x86_64-unknown-linux-gnu path/to/main.rs
Motivation:
Cross-compiling for specific targets is necessary when the development environment and deployment environment differ in architecture or operating system. This ensures compatibility and optimal performance for the end user’s system.
Explanation:
--target x86_64-unknown-linux-gnu
: Specifies the target architecture and OS for the compiled binary. This example compiles for a 64-bit Linux system.path/to/main.rs
: Points to the Rust source file to compile against the specified target architecture and platform.
Example Output:
The compilation results in a binary that is compatible with the specified target environment, rather than the host’s default environment. This binary can now be transferred and executed on a machine matching the target architecture and operating system.
Conclusion
The rustc
command is a versatile and powerful tool for compiling Rust code under various scenarios. While cargo
often fulfills most project needs, rustc
provides fine-grained control over the compilation process, allowing for detailed optimizations, debugging configurations, cross-compilation, and more. By understanding these use cases, developers can harness the full potential of the Rust compiler for their specific needs.