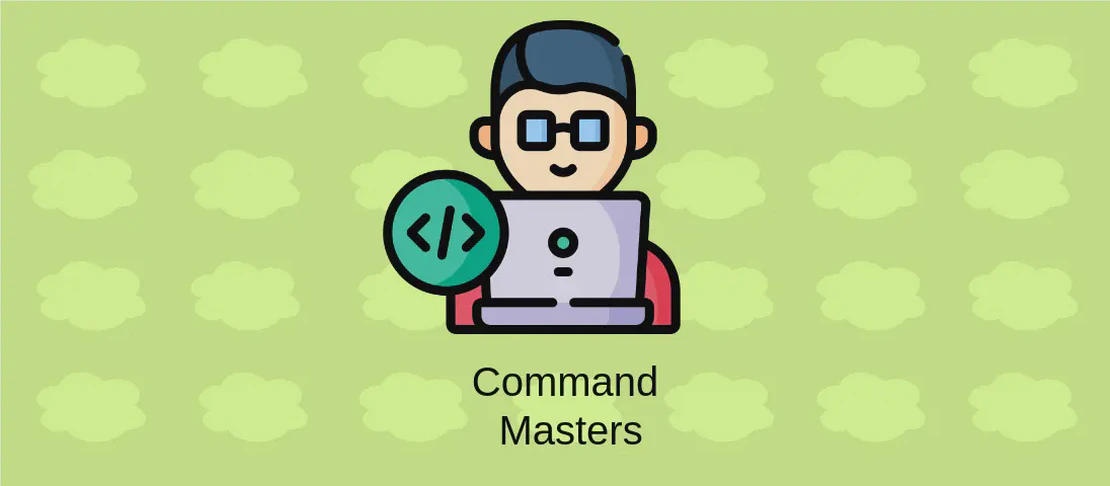
Generating Shell Completions for rustup and cargo (with examples)
Motivation:
When working with the Rust programming language, it is often helpful to have shell completions for the rustup
and cargo
commands. Shell completions provide suggestions and autocompletion for command line arguments, making it easier and faster to type commands accurately and efficiently. By generating shell completions for rustup
and cargo
, developers can enhance their productivity and reduce errors while interacting with the Rust toolchain.
1. Generating Bash Completion Script
Code:
rustup completions bash rustup cargo
Explanation:
The rustup completions
command generates the shell completion script for the specified shell (in this case, bash) and the provided commands (rustup
and cargo
). The completion script for bash will be printed to the standard output (stdout).
Example Output:
# Rustup Bash completion
function _rustup_completion() {
local commands global_opts opts
commands="toolchain override alias run which completions update show default install uninstall list update check search doc man self run completions help"
global_opts="--color always --quiet --no-self-update --offline --verbose"
opts="--help --verbose --quiet --color auto --color always --color never --force --no-pager --version"
COMPREPLY=()
local cur="${COMP_WORDS[COMP_CWORD]}"
local prev="${COMP_WORDS[COMP_CWORD-1]}"
local bin="rustup"
case "${prev}" in
"toolchain"|""|"completions")
COMPREPLY=( $(compgen -W "${commands}" -- ${cur}) )
return 0
;;
2. Generating Elvish Completion Script
Code:
rustup completions elvish rustup cargo
Explanation:
The rustup completions
command is used to generate shell completions for Elvish shell. By specifying the shell as elvish
and providing the commands (rustup
and cargo
), the completion script for Elvish shell will be printed to the standard output (stdout).
Example Output:
~mod@mac ~/rust/rustup (master=)$
editor $
prompt:
▶ eve completion/rustup
Fn {|-path -cmd|} {
put (comps lists: [~rustup ~cargo] $cmd)$paths
}
3. Generating Fish Completion Script
Code:
rustup completions fish rustup cargo
Explanation:
To generate shell completions for the fish shell, we use the rustup completions
command with the fish
argument followed by the commands rustup
and cargo
. The completion script for fish will be printed to the standard output (stdout).
Example Output:
# Rustup Fish completion
#
# To activate this completion, add the following to your
# `~/.config/fish/config.fish`:
#
# rustup completions fish | source
#
# NOTE: Alternatively, you can also generate completions for rustup and the cargo command by following the same steps as above.
function __fish_rustup_using_project_root
if test -z (command -v rustup)
return 1
end
set -q _RU_YOG_SOURCE
and test "$_RU_YOG_SOURCE" = "$PWD"
return $status
end
function _metric_fu_using
end
4. Generating PowerShell Completion Script
Code:
rustup completions powershell rustup cargo
Explanation:
By running the rustup completions
command with the powershell
argument followed by the commands rustup
and cargo
, we can generate shell completions for the PowerShell environment. The completion script for PowerShell will be printed to the standard output (stdout).
Example Output:
# Rustup PowerShell completion
param(
[Parameter(Position = 0, Mandatory = $True, ValueFromPipeline = $False)]
[ValidateSet("cargo", "rustup")]
[String]$CommandName,
[Parameter(Position = 1)]
[String]$BindingVariable,
[Parameter(Position = 2)]
[String]$StubSource
)
Set-StrictMode -Version Latest
if ($CommandName -eq "rustup") {
...
}
elseif ($CommandName -eq "cargo") {
...
}
5. Generating Zsh Completion Script
Code:
rustup completions zsh rustup cargo
Explanation:
To generate shell completions for the Zsh shell, we use the rustup completions
command with the zsh
argument followed by the commands rustup
and cargo
. The completion script for Zsh will be printed to the standard output (stdout).
Example Output:
#compdef rustup cargo
zstyle ':completion::complete:rustup::' use-cache 1
zstyle ':completion::complete:rustup::' cache-path $HOME/.cargo/tmp/.rustup-zcomps
zstyle ':completion::complete:rustup::' dispatcher breadth-first
zstyle ':completion::complete:rustup::' menu select
# Pre-command hook for validating Only-tags
__precommands_tags() {
local -a commands
if [[ ! -r "$XDG_CACHE_HOME/rustup/installed-tools" ]] {
commands=("completions")
}
if [[ ( -n "$commands" ) ]]; then
_wanted commands expl "${commands[@]}" compadd -a commands
fi
}
_rustup() {
local expl
_arguments -C "1: :->subcmds" \
"2: :->args" \
"*:: :->args_rest" \
local subcmds=(...long list of subcommands...)
if [[ ( $state = subcmds ) ]]; then
_wanted commands expl "Subcommand" compadd -a subcmds
fi
subcmds=(
'show: :_rustup_show'
...
)
if [[ ( -n "$subcmds[(r)$words[1]]" ) ]]; then
"$subcmds[(r)$words[1]]"
fi
}
By utilizing the rustup completions
command with different shell arguments, developers can generate shell completion scripts for rustup
and cargo
in their preferred shell environments. These completion scripts provide improved productivity and error-reduction while interacting with the Rust toolchain.