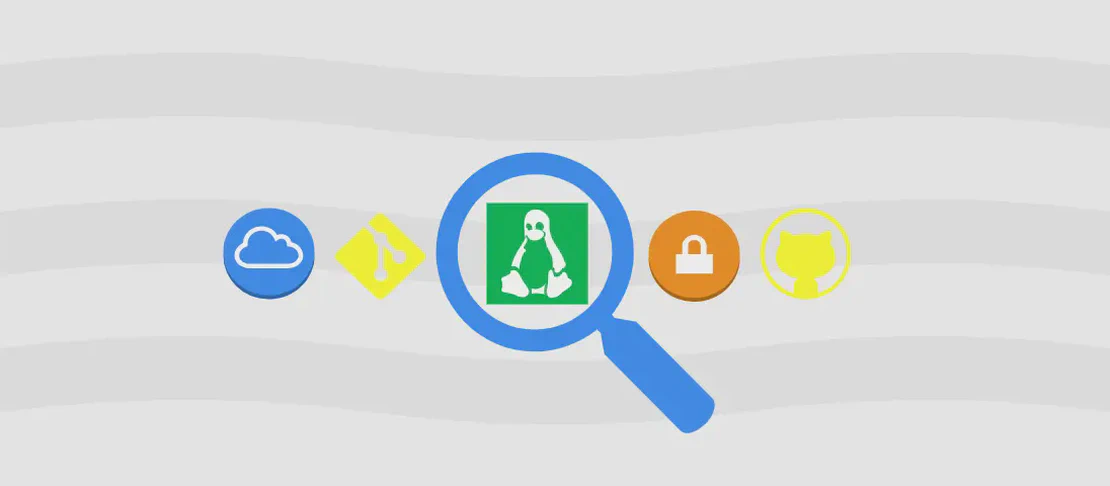
Mastering AWS SAM CLI Commands (with examples)
- Linux
- December 17, 2024
The AWS Serverless Application Model (SAM) CLI is a toolkit designed to simplify the process of building serverless applications on AWS. It provides a command-line interface that allows developers to quickly create, build, test, and deploy serverless applications using AWS resources like AWS Lambda, API Gateway, S3, and more. SAM CLI streamlines the serverless application lifecycle, acting as an efficient, reliable assistant for developers by automating several tasks and reducing the manual configuration work required by AWS.
Use case 1: Initialize a serverless application
Code:
sam init
Motivation:
Starting a serverless application from scratch can be a daunting task, but with the sam init
command, AWS provides a structured, boilerplate application framework, ensuring developers kick off their projects with best practices in mind. This step effectively lowers the entry barrier, enabling beginners to focus on business logic rather than configuration.
Explanation:
When you run sam init
, the SAM CLI prompts a user-friendly interactive session, guiding you through a series of questions to help scaffold your application with the appropriate configuration files and folder structure. It asks about the template you prefer (AWS Quick Start templates, AWS SAM Hello World example, etc.) and whether you’d like a minimal or a full-blown application setup.
Example Output:
After executing the command, you will observe a folder structure similar to:
.
├── events
├── hello-world
│ ├── app.js
│ ├── package.json
│ └── tests
└── template.yaml
This structure serves as a starting point for your serverless application, complete with a template.yaml
configuration file and sample Lambda function code.
Use case 2: Initialize a serverless application with a specific runtime
Code:
sam init --runtime python3.7
Motivation:
Different applications require different runtimes based on the programming language and specific requirements like libraries or frameworks. By specifying a runtime such as python3.7
, developers can ensure they’re working within their familiar environment from the start, aligning the project’s infrastructure and functions with their tech stack.
Explanation:
The --runtime
flag allows the developer to specify the programming language runtime for the Lambda function. In this case, python3.7
sets up the application to use Python 3.7, installing necessary dependencies and creating sample code accordingly.
Example Output:
A folder structure set for a Python environment is generated:
.
├── events
├── hello_world
│ ├── __init__.py
│ ├── requirements.txt
│ ├── app.py
│ └── tests
└── template.yaml
This ensures you’re ready to start coding in Python right away, with essential dependencies managed by requirements.txt
.
Use case 3: Package a SAM application
Code:
sam package
Motivation:
Deploying a serverless application often requires preparing the code and configuration in a format suitable for uploading to AWS. The sam package
command simplifies this process by packaging your application code and uploading it to an S3 bucket, substituting local file paths in the AWS CloudFormation template with S3 URLs.
Explanation:
sam package
builds and uploads the deployment package, transforming local paths into S3 URLs, thus readying the application for deployment in AWS. This step is vital because AWS CloudFormation needs to access code and templates as cloud-hosted resources.
Example Output:
Upon running this command, you receive confirmation like:
Uploading to xyz1234567/template 1234 / 1234.0 (100.00%)
Successfully packaged artifacts and wrote output template to file packaged-template.yaml.
This output indicates that your application artifacts are now hosted in S3 and the template is updated to reflect these changes.
Use case 4: Build your Lambda function code
Code:
sam build
Motivation:
Before testing or deploying the application, compiling and building the function code ensures all dependencies are correctly resolved and packaged. The sam build
command handles this, allowing developers to focus on functionality rather than deal with intricate dependency management.
Explanation:
sam build
processes and compiles the function code by creating a deployment package that includes your app’s code and dependencies. It uses the template.yaml
as a source and creates a .aws-sam
build folder which contains built artifacts in a form ready for deployment or local testing.
Example Output:
The output of this command shows the path where the build artifacts are stored:
Build Succeeded
Built Artifacts : .aws-sam/build
This indicates that the function code is packaged and ready for subsequent steps like local testing or deployment.
Use case 5: Run your serverless application locally
Code:
sam local start-api
Motivation:
Testing applications in the cloud can sometimes be inefficient and costly. By using sam local start-api
, developers get a local testing environment that mimics AWS Lambda and API Gateway, enabling quick iterations and debugging without incurring costs.
Explanation:
This command spins up a local HTTP server that simulates your AWS API Gateway setup. It listens to requests and invokes corresponding Lambda functions according to the template.yaml
file. This is imperative for testing Lambda functions and APIs accurately before deploying them to a live environment.
Example Output:
The output typically begins with a message like:
Mounting HelloWorldFunction at http://localhost:3000/ [GET]
This indicates a local endpoint where you can test your API through HTTP requests, enabling rapid development cycles.
Use case 6: Deploy an AWS SAM application
Code:
sam deploy
Motivation:
After building and testing an application, deploying it to the cloud is the final step. sam deploy
automates this, ensuring applications are smoothly transitioned from local environments to AWS infrastructure while managing resources and permissions efficiently.
Explanation:
sam deploy
uses AWS CloudFormation to deploy your serverless application. It involves creating or updating the necessary AWS resources, enabling deployment of your packaged code and template efficiently onto AWS Lambda, API Gateway, and other services.
Example Output:
Executing this command typically concludes with output like:
CloudFormation stack 'my-sam-app' was successfully deployed
This message confirms successful deployment, with your application now live on AWS infrastructure.
Conclusion:
AWS SAM CLI serves as a powerful tool in the developer’s toolkit for creating, managing, and deploying serverless applications on AWS. With commands tailored to every stage of the development lifecycle—from initialization and building, to local testing and final deployment—it simplifies complex tasks and facilitates efficient serverless application development. Whether you’re starting a new project or updating an existing one, mastering these SAM CLI commands will help optimize your serverless deployment workflow.