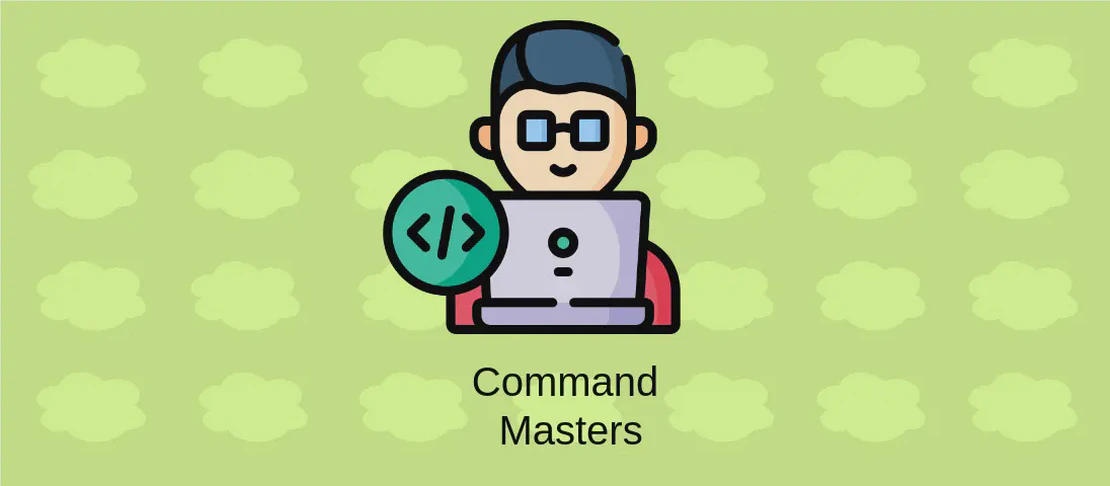
Harnessing Scalafmt for Efficient Scala Code Formatting (with examples)
Scalafmt is a powerful tool for developers working with the Scala programming language. This automatic code formatter ensures that Scala code is consistently formatted according to specified style guidelines. With configurations stored in the .scalafmt.conf
file, Scalafmt offers an advanced, customizable solution for improving code readability and maintainability across Scala projects. More information about this tool can be found at the official website: Scalafmt
.
Use case 1: Reformatting All .scala
Files Recursively
Code:
scalafmt
Motivation: In software development, keeping code style consistent across files enhances readability and reduces the potential for errors. By formatting all Scala files in the current directory recursively, Scalafmt ensures that all code adheres to the project’s style guide without requiring individual manual updates.
Explanation: When run without arguments, scalafmt
searches through the current directory and all its subdirectories for .scala
files, reformatting each one it finds. This approach is optimal for projects where the style guide is consistently applied across all source files, and manual formatting would be time-prohibitive.
Example Output:
Reformatting completed: 20 files updated and 5 files skipped.
Use case 2: Reformatting with a Custom Configuration
Code:
scalafmt --config path/to/.scalafmt.conf path/to/file_or_directory path/to/file_or_directory ...
Motivation: Projects often require specific style guidelines that differ from the default settings provided by formatting tools. By using a custom configuration file, developers can ensure that Scalafmt applies the project’s unique stylistic preferences to the specified files or directories.
Explanation: The --config
flag points to a custom .scalafmt.conf
file, which overrides the default configuration. This command requires input for both configuration and the paths to individual files or entire directories targeted for reformatting. Precision is enhanced through this approach, as only selected components of the project are formatted.
Example Output:
Configuration loaded from path/to/.scalafmt.conf
Formatted 3 directories and 6 individual files as per custom configuration.
Use case 3: Checking Formatting Compliance
Code:
scalafmt --config path/to/.scalafmt.conf --test
Motivation: Before integrating changes through code reviews or automated processes, it’s vital to confirm that all files comply with the established formatting rules. Scalafmt’s test mode assists in maintaining code standards by verifying that the files are correctly formatted, returning a status code of 0
if they comply.
Explanation: The --test
argument works in conjunction with --config
to check against the settings specified in the custom configuration file. This is particularly useful for Continuous Integration (CI) pipelines, where automated checks can prevent improperly formatted code from being merged into the main codebase.
Example Output:
All files are correctly formatted.
(Status code: 0)
Use case 4: Excluding Specific Files or Directories
Code:
scalafmt --exclude path/to/file_or_directory ...
Motivation: In some situations, certain files or directories should remain untouched by automatic formatting due to specific reasons like licensing, generated code, or vendor libraries. Scalafmt provides the ability to exclude these files from being reformatted.
Explanation: The --exclude
argument accepts one or more paths identifying files or directories the user wishes to omit from reformatting operations. It is essential in preserving intended formatting deviations that, if altered, might disrupt functionality or styling intricacies.
Example Output:
Excluding: path/to/vendor, path/to/generated_code
Reformatting completed: 10 files formatted, 3 exclusions applied.
Use case 5: Formatting Edited Files Against the Current Git Branch
Code:
scalafmt --config path/to/.scalafmt.conf --mode diff
Motivation: As development progresses, the focus often lies on modifications made since the last commit. Formatting only the altered files ensures that the differences align with the style guidelines without unnecessarily touching stable sections of the code, enhancing both performance and code quality.
Explanation: The --mode diff
argument checks the changes made against the current Git branch and formats only those altered lines or files. This refinement reduces processing time and conflicts that might arise from reformatting unchanged sections of the codebase. Like previous use cases, the --config
argument allows for the use of a custom configuration file to direct styling operations.
Example Output:
Detected changes in 2 files.
Formating applied only to modified sections.
Conclusion
Overall, Scalafmt provides robust capabilities for managing and enforcing code style guidelines in Scala projects. Through its various uses—from bulk formatting tasks to nuanced exclusion options—developers can leverage Scalafmt to streamline coding efforts and enhance consistency, thereby supporting both individual productivity and team-wide collaboration.