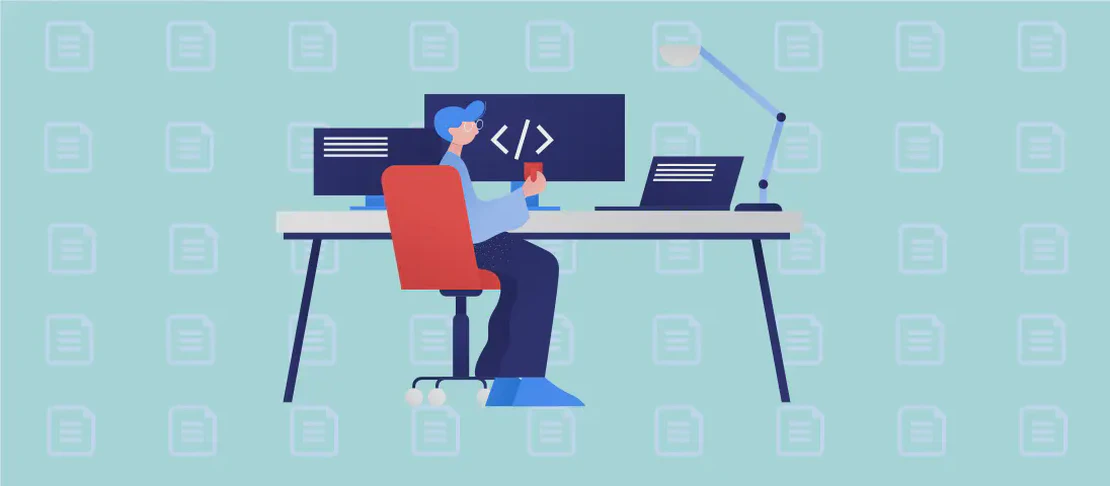
How to use the command 'scc' (with examples)
scc
is a powerful tool written in Go, designed primarily for counting lines of code in a directory or set of directories. Unlike simple line counters, scc
offers a nuanced breakdown of lines into logical, blank, and comment categories, displays code complexity, supports a myriad of programming languages, and allows for customization of output formats. This makes it an invaluable asset for developers and teams aiming to assess the scale, composition, and complexity of their codebases efficiently.
Print lines of code in the current directory
Code:
scc
Motivation:
By running scc
without any additional arguments, users quickly obtain a summary of the number of lines in code, including code, comments, and blank lines, within the current working directory. This offers an immediate snapshot of the codebase’s size in the current project, a crucial aspect for understanding project scale or comparing it across different time points or projects.
Explanation:
- The command
scc
examines all the files within the current directory and its subdirectories, tallying lines while distinguishing between code, comments, and blank lines. Its default behavior is to show a summarized scorecard, facilitating an understanding of the project metrics at a glance.
Example output:
──────────────────────────────────────────────────────────
Language Files Lines Blank Comment Code
──────────────────────────────────────────────────────────
Go 12 2904 300 145 2459
JavaScript 9 2220 180 200 1840
Markdown 2 500 100 0 400
──────────────────────────────────────────────────────────
Total 23 5624 580 345 4699
──────────────────────────────────────────────────────────
Print lines of code in the target directory
Code:
scc path/to/directory
Motivation:
When working with multiple projects, each situated in distinct directories, developers often need code metrics for a specific target directory rather than their present working directory. This command facilitates that by allowing the user to specify the path to any directory of interest, computing its code metrics efficiently.
Explanation:
- By appending
path/to/directory
, the user directsscc
to process this specific directory rather than the current directory. This provides flexibility in monitoring various parts of one’s filesystem, beneficial for managing larger codebases split across multiple folders.
Example output:
──────────────────────────────────────────────────────────
Language Files Lines Blank Comment Code
──────────────────────────────────────────────────────────
Python 5 4300 500 300 3500
YAML 2 150 30 10 110
Dockerfile 1 50 5 5 40
──────────────────────────────────────────────────────────
Total 8 4500 535 315 3650
──────────────────────────────────────────────────────────
Display output for every file
Code:
scc --by-file
Motivation:
In some scenarios, especially while trying to identify problem files—for example, files that may need refactoring due to their size or complexity—it’s advantageous to see metrics per file rather than just summarized data. This feature allows developers to drill down to the line-by-line statistics of each file individually.
Explanation:
- The
--by-file
argument instructsscc
to list each file separately rather than aggregating data by language. This detailed output helps in locating large or complex files within the codebase, enabling more informed decision-making regarding code maintenance.
Example output:
────────────────────────────────────────────────────────────
Language File Lines Blank Comment Code
────────────────────────────────────────────────────────────
Go main.go 300 30 5 265
Go utils.go 150 15 3 132
JavaScript app.js 500 50 60 390
JavaScript config.js 220 20 40 160
────────────────────────────────────────────────────────────
Display output using a specific output format
Code:
scc --format json
Motivation:
Different stakeholders may require data in different formats, especially when integrating scc
with other tools. To automate reporting or for ease of parsing, output formats such as json
or csv
are quite useful. This command specifies the desired format, enhancing interoperability with other data processing tools or reporting systems.
Explanation:
--format json
directsscc
to output its findings in JSON format, which is easily digestible by many programming languages and third-party tools. This is especially practical when integratingscc
analytics into dashboards or when performing automated processing in scripts.
Example output:
[
{
"Language": "Go",
"Files": 12,
"Lines": 2904,
"Blank": 300,
"Comment": 145,
"Code": 2459
},
{
"Language": "JavaScript",
"Files": 9,
"Lines": 2220,
"Blank": 180,
"Comment": 200,
"Code": 1840
}
]
Only count files with specific file extensions
Code:
scc --include-ext go,java,js
Motivation:
During specialized code audits or for analysis purposes, developers or teams may be interested only in particular programming languages, perhaps for a focused refactor or to assess productivity across different languages used in a project. This command enables filtering of files by their extensions, focusing on the relevant parts of the codebase.
Explanation:
- The
--include-ext go,java,js
argument filters the filesscc
analyzes to only those with the specified extensions. This allows users to hone in on particular segments of the codebase, ensuring analysis is targeted and relevant.
Example output:
────────────────────────────────────────────────────────────
Language Files Lines Blank Comment Code
────────────────────────────────────────────────────────────
Go 12 2904 300 145 2459
Java 8 1700 170 120 1410
JavaScript 7 1800 150 175 1475
────────────────────────────────────────────────────────────
Total 27 6404 620 440 5344
────────────────────────────────────────────────────────────
Exclude directories from being counted
Code:
scc --exclude-dir .git,.hg
Motivation:
Large projects often contain directory structures, such as version control directories, which introduce noise in code analysis. Counting these might inflate the perceived size of a codebase or distort the understanding of code layout and complexity. This command is essential for obtaining a more accurate representation of only the relevant code content.
Explanation:
- The
--exclude-dir .git,.hg
argument instructsscc
to ignore directories that are typically hidden system files or version control repositories. By excluding such directories, the user ensures that only the pertinent aspects of the codebase are included in the analysis.
Example output:
────────────────────────────────────────────────────────────
Language Files Lines Blank Comment Code
────────────────────────────────────────────────────────────
Go 12 2780 290 142 2348
Python 10 3200 400 320 2480
────────────────────────────────────────────────────────────
Total 22 5980 690 462 4828
────────────────────────────────────────────────────────────
Display output and sort by column
Code:
scc --sort code
Motivation:
When code metrics are obtained, sorting criteria can be crucial for data interpretation. For instance, sorting by code lines can highlight the largest sources of code directly. This command makes it easier for users to prioritize certain analyses or operations based on their immediate needs.
Explanation:
- The
--sort code
argument reorganizes thescc
output so that it orders entries in terms of code lines. It facilitates easy identification and comparison of the segments of the codebase contributing most significantly to its overall lines of code.
Example output:
────────────────────────────────────────────────────────────
Language Files Lines Blank Comment Code
────────────────────────────────────────────────────────────
Go 12 2904 300 145 2459
JavaScript 9 2220 180 200 1840
TypeScript 4 1150 100 85 965
────────────────────────────────────────────────────────────
Display help
Code:
scc -h
Motivation:
Tools like scc
come with a vast array of options and configurations. New users or those needing a quick refresher can greatly benefit from an accessible help prompt that succinctly documents all available options and basic usage patterns, essential for maximizing the tool’s capabilities.
Explanation:
- The
-h
flag opens the help documentation directly within the terminal. It encapsulates a summary of commands and options, and is an efficient starting point for learning how to utilizescc
thoroughly.
Example output:
Usage of scc:
-by-file
Display output for every file
-format string
Output format: tabular|wide|json|csv|cloc-yaml|html|html-table (default "tabular")
...
Conclusion:
The scc
command is a versatile tool that significantly empowers developers and development teams to analyze and understand their codebases at both macro and micro levels. Through its diverse functionalities, such as targeted path analysis, file extension filtering, output customization, and directory exclusion, scc
provides comprehensive insights tailored to specific project needs. Whether for high-level project overviews or digging into individual files, scc
offers the features needed to maintain and elevate code development practices.