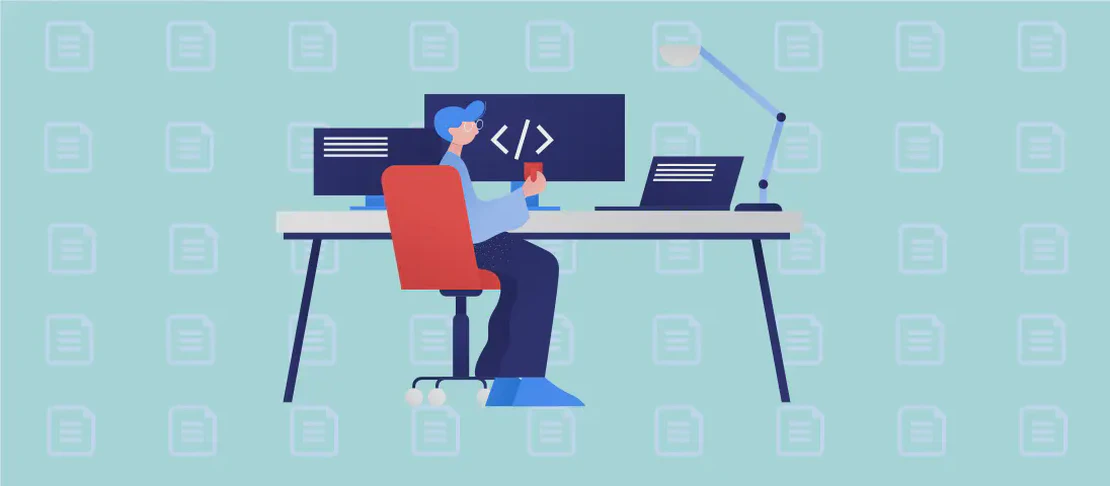
How to Use the Command 'sbt' (with Examples)
The Simple Build Tool (sbt) is a powerful build tool designed for Scala and Java projects. It helps developers manage project dependencies, compile source code, execute tests, and automate numerous other tasks associated with building software. sbt integrates deeply with the Scala programming language and possesses a broad range of functionalities that streamline project development, making it an indispensable asset in Scala-based software development. Below, we explore various use cases of sbt with illustrative examples for each.
Use Case 1: Start a REPL (Interactive Shell)
Code:
sbt
Motivation: Starting a REPL (Read-Eval-Print Loop) is essential for developers who wish to interactively test and explore snippets of code, experiment with Scala libraries, or run quick computations. A REPL provides a dynamic environment conducive to learning and debugging, enabling programmers to get immediate feedback on their code without the overhead of a full compile cycle.
Explanation:
sbt
: Launches the sbt interactive shell interface. Once started, commands can be run directly within this shell to perform various build operations.
Example Output:
[info] Loading project definition from /path/to/project
[info] Set current project to your-project-name
>
Use Case 2: Create a New Scala Project from an Existing Giter8 Template Hosted on GitHub
Code:
sbt new scala/hello-world.g8
Motivation: Creating a new project from a Giter8 template saves time and effort by providing a pre-configured project structure. These templates often include common settings, dependencies, and build configurations that form the best practices for starting new Scala applications. This functionality is particularly useful for beginners entering the Scala ecosystem.
Explanation:
sbt new
: Invokes sbt to create a new project using a specified Giter8 template.scala/hello-world.g8
: This argument specifies the Giter8 template to use, which in this case is a simple “hello-world” project template hosted on GitHub under the “scala” repository.
Example Output:
name [Hello World Template Project]:
Template applied in ./hello-world
Use Case 3: Compile and Run All Tests
Code:
sbt test
Motivation: Running tests is a crucial part of the software development process, enabling developers to ensure that code changes do not break existing functionality. Regularly compiling and executing tests helps maintain code quality and reliability, facilitating early detection and resolution of bugs.
Explanation:
sbt test
: Initiates sbt to compile source files in the test directories and then runs all unit and integration tests defined within the project.
Example Output:
[info] Compiling 3 Scala sources to /path/to/project/target/scala-2.13/test-classes...
[info] Test run finished: 3 passed, 0 failed
Use Case 4: Delete All Generated Files in the target
Directory
Code:
sbt clean
Motivation:
Over time, the target
directory can accumulate temporary files, compiled artifacts, and build outputs. Running sbt clean
removes these files, ensuring a clean state for subsequent builds. This can be beneficial when diagnosing build issues or preparing for a fresh deployment.
Explanation:
sbt clean
: Executes theclean
task, which deletes all generated files in thetarget
directory, including class files and other build outputs.
Example Output:
[success] Total time: 0 s, completed Oct 18, 2023 10:00:00 AM
Use Case 5: Compile the Main Sources in src/main/scala
and src/main/java
Directories
Code:
sbt compile
Motivation: Compiling source code is a fundamental step in the build process. It transforms human-readable code into machine-readable bytecode, which is executable on the Java Virtual Machine (JVM). This step is essential for verifying code correctness before further stages like testing or deploying.
Explanation:
sbt compile
: Directs sbt to compile all source files located in thesrc/main/scala
andsrc/main/java
directories, producing compiled.class
files in thetarget
directory.
Example Output:
[info] Compiling 10 Scala and Java sources to /path/to/project/target/scala-2.13/classes...
[success] Total time: 2 s, completed Oct 18, 2023 10:05:00 AM
Use Case 6: Use a Specified Version of sbt
Code:
sbt -sbt-version version
Motivation: Projects occasionally require specific sbt versions to ensure compatibility with existing plugins or configurations. Specifying the sbt version guarantees a consistent build environment, reducing discrepancies between local and production builds.
Explanation:
sbt -sbt-version version
: The-sbt-version
flag specifies the desired version of sbt for the project. Replaceversion
with the actual version number, such as1.5.5
.
Example Output:
[info] Using sbt version 1.5.5
[info] Loading project definition from /path/to/project
Use Case 7: Use a Specific Jar File as the sbt Launcher
Code:
sbt -sbt-jar path
Motivation: In some situations, custom or experimental sbt launcher versions may be desired to utilize new features or adopt fixes before they officially release. By using a specified jar file, developers can flexibly alter the sbt launch without modifying the global installation.
Explanation:
sbt -sbt-jar path
: The-sbt-jar
option allows the user to specify a particular jar file path that will act as the sbt launcher. Replacepath
with the actual path to the jar.
Example Output:
[info] Using custom sbt launcher from /custom/path/sbt-launch.jar
[info] Loading project definition from /path/to/project
Use Case 8: List All sbt Options
Code:
sbt -h
Motivation: Understanding the available options and configurations can significantly enhance a developer’s proficiency with sbt. Listing all options provides an overview of the possible commands and parameters that can be tailored to fit complex project needs, facilitating a more efficient build process.
Explanation:
sbt -h
: Utilizes the-h
flag, prompting sbt to display a help text summarizing all available options and their descriptions.
Example Output:
Usage: sbt [options]
-h, --help Show help and usage information
-sbt-version Specify the sbt version to use
-sbt-jar Specify the jar file to use as sbt launcher
...
Conclusion
sbt proves itself as an indispensable tool for Scala and Java developers with its multitude of functionalities tailored for effective project management and automation. The examples above illustrate both fundamental and advanced uses of sbt, each catering to distinct stages and requirements within the software development lifecycle. Whether starting a new project, running tests, or configuring the build environment, sbt equips developers with the necessary commands to streamline and optimize their workflow.