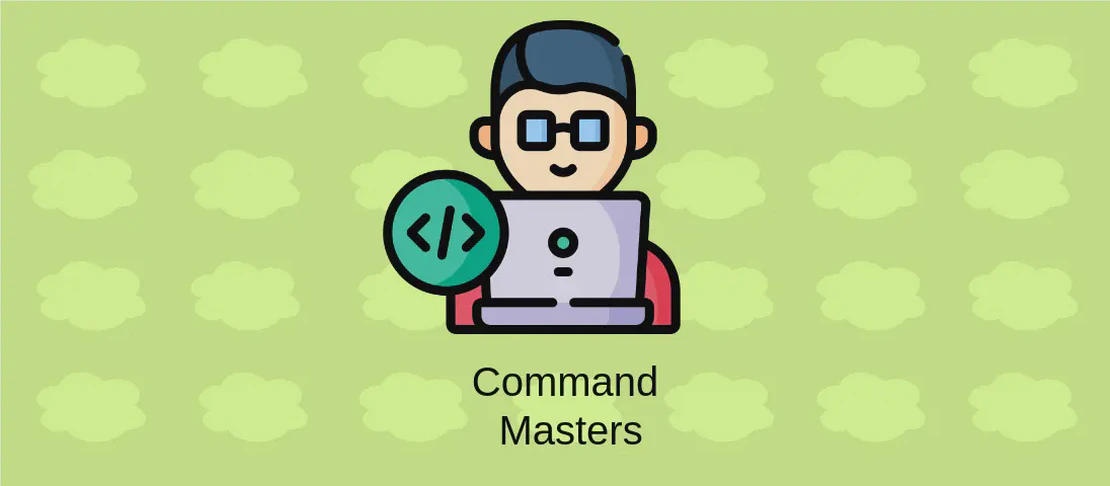
How to use the command 'scp' (with examples)
Secure copy.
Use case 1: Copy a local file to a remote host
Code:
scp path/to/local_file remote_host:path/to/remote_file
Motivation: This use case allows you to copy a file from your local machine to a remote host. It is useful when you want to transfer files between your local machine and a remote server.
Explanation:
path/to/local_file
: Path to the local file you want to copy.remote_host
: The remote host where you want to copy the file.path/to/remote_file
: Path where you want to save the file on the remote host.
Example output: The local file “example.txt” is copied to the remote host at the path “/home/user/example.txt”.
Use case 2: Use a specific port when connecting to the remote host
Code:
scp -P port path/to/local_file remote_host:path/to/remote_file
Motivation: This use case is useful when the remote host is using a non-standard SSH port. It allows you to specify the port number to connect to the remote host.
Explanation:
-P port
: Specifies the port number to use for the connection.path/to/local_file
: Path to the local file you want to copy.remote_host
: The remote host where you want to copy the file.path/to/remote_file
: Path where you want to save the file on the remote host.
Example output: The local file “example.txt” is copied to the remote host at the path “/home/user/example.txt” using port 2222.
Use case 3: Copy a file from a remote host to a local directory
Code:
scp remote_host:path/to/remote_file path/to/local_directory
Motivation: This use case allows you to retrieve a file from a remote host and save it to a local directory. It is helpful when you want to download files from a remote server to your local machine.
Explanation:
remote_host
: The remote host from where you want to copy the file.path/to/remote_file
: Path of the file on the remote host you want to copy.path/to/local_directory
: Local directory where you want to save the copied file.
Example output: The file “example.txt” from the remote host is copied to the local directory “/home/user/downloads”.
Use case 4: Recursively copy the contents of a directory from a remote host to a local directory
Code:
scp -r remote_host:path/to/remote_directory path/to/local_directory
Motivation: This use case is useful when you want to copy the entire contents of a directory from a remote host to a local directory, including subdirectories and files. It allows you to easily transfer entire directories between hosts.
Explanation:
-r
: Recursively copies the contents of the directory.remote_host
: The remote host from where you want to copy the directory.path/to/remote_directory
: Path of the directory on the remote host you want to copy.path/to/local_directory
: Local directory where you want to save the copied directory.
Example output: The contents of the remote directory “/home/user/data” are recursively copied to the local directory “/tmp/data”.
Use case 5: Copy a file between two remote hosts transferring through the local host
Code:
scp -3 host1:path/to/remote_file host2:path/to/remote_directory
Motivation: This use case is useful when you want to transfer a file between two remote hosts, but the hosts cannot directly communicate with each other. Transferring the file through the local host acts as an intermediary to facilitate the transfer.
Explanation:
-3
: Transfers the file through the local host.host1
: The source remote host from where you want to copy the file.path/to/remote_file
: Path of the file on the source remote host you want to copy.host2
: The destination remote host where you want to save the file.path/to/remote_directory
: Path of the directory on the destination remote host where you want to save the file.
Example output: The file “example.txt” is copied from “host1” to “host2” through the local host.
Use case 6: Use a specific username when connecting to the remote host
Code:
scp path/to/local_file remote_username@remote_host:path/to/remote_directory
Motivation: This use case is useful when you need to specify a specific username to connect to the remote host. It allows you to authenticate with a different username than the one you are currently logged in as.
Explanation:
remote_username
: The username to use when connecting to the remote host.remote_host
: The remote host where you want to copy the file.path/to/local_file
: Path to the local file you want to copy.path/to/remote_directory
: Path where you want to save the file on the remote host.
Example output: The local file “example.txt” is copied to the remote host at the path “/home/user/example.txt” using the username “user123”.
Use case 7: Use a specific ssh private key for authentication with the remote host
Code:
scp -i ~/.ssh/private_key path/to/local_file remote_host:path/to/remote_file
Motivation: This use case allows you to use a specific ssh private key for authentication when connecting to the remote host. It is useful when you have multiple ssh keys and need to specify which one to use.
Explanation:
-i ~/.ssh/private_key
: Specifies the path to the ssh private key file to use for authentication.path/to/local_file
: Path to the local file you want to copy.remote_host
: The remote host where you want to copy the file.path/to/remote_file
: Path where you want to save the file on the remote host.
Example output: The local file “example.txt” is copied to the remote host at the path “/home/user/example.txt” using a specific ssh private key.
Use case 8: Use a specific proxy when connecting to the remote host
Code:
scp -J proxy_username@proxy_host path/to/local_file remote_host:path/to/remote_file
Motivation: This use case allows you to specify a specific proxy to use when connecting to the remote host. It is useful when you need to connect to a remote host through a proxy server.
Explanation:
-J proxy_username@proxy_host
: Specifies the proxy to use when connecting to the remote host.path/to/local_file
: Path to the local file you want to copy.remote_host
: The remote host where you want to copy the file.path/to/remote_file
: Path where you want to save the file on the remote host.
Example output: The local file “example.txt” is copied to the remote host at the path “/home/user/example.txt” using the specified proxy.